Android Simple Table Layout Tutorial
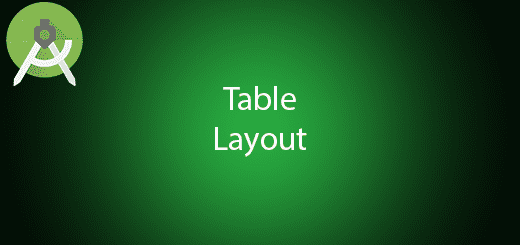
Table Layout is a ViewGroup same as Linear Layout and Relative Layout, it is good for displaying a table in the android application. The feature of table layout is almost similar to the html in the website. It contain row and column to let the developer to customize according their need. In this tutorial, I will teach you some steps to implement android simple table layout tutorial and insert the table row programmatically.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “TableLayoutExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add new xml for table cell shape
Go to res > Right click drawable > new > Layout resource file and name it to cell_shape.xml. After that edit it to the following source code so your table will have the rectangle border.
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape= "rectangle" > <solid android:color="#fff"/> <stroke android:width="1dp" android:color="#000"/> </shape>
Add new xml layout for table row
Go to res > Right click layout > new > Layout resource file and name it to table_item.xml. After that edit it to the following so it will able to add the row into the table layout.
<?xml version="1.0" encoding="utf-8"?> <TableRow xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="wrap_content" android:orientation="horizontal"> <TextView android:layout_width="0dp" android:layout_height="wrap_content" android:id="@+id/tv_no" android:background="@drawable/cell_shape" android:layout_weight="0.25" android:gravity="center"/> <TextView android:layout_width="0dp" android:layout_height="match_parent" android:id="@+id/tv_date" android:background="@drawable/cell_shape" android:layout_weight="0.25" android:gravity="center"/> <TextView android:layout_width="0dp" android:layout_height="match_parent" android:id="@+id/tv_orderid" android:background="@drawable/cell_shape" android:layout_weight="0.25" android:gravity="center"/> <TextView android:layout_width="0dp" android:layout_height="match_parent" android:id="@+id/tv_quantity" android:background="@drawable/cell_shape" android:layout_weight="0.25" android:gravity="center"/> </TableRow>
Edit activity_main.xml layout
Navigate to the activity_main.xml in your layout folder and add the table layout so it will able to show table later.
<?xml version="1.0" encoding="utf-8"?> <TableLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/tableLayout" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="5dp" android:orientation="vertical"> </TableLayout>
Edit MainActivity.java class
Now, you go to your mainactivity.java class to perform some java code so it can add the table_item to the table layout. You can follow the source code in the bottom.
package com.questdot.tablelayoutexample; import android.app.Activity; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.LayoutInflater; import android.view.View; import android.widget.TableLayout; import android.widget.TextView; public class MainActivity extends AppCompatActivity { private TableLayout tableLayout; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); tableLayout=(TableLayout)findViewById(R.id.tableLayout); for (int i=0;i<20;i++){ View tableRow = LayoutInflater.from(this).inflate(R.layout.table_item,null,false); TextView tv_no = (TextView) tableRow.findViewById(R.id.tv_no); TextView tv_date = (TextView) tableRow.findViewById(R.id.tv_date); TextView tv_orderid = (TextView) tableRow.findViewById(R.id.tv_orderid); TextView tv_quantity = (TextView) tableRow.findViewById(R.id.tv_quantity); tv_no.setText(""+(i+1)); tv_date.setText("2016-09-09"); tv_orderid.setText("A00"+(i+1)); tv_quantity.setText(""+(100+(i+1))); tableLayout.addView(tableRow); } } }
Run your project
In the end, you can run the perfect working table layout in your android device or emulator.
res > Right click drawable > new > Layout resource file , this option doesn’t exist