Android Display GIF Image Tutorial
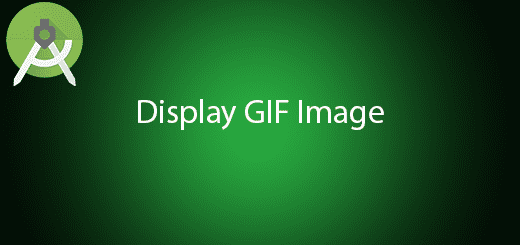
GIF image format (Graphics Interchange Format) is an animated and static image on an application. Besides, It is lossless bitmap image and widely use over the world wide web. Android is able to support and display gif image in the application which can be very useful in specific situation. In this tutorial, I will teach you how to display the gif image in your application, you just need to follow my steps.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “GifExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Sample GIF image
Add a GIF image in Project
Right click res > New > resource directory, make the directory name as “raw”. After that, copy the sample gif image and paste it to the “raw” directory.
Create a Class
Right click project name > New > Java Class and name it as “CustomGifView“.
Edit CustomGifView.java class
Copy and paste the following code to your customgifview.java. This class is to customize the view (gif file) to animation.
package com.example.questdot.gifexample; /** * Created by HP on 20/6/2016. */ import android.content.Context; import android.graphics.Canvas; import android.graphics.Movie; import android.util.AttributeSet; import android.view.View; import java.io.InputStream; /** * Created by HP on 19/6/2016. */ public class CustomGifView extends View { private InputStream gifInputStream; private Movie gifMovie; private int movieWidth, movieHeight; private long movieDuration; private long mMovieStart; public CustomGifView(Context context) { super(context); init(context); } public CustomGifView(Context context, AttributeSet attrs) { super(context, attrs); init(context); } public CustomGifView(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); init(context); } private void init(Context context){ setFocusable(true); gifInputStream = context.getResources() .openRawResource(R.raw.animation); gifMovie = Movie.decodeStream(gifInputStream); movieWidth = gifMovie.width(); movieHeight = gifMovie.height(); movieDuration = gifMovie.duration(); } @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { setMeasuredDimension(movieWidth, movieHeight); } public int getMovieWidth(){ return movieWidth; } public int getMovieHeight(){ return movieHeight; } public long getMovieDuration(){ return movieDuration; } @Override protected void onDraw(Canvas canvas) { long now = android.os.SystemClock.uptimeMillis(); if (mMovieStart == 0) { // first time mMovieStart = now; } if (gifMovie != null) { int dur = gifMovie.duration(); if (dur == 0) { dur = 1000; } int relTime = (int)((now - mMovieStart) % dur); gifMovie.setTime(relTime); gifMovie.draw(canvas, 0, 0); invalidate(); } } }
Edit activity_main.xml layout
Add and use custom view in your activity_main.xml, follow by your package name and class name. eg(com.example.questdot.gifexample.CustomGifView)
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.questdot.gifexample.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" android:id="@+id/textView" /> <com.example.questdot.gifexample.CustomGifView android:layout_gravity="center" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/GIFSingle" android:layout_below="@+id/textView" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> </RelativeLayout>
Edit AndroidManifest.xml
Add android:hardwareAccelerated=”false” in MainActivity, else you will get error when you run your project. Note: Hardware Accelerated cannot use it for drawing lines or curves and other trivial operations.
<activity android:name=".MainActivity" android:hardwareAccelerated="false"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity>
Run Your Project
Now, you can run your project and the application will successfully display the gif image in the screen.