Android EventBus Publish Subscribe Tutorial
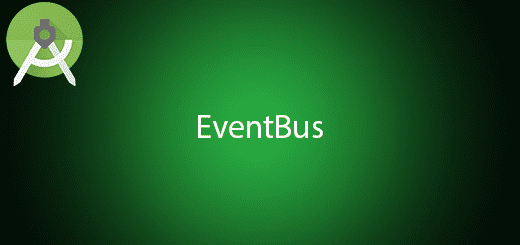
What is event bus? Eventbus is an android library that enables the developer easier to communicate between the components such as activities, fragments, services and more. It is very simple to use, less code and produce better quality in the Android application. Eventbus is very popular to use in the worldwide and this library creates by the famous GreenRobot. In this tutorial, I will tell you how to use android Eventbus publish subscribe the event on your android project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “EventBusExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add eventbus dependency
Go to your build.gradle (Module:app) and add one dependency in the dependencies section.
compile 'org.greenrobot:eventbus:3.0.0'
Create a Modal
Right-click your java package name and create a new modal class “User”. After that add three attributes in this class which are the name, phone, and email.
public class User { String name; String phone; String email; public String getName() { return name; } public User(String name, String phone, String email) { this.name = name; this.phone = phone; this.email = email; } public void setName(String name) { this.name = name; } public String getPhone() { return phone; } public void setPhone(String phone) { this.phone = phone; } public String getEmail() { return email; } public void setEmail(String email) { this.email = email; } }
Create a new empty activity
Right-click your project and create an empty activity for “SecondActivity” in your project. After that edit your activity_second.xml to the following source
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="//schemas.android.com/apk/res/android" xmlns:tools="//schemas.android.com/tools" android:id="@+id/activity_second" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.questdot.eventbusexample.SecondActivity"> <TextView android:text="TextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:id="@+id/txtName" /> <TextView android:text="TextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_alignLeft="@+id/txtName" android:layout_alignStart="@+id/txtName" android:id="@+id/txtEmail" /> <TextView android:text="TextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignLeft="@+id/txtEmail" android:layout_alignStart="@+id/txtEmail" android:id="@+id/txtPhone" /> </RelativeLayout>
Edit SecondActivity.java class
Open your secondactivity.java class and follow the example source in the below. This activity subscribes the event from the main activity.
public class SecondActivity extends AppCompatActivity { private TextView txtName,txtPhone,txtEmail; private EventBus bus = EventBus.getDefault(); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_second); txtName = (TextView) findViewById(R.id.txtName); txtPhone = (TextView) findViewById(R.id.txtPhone); txtEmail = (TextView) findViewById(R.id.txtEmail); } @Subscribe(sticky = true, threadMode = ThreadMode.MAIN) public void getUser(User event){ txtName.setText( event.getName()); txtPhone.setText(event.getPhone()); txtEmail.setText(event.getEmail()); } @Override public void onStart() { super.onStart(); bus.register(this); } @Override public void onStop() { bus.unregister(this); super.onStop(); } }
Edit mainactivity.xml layout
This XML file will add some edited text to publish the event in the main activity.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="//schemas.android.com/apk/res/android" xmlns:tools="//schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.questdot.eventbusexample.MainActivity"> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textPersonName" android:text="Name" android:ems="10" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/etName" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textEmailAddress" android:ems="10" android:id="@+id/etEmail" android:text="Email" android:layout_below="@+id/etName" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="phone" android:ems="10" android:id="@+id/etPhone" android:layout_below="@+id/etEmail" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" android:text="Phone" /> <TextView android:text="TextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_alignLeft="@+id/txtName" android:layout_alignStart="@+id/txtName" android:id="@+id/txtEmail" /> <Button android:text="Post Sticky" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/btnPostSticky" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <Button android:text="POST" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_above="@+id/btnPostSticky" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_marginBottom="11dp" android:id="@+id/btnPost" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <TextView android:text="TextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/txtName" android:layout_above="@+id/txtEmail" android:layout_centerHorizontal="true" /> <TextView android:text="TextView" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/txtPhone" android:layout_below="@+id/txtEmail" android:layout_alignLeft="@+id/txtEmail" android:layout_alignStart="@+id/txtEmail" /> </RelativeLayout>
Edit MainActivity.java
Go to the mainactivity.java class and edit the following source code. This activity registers the event bus in the onstart method. PostSticky means it will store for the future use until the app is destroyed while Post only able to receive the class.
public class MainActivity extends AppCompatActivity { private Button btnPostSticky,btnPost; private EditText etName,etPhone,etEmail; private EventBus bus = EventBus.getDefault(); private TextView txtName,txtPhone,txtEmail; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnPostSticky = (Button) findViewById(R.id.btnPostSticky); btnPost = (Button) findViewById(R.id.btnPost); etName = (EditText) findViewById(R.id.etName); etPhone = (EditText) findViewById(R.id.etPhone); etEmail = (EditText) findViewById(R.id.etEmail); txtName = (TextView) findViewById(R.id.txtName); txtPhone = (TextView) findViewById(R.id.txtPhone); txtEmail = (TextView) findViewById(R.id.txtEmail); btnPostSticky.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (etName.getText().toString().isEmpty()||etPhone.getText().toString().isEmpty()||etPhone.getText().toString().isEmpty()) { Toast.makeText(getApplicationContext(),"Please fill in all empty field",Toast.LENGTH_LONG).show(); } else { bus.postSticky(new User(etName.getText().toString(),etPhone.getText().toString(),etEmail.getText().toString())); startActivity(new Intent(MainActivity.this, SecondActivity.class)); } } }); btnPost.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (etName.getText().toString().isEmpty()||etPhone.getText().toString().isEmpty()||etPhone.getText().toString().isEmpty()) { Toast.makeText(getApplicationContext(),"Please fill in all empty field",Toast.LENGTH_LONG).show(); } else { bus.post(new User(etName.getText().toString(),etPhone.getText().toString(),etEmail.getText().toString())); } } }); } @Override public void onStart() { super.onStart(); bus.register(this); } @Override public void onStop() { bus.unregister(this); super.onStop(); } @Subscribe(threadMode = ThreadMode.MAIN) public void getUser(User event){ txtName.setText( event.getName()); txtPhone.setText(event.getPhone()); txtEmail.setText(event.getEmail()); } }
Run Your Project
At last, you can run this project in your android studio and test it out in the emulator or real android device to check out the features.