Android PopUp Floating Window Tutorial
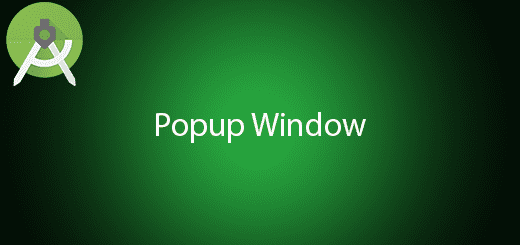
Android has a PopupWindow object to implements floating windows in the activity to display information or perform some action. It is very convenient when its come to some situation like give the user an extra window to interact with your app. You can customize your popup window in which you wanna display on that window. In this tutorial, I will let you understand how the android popup floating window work in the android application.
PopUp Floating Window Sample Screen
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “FloatingWindowExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add a new custom popupwindow xml
In the res>layout and right click create a new layout name “popup”, after that follow the source code in the below section.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="wrap_content" android:background="@color/colorAccent" android:layout_height="wrap_content"> <TextView android:text="Floating Windows" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/textView" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <Button android:text="Close" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/btnClose" android:layout_below="@+id/textView" android:layout_centerHorizontal="true" /> </RelativeLayout>
Edit activity_main.xml
Go to the activity_main.xml and add one button to display the floating button on your screen.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="//schemas.android.com/apk/res/android" xmlns:tools="//schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.questdot.floatingwindowexample.MainActivity"> <Button android:text="Click" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/btnFloating" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
Edit MainActivity.java class
After that, go to the mainactivity class and add the button listener to add the window in the screen and also set touch listener to drag the windows on the screen.
public class MainActivity extends AppCompatActivity { Button btnFloating; RelativeLayout relativeLayout; PopupWindow popupWindow; View popupView; int mCurrentX,mCurrentY; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnFloating = (Button)findViewById(R.id.btnFloating); relativeLayout = (RelativeLayout) findViewById(R.id.activity_main); btnFloating.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { LayoutInflater layoutInflater = (LayoutInflater)getBaseContext().getSystemService(LAYOUT_INFLATER_SERVICE); popupView = layoutInflater.inflate(R.layout.popup,null); popupWindow = new PopupWindow(popupView, RelativeLayout.LayoutParams.WRAP_CONTENT,RelativeLayout.LayoutParams.WRAP_CONTENT); Button btnClose = (Button)popupView.findViewById(R.id.btnClose); btnClose.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { popupWindow.dismiss(); } }); mCurrentX = 20; mCurrentY = 50; popupWindow.showAtLocation(relativeLayout, Gravity.NO_GRAVITY, mCurrentX, mCurrentY); popupView.setOnTouchListener(new View.OnTouchListener() { private float mDx; private float mDy; @Override public boolean onTouch(View v, MotionEvent event) { int action = event.getAction(); if (action == MotionEvent.ACTION_DOWN) { mDx = mCurrentX - event.getRawX(); mDy = mCurrentY - event.getRawY(); } else if (action == MotionEvent.ACTION_MOVE) { mCurrentX = (int) (event.getRawX() + mDx); mCurrentY = (int) (event.getRawY() + mDy); popupWindow.update(mCurrentX, mCurrentY, -1, -1); } return true; } }); } }); } }
Run Your Project
In conclusion, you are successfully implement a popupwindow in your android project. Now you can test it out in your device.
Hi,
I tried to implement this popup as you did exactly.
my app falls at this line:
——> popupView = layoutInflater.inflate(R.layout.popup,null);
Do you know why? or how can i figure it out?
TKS, that works very fine. Simple and complete.
I just made a little change.
I moved this hole part out of the click event:
LayoutInflater layoutInflater =(LayoutInflater)getBaseContext().getSystemService(LAYOUT_INFLATER_SERVICE);
popupView = layoutInflater.inflate(R.layout.popup,null);
popupWindow = new PopupWindow(popupView, RelativeLayout.LayoutParams.WRAP_CONTENT,RelativeLayout.LayoutParams.WRAP_CONTENT);
Inside the click event, it was intancing a new popupWindow on each click, even if there was some one up. And the close button was clossing just the last instance.
VideoView Is not working in this PopUp window..??
Nothing shows ,no any popUps.