Android Persistent and Modal Bottom Sheet Tutorial
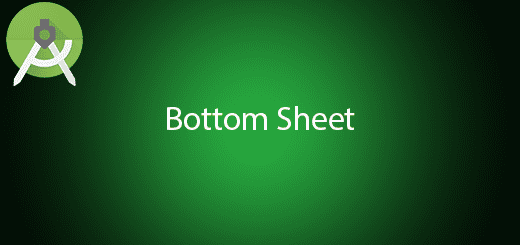
Bottom Sheet is the new material design widget and it is a simple bottom window display in the bottom of the screen. There have two type of Bottom Sheet which are Persistent and Modal. The first type Persistent Bottom Sheet is structural part of a User Interface and it is permanent in the activity, user can expand and collapse the view. While the second type Modal Bottom Sheet is a temporary dialog that display in the screen, it is not a part of view hierarchy. In this tutorial, I will teach you on how to create the new android persistent and modal bottom sheet in your project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “BottomSheetExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add new dependency
Open your build.gradle file and Add new library in your dependency section.
compile 'com.android.support:design:25.1.1'
Create Custom Modal Bottom Sheet Layout
Right click the layout folder and create a new xml file “custom_bottom_sheet_dialog”.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:id="@+id/bottomSheetLayout" android:layout_width="match_parent" android:layout_height="300dp" android:background="@android:color/holo_red_light" android:padding="16dp" app:behavior_peekHeight="60dp" app:layout_behavior="@string/bottom_sheet_behavior"> <!-- app:behavior_hideable="true"--> <TextView android:id="@+id/bottomSheetHeading" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Any Title" android:textAppearance="@android:style/TextAppearance.Large" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="This is Modal Bottom Sheet Dialog" android:textAppearance="@android:style/TextAppearance.Large" android:layout_below="@+id/bottomSheetHeading" android:layout_centerHorizontal="true" android:layout_marginTop="79dp" /> </RelativeLayout>
Create Custom Persistent Bottom Sheet Layout
After create the modal bottom sheet layout, and create a new custom layout for persistent bottom sheet. The file name will be “content_bottom_sheet”.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" android:id="@+id/bottomSheetLayout" android:layout_width="match_parent" android:layout_height="300dp" android:background="@android:color/holo_blue_dark" android:padding="16dp" app:behavior_peekHeight="60dp" app:layout_behavior="@string/bottom_sheet_behavior"> <TextView android:id="@+id/bottomSheetHeading" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Expand Me" android:textAppearance="@android:style/TextAppearance.Large" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="This is Persistent Bottom Sheet" android:textAppearance="@android:style/TextAppearance.Large" android:layout_centerVertical="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> </RelativeLayout>
Create layout for mainactivity content
Add a new xml file “content_main” for your activity content.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" app:layout_behavior="@string/appbar_scrolling_view_behavior" tools:context="com.questdot.bottomsheetexample.MainActivity" tools:showIn="@layout/activity_main"> <Button android:id="@+id/show_bottom_sheet_dialog_button" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Show Modal Bottom Sheet" /> </RelativeLayout>
Edit activity_main.xml layout
This layout will include the content_main and content_bottom_sheet.
<?xml version="1.0" encoding="utf-8"?> <android.support.design.widget.CoordinatorLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:fitsSystemWindows="true" > <android.support.design.widget.AppBarLayout android:layout_width="match_parent" android:layout_height="wrap_content" > <android.support.v7.widget.Toolbar android:id="@+id/toolbar" android:layout_width="match_parent" android:layout_height="?attr/actionBarSize" android:background="?attr/colorPrimary" /> </android.support.design.widget.AppBarLayout> <include layout="@layout/content_main" /> <include layout="@layout/content_bottom_sheet" /> </android.support.design.widget.CoordinatorLayout>
Create a new class for Custom Modal Bottom Sheet
Right click your package name and add a new class “CustomBottomSheetDialogFragment”. Aftert that follow the source code below.
import android.os.Bundle; import android.support.design.widget.BottomSheetDialogFragment; import android.view.LayoutInflater; import android.view.View; import android.view.ViewGroup; public class CustomBottomSheetDialogFragment extends BottomSheetDialogFragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View v = inflater.inflate(R.layout.custom_bottom_sheet_dialog, container, false); return v; } }
Edit MainActivity.java class
Open your MainActivity.java class and edit it to the source code below.
public class MainActivity extends AppCompatActivity implements View.OnClickListener { private BottomSheetBehavior bottomSheetBehavior; private Button showBottomSheetDialogButton; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); Toolbar toolbar = (Toolbar) findViewById(R.id.toolbar); setSupportActionBar(toolbar); bottomSheetBehavior = BottomSheetBehavior.from(findViewById(R.id.bottomSheetLayout)); showBottomSheetDialogButton = (Button) findViewById(R.id.show_bottom_sheet_dialog_button); showBottomSheetDialogButton.setOnClickListener(this); bottomSheetBehavior.setBottomSheetCallback(new BottomSheetBehavior.BottomSheetCallback() { @Override public void onStateChanged(View bottomSheet, int newState) { if (newState == BottomSheetBehavior.STATE_EXPANDED) { // bottomSheetHeading.setText(getString(R.string.text_collapse_me)); } else { // bottomSheetHeading.setText(getString(R.string.text_expand_me)); } switch (newState) { case BottomSheetBehavior.STATE_COLLAPSED: Log.e("Bottom Sheet Behaviour", "STATE_COLLAPSED"); break; case BottomSheetBehavior.STATE_DRAGGING: Log.e("Bottom Sheet Behaviour", "STATE_DRAGGING"); break; case BottomSheetBehavior.STATE_EXPANDED: Log.e("Bottom Sheet Behaviour", "STATE_EXPANDED"); break; case BottomSheetBehavior.STATE_HIDDEN: Log.e("Bottom Sheet Behaviour", "STATE_HIDDEN"); break; case BottomSheetBehavior.STATE_SETTLING: Log.e("Bottom Sheet Behaviour", "STATE_SETTLING"); break; } } @Override public void onSlide(View bottomSheet, float slideOffset) { } }); } @Override public void onClick(View v) { switch (v.getId()) { case R.id.show_bottom_sheet_dialog_button: new CustomBottomSheetDialogFragment().show(getSupportFragmentManager(), "Dialog"); break; } } }
Run Your Project
Finally you get it done ! You can run this project in your android device or emulator to test the persistent and modal bottom sheet.
Thank you for the great tutorial!