Android SurfaceView Moving Rectangle Tutorial
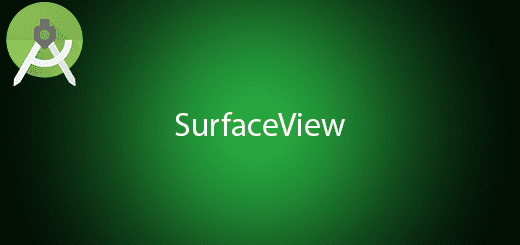
SurfaceView is a custom view that able to update the GUI rapidly such as rendering the animation in the activity. Android view is different with surfaceView which cannot update the GUI. When we need to use surfaceview? We can use the surfaceView with camera or playing the video. Besides that, we also can use canvas to draw image every second and update the GUI so the image will be look like its moving on. In this tutorial, I will teach you how to use android surfaceview and draw a rectangle on it to keep update it every second.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “SurfaceViewExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit MainActivity.java class
After create your project, go to the mainactivity.java class and copy paste the source code in the bottom. In this class I will add one more class which name “RectangleView” and extends it to SurfaceView and implements SurfaceHolder Callback. When the SurfaceView is created, I will start the timer and it will be stop the timer when surfaceview is destroyed.
For the timer schedule, I set the delay and period to 100 milliseconds which is 0.1 seconds.
In the draw() method, I draw the rectangle which has 100 width and height and updating the rectangle by adding y-axis which is vertical.
public class MainActivity extends Activity { RectangleView myview; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); myview = new RectangleView(this); setContentView(myview); } @Override protected void onPause() { super.onPause(); myview.stopTimer(); } public class RectangleView extends SurfaceView implements SurfaceHolder.Callback { private float x = 0, y = 0; private Paint paint = null; public RectangleView(Context context) { super(context); paint = new Paint(); paint.setColor(Color.RED); getHolder().addCallback(this); } public void draw() { Canvas canvas = getHolder().lockCanvas(); canvas.drawColor(Color.WHITE); canvas.save(); canvas.translate(x, y); canvas.drawRect(0, 0, 100, 100, paint); canvas.restore(); getHolder().unlockCanvasAndPost(canvas); y++; } private Timer timer = null; private TimerTask task = null; public void startTimer() { timer = new Timer(); task = new TimerTask() { @Override public void run() { draw(); } }; timer.schedule(task, 100, 100); } public void stopTimer() { if (timer != null) { timer.cancel(); timer = null; } } @Override public void surfaceChanged(SurfaceHolder holder, int format, int width, int height) { } @Override public void surfaceCreated(SurfaceHolder holder) { startTimer(); } @Override public void surfaceDestroyed(SurfaceHolder holder) { stopTimer(); } } }
Run Your Project
In conclusion, now you can run this project to see what will be happen in your activity. You will see the rectangle moving down every 0.1 seconds.
Here we have only one image and one direction, if there are multiple, images and multiple different directions for each image, how can we do this.
I came to this tut for changing the bitmap drawn on an image every second