Android Service Tutorial
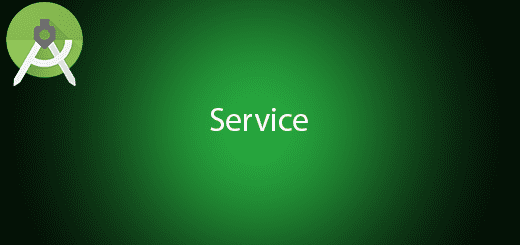
Android Service is a component that running in the background without a user interface. It is a long running operation and does not need to interact with the user. Besides, android service is mostly use for specific situation such as download files in the internet and listening music. There have 2 type of services which are started and bound. Android Start Service is started when calling startservice() method by an application component (eg. Activity). Once the service started, it will run in the background forever and cannot be destroy without calling stopservice method. Android Bind Service is bound when an application component (eg activity) binds to it by calling bindService() method. It offers a client-server interface and allows components to interact with the service. For example, service process calculation and updating the activity ui. The bound service able to return a value or result to the user which started service cannot perform.
Both have different behaviour and life cycle. You can use either startService() or bindService() to start the android service. If you create a service by binding to it, it will die when you unbind the service. To keep the service working around without component activities binding to it is to start it with startService(). The started service is no conflict to the application lifecycle because it does not bind to any activity. Therefore, you are able to bind and unbind to it as much as you wish and it will only die when you call stopService(). In this tutorial, I will show you how the activity interacts with the android service and transmit data in android service.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “ServiceExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Project” and Click Next button
5. Lastly, press finish button.
Create a new Service
Add the service class in your package. Right click your package name click New > Service > Service. It will pop up a windows and click finish button. (Optional: you can change service class name).
Once service class created, you will see <service> is include in your AndroidManist.xml.
<service android:name=".MyService" android:enabled="true" android:exported="true"></service>
Edit MyService.java class
Go to your MyService.java add the following code. I add the callback interface to display the internal message to the activity. In the thread need to use handler to update UI in the activity.
IBinder onBind(Intent intent)
– It will called when the activity bind to the service and return the communication channel to the service.
For below example I return a new Binder for this method. I overwrite new Binder which using the class Binder that extends android.os.Binder.
int onStartCommand(Intent intent, int flags, int startId)
– It will called by everytime explicitly start the service by calling startService().
package com.example.questdot.serviceexample; import android.app.Service; import android.content.Intent; import android.os.IBinder; import android.telecom.Call; import android.widget.Toast; public class MyService extends Service { private boolean running=false; private String data ="default data"; public MyService() { } @Override public IBinder onBind(Intent intent) { // TODO: Return the communication channel to the service. return new Binder(); } public class Binder extends android.os.Binder{ public void setData(String data){ MyService.this.data= data; } public MyService getMyService(){ return MyService.this; } } @Override public int onStartCommand(Intent intent, int flags, int startId) { data = intent.getStringExtra("data"); return super.onStartCommand(intent, flags, startId); } @Override public void onCreate() { super.onCreate(); running=true; new Thread(){ @Override public void run() { super.run(); int i = 0; while (running) { i++; String string = i+" : "+data; System.out.println(string); if(callback!=null){ callback.onDateChange(string); } try { sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } }.start(); } @Override public void onDestroy() { super.onDestroy(); running=false; } public void setCallback(Callback callback) { this.callback = callback; } public Callback getCallback() { return callback; } private Callback callback= null; public static interface Callback{ void onDateChange(String data); } }
Edit activity_main.xml layout
Add the textView, editText and 5 buttons in your activity_main.xml. The 5 button perform the feature such as start,stop,bind,unbind and synchronise service.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.questdot.serviceexample.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="-" android:id="@+id/textView" android:layout_gravity="center_horizontal" /> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/editText" android:text="default data" android:layout_gravity="center_horizontal" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Start Service" android:id="@+id/btnStart" android:layout_gravity="center_horizontal" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Stop Service" android:id="@+id/btnStop" android:layout_gravity="center_horizontal" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Bind Service" android:id="@+id/btnBind" android:layout_gravity="center_horizontal" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Unbind Service" android:id="@+id/btnUnbind" android:layout_gravity="center_horizontal" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Syn Data" android:id="@+id/btnSyn" android:layout_gravity="center_horizontal" /> </LinearLayout>
Edit MainActivity.java class
In your MainActivity.java insert the following code. You should implements View.OnClickListener and ServiceConnection in your activity. If you want to use the bind service you need to implement ServiceConnection interface and override two method.
void onServiceConnected(ComponentName name, IBinder service)
– This method will called when the bind service is connected.
void onServiceDisconnected(ComponentName name)
– This method will called when the connection of bind service is lost, killed or crashed.
package com.example.questdot.serviceexample; import android.content.ComponentName; import android.content.Context; import android.content.Intent; import android.content.ServiceConnection; import android.os.Handler; import android.os.IBinder; import android.os.Message; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.EditText; import android.widget.TextView; public class MainActivity extends AppCompatActivity implements View.OnClickListener, ServiceConnection { EditText editText; TextView textView; Intent i; private MyService.Binder binder=null; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); i= new Intent(this,MyService.class); editText=(EditText)findViewById(R.id.editText); textView= (TextView)findViewById(R.id.textView); findViewById(R.id.btnStart).setOnClickListener(this); findViewById(R.id.btnStop).setOnClickListener(this); findViewById(R.id.btnBind).setOnClickListener(this); findViewById(R.id.btnUnbind).setOnClickListener(this); findViewById(R.id.btnSyn).setOnClickListener(this); } @Override public void onClick(View v) { switch(v.getId()){ case R.id.btnStart: i.putExtra("data",editText.getText().toString()); startService(i); break; case R.id.btnStop: stopService(i); break; case R.id.btnBind: bindService(new Intent(this,MyService.class),this, Context.BIND_AUTO_CREATE); break; case R.id.btnUnbind: unbindService(this); break; case R.id.btnSyn: if(binder!=null){ binder.setData(editText.getText().toString()); } break; } } @Override public void onServiceConnected(ComponentName name, IBinder service) { binder = (MyService.Binder) service; binder.getMyService().setCallback(new MyService.Callback() { @Override public void onDateChange(String data) { Message msg = new Message(); Bundle b = new Bundle(); b.putString("data",data); msg.setData(b); handler.sendMessage(msg); } }); } @Override public void onServiceDisconnected(ComponentName name) { } private Handler handler = new Handler(){ @Override public void handleMessage(Message msg) { super.handleMessage(msg); textView.setText(msg.getData().getString("data")); } }; }
Run your project
Now, you can start your project and try the android service now.