Android combine Retrofit + RxJava Tutorial
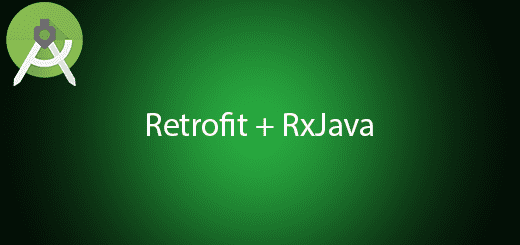
RxJava is the good java library for android development which composing asynchronous and event-based programs. It is a better than AsynTask because it has more control of the thread and more features to use. For retrofit, its supports the asynchronous and synchronize thread but its does not able to get much control of the thread. So we use use rxJava to replace with the retrofit asynchronous method. In this tutorial, I will tell you about android combine retrofit + rxjava in the project so you can be more understand about it.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “RetrofitRxJavaExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add rxJava, retrofit and gson dependencies
Go to build.gradle (Module:app) and add rxjava,retrofit and gson in the dependencies section. After that click sync so that the library will be added to your project.
compile 'com.google.code.gson:gson:2.6.2' compile 'com.squareup.retrofit2:retrofit:2.1.0' compile 'io.reactivex:rxandroid:1.2.1' compile 'io.reactivex:rxjava:1.1.6' compile 'com.squareup.retrofit2:converter-gson:2.1.0' compile 'com.squareup.retrofit2:adapter-rxjava:2.1.0'
Add a Model
Right click your project and create a new model class “ProfileResponses”. Copy paste the code into your model.
public class ProfileResponse { private String name; private String status; private String talent; public String getName() { return name; } public void setName(String name) { this.name = name; } public String getStatus() { return status; } public void setStatus(String status) { this.status = status; } public String getTalent() { return talent; } public void setTalent(String talent) { this.talent = talent; } }
Create an Interface
After that, create an interface “ProfileServices” by right click your project name”.
public interface ProfileServices { @POST("v2/584900841100004312590b79") Observable<Response<ProfileResponse>> postRawJson(); }
Create a new class
Add one more new class “RetrofitManager” for init the retrofit rest services so you can use and call it in your mainactivity.
public class RetrofitManager { private ProfileServices profileServices; public ProfileServices getDoorService() { if (profileServices == null) { Retrofit retrofit = new Retrofit.Builder() .baseUrl("http://www.mocky.io/") .addConverterFactory(GsonConverterFactory.create()) .addCallAdapterFactory(RxJavaCallAdapterFactory.create()) .build(); profileServices = retrofit.create(ProfileServices.class); } return profileServices; } }
Edit activity.main.xml layout
Go and edit the layout of your main activity.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.questdot.retrofitrxjavaexample.MainActivity"> <TextView android:text="Retrofit + RxJava" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_centerHorizontal="true" android:layout_marginBottom="92dp" /> <TextView android:text="Talent" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/txtTalent" android:layout_centerVertical="true" android:layout_alignLeft="@+id/txtName" android:layout_alignStart="@+id/txtName" /> <TextView android:text="Status" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/txtStatus" android:layout_above="@+id/txtTalent" android:layout_centerHorizontal="true" android:layout_marginBottom="33dp" /> <TextView android:text="Name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/txtName" android:layout_alignBottom="@+id/txtStatus" android:layout_alignLeft="@+id/txtStatus" android:layout_alignStart="@+id/txtStatus" android:layout_marginBottom="40dp" /> </RelativeLayout>
Edit MainActivity.java class
Last, navigate to the mainactivity class and follow the source code in the below to perform the asynchronous call in your project.
public class MainActivity extends AppCompatActivity { RetrofitManager retrofitManager; private TextView txtName; private TextView txtStatus; private TextView txtTalent; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); txtName = (TextView) findViewById(R.id.txtName); txtStatus = (TextView) findViewById(R.id.txtStatus); txtTalent = (TextView) findViewById(R.id.txtTalent); retrofitManager = new RetrofitManager(); getProfile(); } private void getProfile(){ Subscription subscription = retrofitManager.getDoorService().postRawJson() .observeOn(AndroidSchedulers.mainThread()) .subscribeOn(Schedulers.io()) .subscribe(new Subscriber<Response<ProfileResponse>>() { @Override public void onCompleted() { } @Override public void onError(Throwable t) { } @Override public void onNext(Response<ProfileResponse> profileResponseResponse) { txtName.setText(profileResponseResponse.body().getName().toString()); txtStatus.setText(profileResponseResponse.body().getStatus().toString()); txtTalent.setText(profileResponseResponse.body().getTalent().toString()); } }); } }
Run your Project
In conclusion, the rxjava + retrofit is successfully to implement in your project. You now can check it out.