Android Image Gallery HorizontalScrollView Tutorial
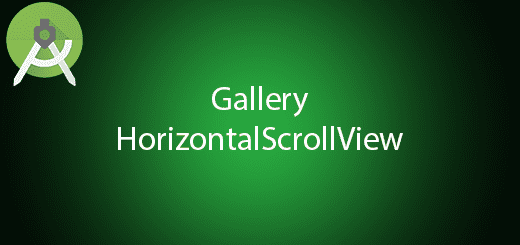
Gallery widely used in displaying a list of thumbnails image but Gallery class was depreciated in API 16 which is Android version 4.1. So, we will need to use another widget to fully replace gallery widget. The best solution to solve this problem is using horizontalScrollView, it very simple to implement in the activity. HorizontalScrollView is a frame layout and scroll item horizontally which different with ScrollView in vertical scrolling. You should place one child inside the horizontal scroll view such as linear layout. In this tutorial, I will give you some steps to use the horizontal scroll view in the Android activity.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “HorizontalSVExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit activity_main.xml layout
Go to activity_main.xml edit your XML to below source code. I add horizontal scroll view and linear layout in this XML.
<LinearLayout xmlns:android="//schemas.android.com/apk/res/android" xmlns:tools="//schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" > <HorizontalScrollView android:layout_width="wrap_content" android:layout_height="150dp" android:layout_gravity="center_vertical" android:scrollbars="none" > <LinearLayout android:id="@+id/id_gallery" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:orientation="horizontal" > </LinearLayout> </HorizontalScrollView> </LinearLayout>
Add a new xml
Create a new xml file by go to res > right click layout > new > layout resource file. Name it to activity_gallery_item.xml then click ok buton.
Edit activity_gallery_item.xml layout
This customizes the item layout in the horizontal scrollview. So u can inflate the xml later.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="//schemas.android.com/apk/res/android" android:layout_width="120dp" android:layout_height="120dp" android:background="@android:color/white" > <ImageView android:id="@+id/id_index_gallery_item_image" android:layout_width="80dp" android:layout_height="80dp" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:layout_margin="5dp" android:scaleType="centerCrop" /> <TextView android:id="@+id/id_index_gallery_item_text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@id/id_index_gallery_item_image" android:layout_centerHorizontal="true" android:layout_marginBottom="5dp" android:layout_marginTop="5dp" android:textColor="#ff0000" android:textSize="12dp" /> </RelativeLayout>
Add an image in drawable
Add one image to your drawable package, just copy and paste one image file to your drawable package.
Edit MainActivity.java class
Follow below source code and write to mainactivity.java. This class is to add the view to the horizontalscrollview.
package com.example.questdot.horizontalsvexample; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.LayoutInflater; import android.view.View; import android.widget.HorizontalScrollView; import android.widget.ImageView; import android.widget.LinearLayout; import android.widget.TextView; public class MainActivity extends AppCompatActivity { private LinearLayout mGallery; private int[] mImgIds; private LayoutInflater mInflater; private HorizontalScrollView horizontalScrollView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mInflater = LayoutInflater.from(this); initData(); initView(); } private void initData() { mImgIds = new int[] { R.drawable.screenshot, R.drawable.screenshot, R.drawable.screenshot,R.drawable.screenshot }; } private void initView() { mGallery = (LinearLayout) findViewById(R.id.id_gallery); for (int i = 0; i < mImgIds.length; i++) { View view = mInflater.inflate(R.layout.activity_gallery_item, mGallery, false); ImageView img = (ImageView) view .findViewById(R.id.id_index_gallery_item_image); img.setImageResource(mImgIds[i]); TextView txt = (TextView) view .findViewById(R.id.id_index_gallery_item_text); txt.setText("info "+i); mGallery.addView(view); } } }
Run your project
Now, you can run your project in your emulator or android device.
(Android Image Gallery HorizontalScrollView)
Source Code
i want from gallery images not from drawble .can any one help me pls. thanks in advance.
This tutorial helped me a lot.clear explanation, thank you.
Thanks – I converted this quick snippet to Kotlin for a project I am working on.
Awesome. 🙂