Android Send and Read SMS Tutorial
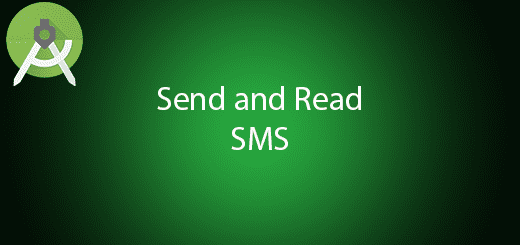
In android application, SMS allow you to send to another smartphone user. While you can also implement a broadcast receiver to read the sms receive in the smartphone. Send and Read SMS may useful in some situation in mobile application. Developer can custom a mobile application to send sms by replace the original send sms application in your smartphone. In this tutorial, I will tell you how to use android send and read sms in your project, its just simple.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “SendReadSMSExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit activity_main.xml layout
I will add 2 edittext in the layout, the first edittext is to enter the number of user and another field is the message that you wish to send. Besides that, I also add button to perform send sms feature. I also add 2 textview to read the incoming message send by other user or yourself.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <EditText android:id="@+id/editTextPhoneNo" android:layout_width="fill_parent" android:layout_height="wrap_content" android:inputType="phone" android:hint="Phone" /> <EditText android:id="@+id/editTextSMS" android:layout_width="fill_parent" android:layout_height="wrap_content" android:inputType="textMultiLine" android:hint="Message" /> <Button android:id="@+id/btnSendSMS" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Send SMS"/> <RelativeLayout android:layout_width="match_parent" android:layout_height="match_parent"> <TextView android:text="Message" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/txtMessage" android:layout_centerVertical="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <TextView android:text="From" android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/txtFrom" android:layout_above="@+id/txtMessage" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_marginBottom="13dp" /> </RelativeLayout> </LinearLayout>
Add a new broadcast receiver
Right click your java package and create a new broadcastreceiver to read your message. You can follow the sample code in the bottom.
public class SmsBroadcastReceiver extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { if(intent.getAction().equals("android.provider.Telephony.SMS_RECEIVED")){ Bundle bundle = intent.getExtras(); SmsMessage[] msgs = null; String msg_from; if (bundle != null){ try{ Object[] pdus = (Object[]) bundle.get("pdus"); msgs = new SmsMessage[pdus.length]; for(int i=0; i<msgs.length; i++){ msgs[i] = SmsMessage.createFromPdu((byte[])pdus[i]); msg_from = msgs[i].getOriginatingAddress(); String msgBody = msgs[i].getMessageBody(); if (MainActivity.mThis != null) { ((TextView) MainActivity.mThis.findViewById(R.id.txtFrom)).setText(msg_from); ((TextView) MainActivity.mThis.findViewById(R.id.txtMessage)).setText(msgBody); } } }catch(Exception e){ } } } } }
Edit AndroidManifest.xml
Add receiver in the application section and add SMS permissions as define in the bottom.
<uses-permission android:name="android.permission.SEND_SMS"/> <uses-permission android:name="android.permission.READ_SMS" /> <uses-permission android:name="android.permission.RECEIVE_SMS" /> <application android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <receiver android:name=".SmsBroadcastReceiver"> <intent-filter > <action android:name="android.provider.Telephony.SMS_RECEIVED" /> </intent-filter> </receiver> </application>
Edit MainActivity.java class
Lastly, go to your mainactivity.java and this class will perform read and send sms function in your project. The source code bottom is the way to implement it.
import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.telephony.SmsManager; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; public class MainActivity extends AppCompatActivity { Button sendBtn; EditText edPhone; EditText edMessage; public static MainActivity mThis = null; @Override protected void onResume() { super.onResume(); mThis = this; } @Override protected void onPause() { super.onPause(); mThis = null; } @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); sendBtn = (Button) findViewById(R.id.btnSendSMS); edPhone = (EditText) findViewById(R.id.editTextPhoneNo); edMessage = (EditText) findViewById(R.id.editTextSMS); sendBtn.setOnClickListener(new View.OnClickListener() { public void onClick(View view) { sendSMS(); } }); } protected void sendSMS() { String toPhoneNumber = edPhone.getText().toString(); String smsMessage = edMessage.getText().toString(); try { SmsManager smsManager = SmsManager.getDefault(); smsManager.sendTextMessage(toPhoneNumber, null, smsMessage, null, null); Toast.makeText(getApplicationContext(), "SMS sent success.", Toast.LENGTH_LONG).show(); } catch (Exception e) { Toast.makeText(getApplicationContext(), "Sending SMS failed.", Toast.LENGTH_LONG).show(); e.printStackTrace(); } } }
Run your project
Finally, you can try to send sms to yourself or your friend, it will charge a cost in your sims. You can also read sms in the application.