Android Youtube Data API Play Youtube Video Tutorial
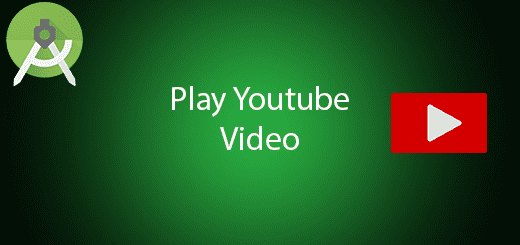
Youtube is now the best video sharing company and there have many active users over the world. Google provide Youtube Data API to let the developer allow to play Youtube video in their mobile application or websites. It is open source and free to use for every developer, its useful for the developer. It just need to know how to use the library to communicate with the API, nothing else to worry about how Youtube works. In this tutorial, I will let you know android Youtube data API play Youtube video in your apps.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “YoutubePlayerExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Google Developer Console
Go to https://console.developers.google.com/ and create a new project for your current application.
Enable Youtube Data API and Create API Key
After that, in the library section and click the youtube data API to enable it, then go to Credentials create an API key.
You will get an API Key. eg
AIzaSyDi97kbUT9Zek8MD495XXvz9oHrUwwPovE
Restrict API Key (Optional)
This is security purpose to let your API key access to specific application. So the hacker will not able to use your API key easily.
- Create KeyStore, (Toolbar > Build > Generate Signted APK > Create New Button)
- By enter “keytool -list -v -keystore (keystore location)” to Get SHA-1 signing-certificate fingerprint to restrict usage to your Android apps.
- Submit your project package name and SHA-1 fingerprint in the Google Developer Console.
Download Youtube Android Player API library
Go to this link to install the zip file for the library https://developers.google.com/youtube/android/player/downloads/. Open the zip file and get the library from lib folder. The name of library is “YoutubeAndroidApi.jar”.
Import the jar library to your project
In the Project section, Copy and paste the jar to your project “libs” folder. You can see the example image in the below.
Add dependency
Add the jar dependency in your project so you can use the library.
Add internet permissions
Add internet permissions in your androidmanifest file so you and load the youtube video.
<uses-permission android:name="android.permission.INTERNET" />
Create two Activities
Right click your project and create two activities which name “YoutubeActivity” and “YoutubeFragment”.
Edit activity_youtube.xml layout
Go to this file and edit the layout to add the youtube player view.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <com.google.android.youtube.player.YouTubePlayerView android:id="@+id/youtube_player_view" android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout>
Edit activity_youtube_fragment.xml layout
Modify this file and add the framelayout so you can add fragment in the class.
<?xml version="1.0" encoding="utf-8"?> <FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/content" android:layout_width="match_parent" android:layout_height="match_parent" />
Edit YoutubeActivity.java class
Go to this class and extends YoutubeBaseActivity and implement YoutubePlayer.OnInitializedListener.
public class YoutubeActivity extends YouTubeBaseActivity implements YouTubePlayer.OnInitializedListener { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_youtube); YouTubePlayerView youTubePlayerView = (YouTubePlayerView) findViewById(R.id.youtube_player_view); youTubePlayerView.initialize(MainActivity.API_KEY, this); } @Override public void onInitializationFailure(YouTubePlayer.Provider provider, YouTubeInitializationResult result) { Toast.makeText(this, "Failed.", Toast.LENGTH_LONG).show(); } @Override public void onInitializationSuccess(YouTubePlayer.Provider provider, YouTubePlayer player, boolean wasRestored) { if(null== player) return; if (!wasRestored) { player.loadVideo(MainActivity.VIDEO_ID); } player.setPlayerStateChangeListener(new YouTubePlayer.PlayerStateChangeListener() { @Override public void onAdStarted() { } @Override public void onError(YouTubePlayer.ErrorReason arg0) { } @Override public void onLoaded(String arg0) { } @Override public void onLoading() { } @Override public void onVideoEnded() { } @Override public void onVideoStarted() { } }); player.setPlaybackEventListener(new YouTubePlayer.PlaybackEventListener() { @Override public void onBuffering(boolean arg0) { } @Override public void onPaused() { } @Override public void onPlaying() { } @Override public void onSeekTo(int arg0) { } @Override public void onStopped() { } }); } }
Edit YoutubeFragment.java class
Modify this java class and add fragment in this class. Follow the source code below.
public class YoutubeFragment extends YouTubeBaseActivity { @Override protected void onCreate(@Nullable Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_youtube_fragment); FragmentManager fm = getFragmentManager(); String tag = YouTubePlayerFragment.class.getSimpleName(); YouTubePlayerFragment playerFragment = (YouTubePlayerFragment) fm.findFragmentByTag(tag); if (playerFragment == null) { FragmentTransaction ft = fm.beginTransaction(); playerFragment = YouTubePlayerFragment.newInstance(); ft.add(android.R.id.content, playerFragment, tag); ft.commit(); } playerFragment.initialize(MainActivity.API_KEY, new YouTubePlayer.OnInitializedListener() { @Override public void onInitializationSuccess(YouTubePlayer.Provider provider, YouTubePlayer youTubePlayer, boolean b) { youTubePlayer.loadVideo(MainActivity.VIDEO_ID); } @Override public void onInitializationFailure(YouTubePlayer.Provider provider, YouTubeInitializationResult youTubeInitializationResult) { Toast.makeText(YoutubeFragment.this, "Failed.", Toast.LENGTH_LONG).show(); } }); } }
Edit activity_main.xml layout
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.questdot.youtubeplayerexample.MainActivity"> <Button android:text="Fragment" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:id="@+id/btnFragment" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <Button android:text="Activity" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/btnActivity" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> </RelativeLayout>
Edit MainActivity.java class
Modify this class, add your API key from the Google Console. And also add the video id from the youtube like https://www.youtube.com/watch?v=(xxxxxx). The xxx is video id in the youtube website.
public class MainActivity extends Activity { Button btnActivity; Button btnFragment; public static final String API_KEY = "AIzaSyDi97kbUT9Zek8MD495XXvz9oHrUwwPovE"; public static final String VIDEO_ID = "Ey_hgKCCYU4"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnActivity = (Button)findViewById(R.id.btnActivity); btnFragment = (Button)findViewById(R.id.btnFragment); btnActivity.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent = new Intent(getApplicationContext(),YoutubeActivity.class); startActivity(intent); } }); btnFragment.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent = new Intent(getApplicationContext(),YoutubeFragment.class); startActivity(intent); } }); } }
Run Your Project
Yeah, you are completed to use the youtube video in your android project. You can test it now.
how to get “VIDEO_ID” from json bcz i dont have static url …every time i have to update dynamic url….so plz let me know how to do dynamic url ….other wise all ur project work well…
Its simple…. you can get VIDEO_ID from any youtube video.
Example : https://www.youtube.com/watch?v=pPkf7hylUuA
pPkf7hylUuA is the video ID 🙂