Android Fragment Tutorial
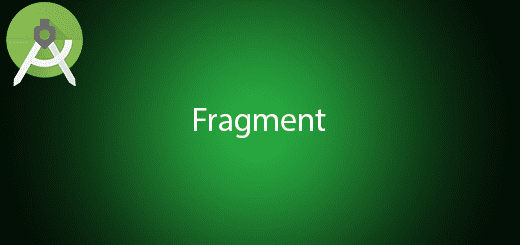
Android Fragment is a modular section of an activity which enable to combine multiple fragment into one activity. Each fragment activity have different layout, behavior and life cycle. Fragment should always embedded in the activity and it will affect by the host activity life cycle. For example, when the activity is destroyed then all the fragments will behave same as the activity.
In this tutorial, I will discuss on how to implement the android fragment in the android project. I will briefly explain how its work in the activity.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “FragmentExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Project” and Click Next button
5. Lastly, press finish button.
Create a new layout file
Go to res > layout, right click and create a new layout resource file for fragment_one.
Edit fragment_one.xml layout
Open your fragment_one.xml and edit the design to the following code. I put the textview for displaying which fragment is opening and the button is to switch to fragment two.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Fragment 1" android:textSize="36dp" android:id="@+id/textView" android:layout_gravity="center_horizontal" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="SWITCH FRAGMENT" android:id="@+id/button" android:layout_gravity="center_horizontal" /> </LinearLayout>
Create a new java class
Go to java > your package name right click and create new java class for fragment one. For example, I name the class as PlaceHolderFragment.
Edit PlaceHolderFragment.java class
This class should extends Fragment so it will categories as a Fragment. The inflator is an Object that can inflate any views in the fragment.
package com.example.questdot.fragmentexample; import android.os.Bundle; import android.support.annotation.Nullable; import android.support.v4.app.Fragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; /** * Created by HP on 19/5/2016. */ public class PlaceHolderFragment extends Fragment { public PlaceHolderFragment(){ } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View rootView = inflater.inflate(R.layout.fragment_one,container,false); rootView.findViewById(R.id.button).setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v) { getFragmentManager().beginTransaction() .addToBackStack(null) .replace(R.id.container, new PlaceHolder2Fragment()) .commit(); } }); return rootView; } }
Repeat Step 2 to create fragment two
Repeat the step 2. Go to res > layout, right click and create a new layout resource file for fragment_two.
Edit fragment_two.xml layout
Open your fragment_two.xml and edit the design to the following code. The button is to go back to the first fragment.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Fragment 2" android:textSize="72dp" android:id="@+id/textView2" android:layout_gravity="center_horizontal" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/button2" android:layout_gravity="center_horizontal" android:text="SWITCH FRAGMENT" /> </LinearLayout>
Create a new java class
Go to java > your package name right click and create new java class for fragment two. For example, I name the class as PlaceHolder2Fragment.
Edit PlaceHolder2Fragment.java class
package com.example.questdot.fragmentexample; import android.support.v4.app.Fragment; import android.os.Bundle; import android.support.annotation.Nullable; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; /** * Created by HP on 19/5/2016. */ public class PlaceHolder2Fragment extends Fragment { public PlaceHolder2Fragment(){ } @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View rootView = inflater.inflate(R.layout.fragment_two,container,false); rootView.findViewById(R.id.button2).setOnClickListener(new View.OnClickListener(){ @Override public void onClick(View v) { getFragmentManager().popBackStack(); } }); return rootView; } }
Edit activity_main.xml
In your activity_main.xml edit the relativelayout to framelayout.
<?xml version="1.0" encoding="utf-8"?> <FrameLayout android:layout_width="match_parent" android:layout_height="match_parent" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/container" tools:ignore="MergeRootFrame" tools:context=".MainActivity" xmlns:android="http://schemas.android.com/apk/res/android"></FrameLayout>
Edit MainActivity.java class
For your MainActivity class and insert the following code.
getSupportFragmentManager()
– Return the fragmentmanager to interacting with fragments assoicated with this activity.
beginTransaction()
– Start the edit operations on the fragments in the fragmentmanager.
package com.example.questdot.fragmentexample; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); if(savedInstanceState == null) { getSupportFragmentManager().beginTransaction() .add(R.id.container, new PlaceHolderFragment()) .commit(); } } }
Run your project
Now, you can start your android project and try the fragment in your own device.