Building Unit and Instrumental Tests on Android
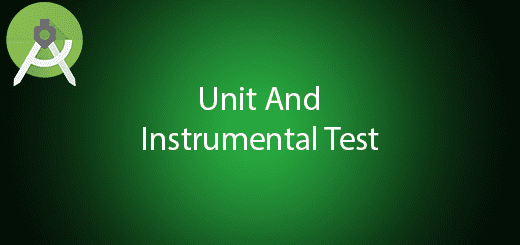
In Android Studio, Its support 2 type of Testing which are Unit and Instrumental test. What difference between unit and instrumental test? Unit tests is testing a Class or Method for its behaviour and it run on your local machine only. These tests are compiled to run locally on the JVM to minimise execution time. Instrumental tests is a User Interface testing that run on an Android device or emulator. These tests have access to Instrumentation information such as Context of the application. It also allow to control the life cycle and the event of the activity. In this tutorial, I will teach you how to building unit and instrumental tests on Android project. We will create the simple unit and instrumental test to test the application.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “InstrumentalUnitTestExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
After you create the project, the android will automatic add the unit and instrumental test in your gradle. There are Junit (Unit Test) and Espresso (Instrumental Test)
Edit MainActivity.java class
Open this file and add the sample method for unit testing the method is it working a not. You can follow the below source code.
public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public static int addNumber(int a,int b){ return a+b; } }
Edit ExampleInstrumentedTest.java class
Modify this class, this class is in your package name “xxx”(androidTest). Add the TestRule so this class will simulate the Activity and test out the method.
@RunWith(AndroidJUnit4.class) public class ExampleInstrumentedTest { @Rule public ActivityTestRule<MainActivity> mActivityRule = new ActivityTestRule(MainActivity.class); @Test public void useAppContext() throws Exception { // Context of the app under test. Context appContext = InstrumentationRegistry.getTargetContext(); assertEquals("com.questdot.instrumentalunittestexample", appContext.getPackageName()); } @Test public void shouldBeAbleToLaunch() throws Exception { onView(withText("Hello World!")).check(matches(isDisplayed())); } }
Edit ExampleUnitTest.java class
Find this class in your package name “xxx”(test). Add Extra method to test the method from MainActivity.
public class ExampleUnitTest { @Test public void addition_isCorrect() throws Exception { assertEquals(4, 2 + 2); } @Test public void Test() throws Exception{ int result =MainActivity.addNumber(1,2); assertEquals(3,result); } }
Tips
You can refer the Espresso cheat sheet for the quick reference to perform your test case. This cheat sheet contains most available Matchers
, ViewActions
and ViewAssertions
.
Run Your Test
In conclusion, the unit and instrumental test has been done to implement, you can try it out. For Unit and Instrumental Test, Right click the Class and click Run XXX. It will run the test for the class. You can refer the following picture.