Interacting Web UI and Solidity Smart Contract
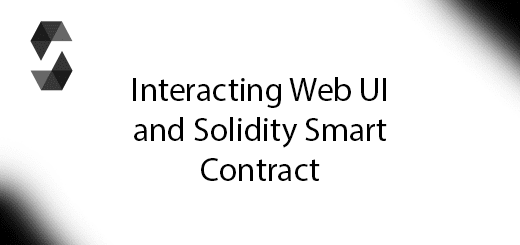
Install local blockchain
You need to install blockchain on your computer to run local testing. It is very expansive to run on Ethereum main-net, you need to spend real Ethereum to perform the transaction. Download Ganache to get free Ethereum to test on your local environment. Here is the link //truffleframework.com/ganache/.
Run Ganache
After install, open it on your computer, you will see the screen like below. Your localhost is HTTP://127.0.0.1:7545
Installing Web3.js
Web3.js is the official Ethereum Javascript API. Download this library to interact with smart contract on the Ethereum network. Before we install this library, we need to create a new folder call “webui_solidity”.
Open terminal and go to the folder.
cd webui_solidity
Next, run the npm init command to create a package.json file, which will store project dependencies:
npm init
Hit enter to all of the prompt messages. Next, run the following command to install web3.js:
npm install ethereum/web3.js --save
Create smart contract
Go to Remix Solidity IDE via //remix.ethereum.org/. In the “Run” tab, change the environment to Web3Provider and specific the blockchain node address. HTTP://127.0.0.1:7545
pragma solidity ^0.4.18; contract User { string fName; uint age; event CallbackUser (string name, uint age); function setUser(string _fName, uint _age) public { fName = _fName; age = _age; CallbackUser(_fName,_age); } function getUser() public constant returns (string, uint) { return (fName, age); } }
Press “Create” button and it will publish to the Ethereum network.
Create Web UI
Open the folder using your editor ( I am using Atom). Create a new html file called “index.html” and copy the following code.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <meta http-equiv="X-UA-Compatible" content="ie=edge"> <title>Document</title> <link rel="stylesheet" type="text/css" href="main.css"> <script src="./node_modules/web3/dist/web3.min.js"></script> </head> <body> <div class="container"> <h1>User</h1> <h2 id="user"></h2> <img id="loader" src="//loading.io/spinners/double-ring/lg.double-ring-spinner.gif"> <label for="name" class="col-lg-2 control-label">Name</label> <input id="name" type="text"> <label for="name" class="col-lg-2 control-label">Age</label> <input id="age" type="text"> <button id="button">Update</button> </div> <script src="//code.jquery.com/jquery-3.2.1.slim.min.js"></script> <script> if (typeof web3 !== 'undefined') { web3 = new Web3(web3.currentProvider); } else { // set the provider you want from Web3.providers web3 = new Web3(new Web3.providers.HttpProvider("//localhost:7545")); } web3.eth.defaultAccount = web3.eth.accounts[0]; var UserContract = web3.eth.contract(?); var User = UserContract.at('?'); var userEvent = User.CallbackUser(); userEvent.watch(function(error, result){ if(!error){ $("#loader").hide(); $("#user").html(result.args.name +"/"+result.args.age); }else{ $("#loader").hide(); } }); $("#button").click(function() { User.setUser($("#name").val(), $("#age").val()); $("#loader").show(); }); </script> </body> </html>
Edit to your ABI and Contract Address
var UserContract = web3.eth.contract(?);
replace ? to your ABI eg “[ { “constant”: true, “inputs”: [], “name”: “getUser”, “outputs”: [ { “name”: “”, “type”: “string” }, { “name”: “”, “type”: “uint256” } ], “payable”: false, “stateMutability”: “view”, “type”: “function” }, { “anonymous”: false, “inputs”: [ { “indexed”: false, “name”: “name”, “type”: “string” }, { “indexed”: false, “name”: “age”, “type”: “uint256” } ], “name”: “CallbackUser”, “type”: “event” }, { “constant”: false, “inputs”: [ { “name”: “_fName”, “type”: “string” }, { “name”: “_age”, “type”: “uint256” } ], “name”: “setUser”, “outputs”: [], “payable”: false, “stateMutability”: “nonpayable”, “type”: “function” } ]”.
Compile > Detail > Copy ABI.
var User = UserContract.at('?');
replace ? to your contract address eg “0xdda6327139485221633a1fcd65f4ac932e60a2e1”. In the run tab, bottom have a User contract adress and copy it.
Create a css file called “main.css”. Copy and paste the source code below.
body { background-color:#F0F0F0; padding: 2em; font-family: 'Raleway','Source Sans Pro', 'Arial'; } .container { width: 50%; margin: 0 auto; } label { display:block; margin-bottom:10px; } input { padding:10px; width: 50%; margin-bottom: 1em; } button { margin: 2em 0; padding: 1em 4em; display:block; } #instructor { padding:1em; background-color:#fff; margin: 1em 0; } #loader { width: 100px; display: none; }
Run Your Project
Now, you can run the html and try it !!!
This was for remix ide, what about running nodes in Ethereum blockchain? would the steps will be same for that as well??