Android Toolbar Add SearchView Tutorial
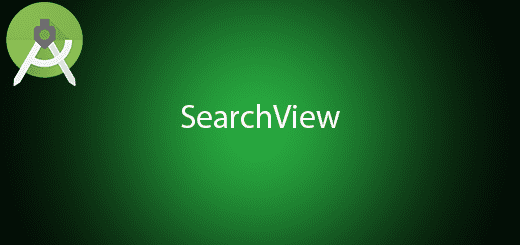
Search feature is a useful tool for the user to search out what they want to see. SearchView is providing in app search for your Android app to improve user experience. Besides, it is widely available to the latest Android N back to Android 2.1. In my tutorial, I will give you SearchView tutorial on how to use it in android application. You just need to follow my steps, no stress no pressure.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “SearchExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add new dependencies
You need to add 3 dependency for appcompt, design and recyclerview. It will be use those library later.
compile 'com.android.support:appcompat-v7:24.0.0' compile 'com.android.support:design:24.0.0' compile 'com.android.support:recyclerview-v7:24.0.0'
Change your Theme
Go to styles.xml to change your application theme. The bottom are the sample source code, I change the parent to NoActionBar because I am using toolbar later.
<resources> <!-- Base application theme. --> <style name="AppTheme" parent="Theme.AppCompat.Light.NoActionBar"> <!-- Customize your theme here. --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> </style> </resources>
Add a new menu
By right click your res folder and create a new menu folder. After that create a new menu xml name it as “menu_main.xml” to use for the main activity later. The following are the new menu xml source code.
<menu xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto"> <item android:id="@+id/action_search" android:icon="@android:drawable/ic_menu_search" android:title="Search" app:actionViewClass="android.support.v7.widget.SearchView" app:showAsAction="always|collapseActionView" /> </menu>
Add a new layout for recyclerview item
Create a new xml layout in the layout folder and name it as “item_row.xml”. After that, edit the xml to the sample source code that i provide in the bottom.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="wrap_content" android:clickable="true" android:focusable="true" android:background="?android:attr/selectableItemBackground" > <TextView android:id="@+id/name" android:text="Text" android:gravity="center" android:layout_width="match_parent" android:layout_height="wrap_content" android:textSize="22dp" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_marginTop="5dp" android:layout_marginLeft="5dp" android:layout_marginBottom="5dp" android:layout_marginRight="5dp" /> </RelativeLayout>
Add a new layout for activity content
After that, add a new layout for your activity main content to display the item. Name the file to “content_main” and Edit the xml file.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="@dimen/activity_horizontal_margin" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:showIn="@layout/activity_main"> <android.support.v7.widget.RecyclerView android:id="@+id/recyclerView" android:layout_width="match_parent" android:layout_height="match_parent"/> </RelativeLayout>
Edit activity_main.xml layout
Modify your activity_main.xml layout to the following sample. This layout is display the toolbar and include the layout of content_main.xml.
<?xml version="1.0" encoding="utf-8"?> <android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" > <android.support.design.widget.AppBarLayout android:layout_width="match_parent" android:layout_height="wrap_content" > <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" /> </android.support.design.widget.AppBarLayout> <include layout="@layout/content_main" /> </android.support.design.widget.CoordinatorLayout>
Add a new Adapter
Right click your java package folder and create a new java class. After that, extends to RecyclerView.Adapter. You can follow sample in the bottom.
public class SearchItemAdapter extends RecyclerView.Adapter<SearchItemAdapter.ViewHolder> { private List<String> originalNamesList; private Context context; public SearchItemAdapter(List<String> names, Context context) { this.originalNamesList = new ArrayList<>(names); this.context = context; } @Override public SearchItemAdapter.ViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { View itemView = LayoutInflater.from(parent.getContext()).inflate(R.layout.list_row, parent, false); return new ViewHolder(itemView); } @Override public void onBindViewHolder(SearchItemAdapter.ViewHolder holder, int position) { holder.tvName.setText(originalNamesList.get(position)); } @Override public int getItemCount() { return originalNamesList.size(); } public class ViewHolder extends RecyclerView.ViewHolder { private TextView tvName; public ViewHolder(View view) { super(view); tvName = (TextView) view.findViewById(R.id.name); } } public List<String> updateData(List<String> datas,String text) { ArrayList<String> newNames = new ArrayList<>(); for (String name : datas) { if (name.contains(text)) { newNames.add(name); } } return newNames; } public void setItems(List<String> datas){ originalNamesList = new ArrayList<>(datas); } }
Edit MainActivity.java class
Modify your mainactivity.java class and implements the serchview.onquerytextlistener. I add some dummy item to test out the search feature.
public class MainActivity extends AppCompatActivity implements SearchView.OnQueryTextListener { private RecyclerView recyclerView; private SearchItemAdapter adapter; private ArrayList<String> sampleList; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar); setSupportActionBar(toolbar); sampleList = new ArrayList<>(); recyclerView = (RecyclerView) findViewById(R.id.recyclerView); recyclerView.setHasFixedSize(true); RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(this); recyclerView.setLayoutManager(layoutManager); addItem(); adapter = new SearchItemAdapter(sampleList, this); recyclerView.setAdapter(adapter); adapter.notifyDataSetChanged(); } public void addItem(){ sampleList.add("android"); sampleList.add("better"); sampleList.add("coklate"); sampleList.add("dog"); sampleList.add("east"); sampleList.add("fun"); sampleList.add("gap"); sampleList.add("happy"); sampleList.add("illusion"); } @Override public boolean onCreateOptionsMenu(Menu menu) { MenuInflater menuInflater = getMenuInflater(); menuInflater.inflate(R.menu.menu_main, menu); SearchView searchView = (SearchView) MenuItemCompat.getActionView(menu.findItem(R.id.action_search)); searchView.setOnQueryTextListener(this); return super.onCreateOptionsMenu(menu); } @Override public boolean onOptionsItemSelected(MenuItem item) { int id = item.getItemId(); if (id == R.id.action_search) { return true; } return super.onOptionsItemSelected(item); } @Override public boolean onQueryTextSubmit(String query) { return false; } @Override public boolean onQueryTextChange(String newText) { List<String> filteredModelList = adapter.updateData(sampleList,newText); adapter.setItems(filteredModelList); adapter.notifyDataSetChanged(); recyclerView.scrollToPosition(0); return true; } }
Run your project
Lastly, you finish the search feature in this project, now you can test out the search feature in your real device.