Android Custom Spinner Tutorial
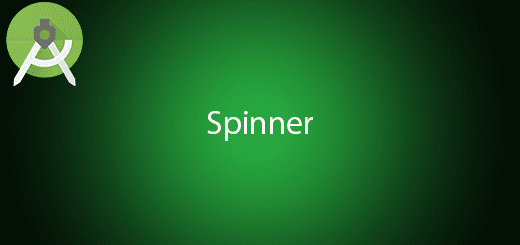
Spinner is a drop-down menu that displays multiple items to be able user to select an item from the menu. After user select item, the spinner will show the selected item on the device screen. It is much useful when you have multiple items and only allow the user to select one item on it. There have many ways to populate the list in the spinner. In this tutorial, I will guide you how to make android custom spinner in your android project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “SpinnerExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Create a new Custom Spinner Layout
Right-click your layout folder from the res package, and right click creates a new XML “custom_spinner_items”. After that edit this file to the following source code.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="//schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:id="@+id/tvNumber" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_weight="1" android:gravity="center" android:text="number" /> <TextView android:id="@+id/tvContent" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_gravity="center" android:layout_weight="1" android:text="content" /> </LinearLayout>
Create a new Adapter spinner
Right-click your package name and create a new class for your spinner adapter name”CustomSpinnerAdapter”. Follow the sample source code below.
package com.questdot.spinnerexample; import android.content.Context; import android.graphics.Color; import android.view.Gravity; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; import android.widget.BaseAdapter; import android.widget.ImageView; import android.widget.TextView; public class CustomSpinnerAdapter extends BaseAdapter { Context context; int num[]; String[] content; LayoutInflater inflter; public CustomSpinnerAdapter(Context applicationContext, int[] num, String[] content) { this.context = applicationContext; this.num = num; this.content = content; inflter = (LayoutInflater.from(applicationContext)); } @Override public int getCount() { return num.length; } @Override public Object getItem(int i) { return null; } @Override public long getItemId(int i) { return 0; } @Override public View getView(int i, View view, ViewGroup viewGroup) { view = inflter.inflate(R.layout.custom_spinner_items, null); TextView number = (TextView) view.findViewById(R.id.tvNumber); TextView names = (TextView) view.findViewById(R.id.tvContent); number.setText(""+num[i]); names.setText(content[i]); return view; } }
Edit activity_main.xml layout
Go to this layout and add spinner in the relative layout.
<RelativeLayout xmlns:android="//schemas.android.com/apk/res/android" xmlns:tools="//schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <Spinner android:id="@+id/simpleSpinner" android:background="@drawable/custom_spinner_background" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> </RelativeLayout>
Edit MainActivity.java class
Go to MainActivity class, this class will populate the content to the customSpinnerAdapter and display to the screen.
public class MainActivity extends AppCompatActivity implements AdapterView.OnItemSelectedListener{ String[] content ={"Android","IOS","Windows","Mac OS","Ubuntu","Windows Phone"}; int number[] = {1,2,3,4,5,6}; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Spinner spin = (Spinner) findViewById(R.id.simpleSpinner); spin.setOnItemSelectedListener(this); CustomSpinnerAdapter customAdapter=new CustomSpinnerAdapter(getApplicationContext(),number, content); spin.setAdapter(customAdapter); } @Override public void onItemSelected(AdapterView<?> arg0, View arg1, int position, long id) { Toast.makeText(getApplicationContext(), content[position], Toast.LENGTH_LONG).show(); } @Override public void onNothingSelected(AdapterView<?> arg0) { // TODO Auto-generated method stub } }
Add new drawable file
Create new XML file”custom_spinner_background” in your drawable, this file is to customize your spinner background.
<?xml version="1.0" encoding="UTF-8"?> <shape xmlns:android="//schemas.android.com/apk/res/android" android:shape="rectangle" > <solid android:color="@android:color/white"/> <corners android:radius="4dp"></corners> <stroke android:color="#cccccc" android:width="1dp"/> </shape>
Run Your Project
In conclusion, now you can run this project in your device.