Android Basic Button Callback Tutorial
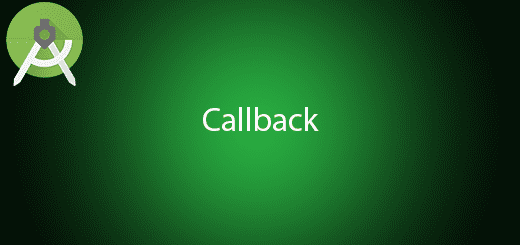
Android callback is very useful when come to specific situation such as button click event. In android, it have many pre-callback method for different purpose such as oncreate, onpause, onresume and more. The callback mean the system will automatically activate the method when the event is occur, so user will dont need to call the method manually. In this tutorial, I will teach you create a callback event in the android application.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “CallbackExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Create a new Interface
Add a new interface by right click the java package > new > new class. Copy and paste the following code in your class.
package com.example.questdot.callbackexample; interface OnToggleClickListener { void onDoClick(); }
Add a new Class
Follow the previous step to create a class and paste the following code. This class is to customize the view so that we can use it in the xml layout.
package com.example.questdot.callbackexample; import android.content.Context; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.graphics.Canvas; import android.util.AttributeSet; import android.view.MotionEvent; import android.view.View; /** * Created by HP on 13/6/2016. */ public class DiyToggle extends View { private Bitmap mBackground; private OnToggleClickListener onToggleClickListener; public DiyToggle(Context context) { super(context); } public DiyToggle(Context context, AttributeSet attrs) { super(context, attrs); } public DiyToggle(Context context, AttributeSet attrs, int defStyleAttr) { super(context, attrs, defStyleAttr); } /* public void setBackground(int resId) { mBackground = BitmapFactory.decodeResource(getResources(), resId); }*/ /* @Override protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) { int measuredWidth = mBackground.getWidth(); int measuredHeight = mBackground.getHeight(); setMeasuredDimension(measuredWidth, measuredHeight); }*/ /* @Override protected void onDraw(Canvas canvas) { if (mBackground != null) { canvas.drawBitmap(mBackground, 0, 0, null); } }*/ public void setToggleClickListener(OnToggleClickListener onToggleClickListener){ if(onToggleClickListener != null){ this.onToggleClickListener = onToggleClickListener; } } @Override public boolean onTouchEvent(MotionEvent event) { int action = event.getAction(); if(action==MotionEvent.ACTION_UP){ onToggleClickListener.onDoClick(); } return true; } }
Edit activity_main.xml layout
Use the customize view in your xml, follow by your package name and view class. Eg as following code.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" > <com.example.questdot.callbackexample.DiyToggle android:id="@+id/mTog" android:layout_width="100dp" android:layout_height="100dp" android:background="@mipmap/ic_launcher" android:layout_centerVertical="true" android:layout_centerHorizontal="true"> </com.example.questdot.callbackexample.DiyToggle> </RelativeLayout>
Edit MainActivity.java class
Go to your mainactivity class and this class will set the listener to the view and perform click event.
package com.example.questdot.callbackexample; import android.app.Activity; import android.os.Bundle; import android.util.Log; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends Activity { private DiyToggle mTog; private TextView mTvCenter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mTog = (DiyToggle) findViewById(R.id.mTog); //mTog.setBackground(R.mipmap.ic_launcher); mTog.setToggleClickListener(new OnToggleClickListener() { @Override public void onDoClick() { Toast.makeText(getApplication(),"Button Clicked",Toast.LENGTH_SHORT).show(); } }); } }
Run your project
After that, now can run your project in the android emulator or android device to test the callback click event.