Android Simple Dialog Fragment Tutorial
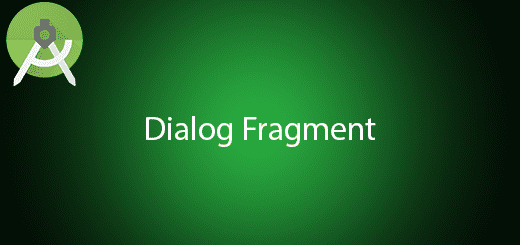
A Dialog Fragment is a fragment that can be use as a fragment and also showing as a dialog in your activity. It will be same as a fragment lifecycle when it create. Therefore, Google have introduce new Dialog Fragment to overcome the disadvantages of using Dialog. Why choose Dialog Fragment over Dialog? DialogFragment has lifecycle management for configuration changes such as screen rotation. It will restore the state if your app process is killed in the background and user returns to it later. If you using Dialog you will need to handle that situation in another way. In this tutorial, I will teach you how to use dialog fragment on your android project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “DialogFragmentExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Create a custom dialog
Right click your layout folder and create a new xml file “custom_df.xml” for the dialogfragment. After that, copy the source code below to your xml file. This is a sample layout you want to show in this dialog.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:padding="10dp" android:text="Your Custom Dialog Fragment!" /> </RelativeLayout>
Create a new Custom Dialog Fragment class
Right click your package name from your java folder and create a new class “CustomDFragment”. This class will be show the layout of the custom_df.xml and extends the DialogFragment class.
public class CustomDFragment extends DialogFragment { @Override public View onCreateView(LayoutInflater inflater, ViewGroup container, Bundle savedInstanceState) { View rootView = inflater.inflate(R.layout.custom_df, container, false); getDialog().setTitle("DialogFragment Tutorial"); return rootView; } }
Create another class for AlertDialog
Create another class “AlertDFragment”, This class will recreate dialog to alert dialog. So this alertdialog will have the lifecycle of fragment.
public class AlertDFragment extends DialogFragment { @Override public Dialog onCreateDialog(Bundle savedInstanceState) { return new AlertDialog.Builder(getActivity()) .setIcon(R.mipmap.ic_launcher) .setTitle("Alert DialogFragment") .setMessage("Alert DialogFragment Tutorial") .setPositiveButton("OK", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { } }) .setNegativeButton("Cancel", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { } }).create(); } }
Edit activity_main.xml layout
Modify your activity_main.xml to the following source code, this layout will have two buttons on it.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="match_parent" > <Button android:id="@+id/btn_cd" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Custom Dialog" /> <Button android:id="@+id/btn_ad" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Alert Dialog" /> </LinearLayout>
Edit MainActivity.java class
Copy and paste the sample below, it will be showing the custom dialog fragment and alert dialog fragment.
public class MainActivity extends AppCompatActivity { Button btn_cd; Button btn_ad; FragmentManager fm = getSupportFragmentManager(); @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btn_cd = (Button) findViewById(R.id.btn_cd); btn_ad = (Button) findViewById(R.id.btn_ad); btn_cd.setOnClickListener(new OnClickListener() { public void onClick(View arg0) { CustomDFragment dFragment = new CustomDFragment(); dFragment.show(fm, "Custom Dialog Fragment"); } }); btn_ad.setOnClickListener(new OnClickListener() { public void onClick(View arg0) { AlertDFragment alertdFragment = new AlertDFragment(); alertdFragment.show(fm, "Alert Dialog Fragment"); } }); } }
Edit styles.xml
Dont forgot to edit styles.xml, you should edit the style of Dialog if your dialog title is not showing in your dialog fragment.
<resources> <!-- Base application theme. --> <style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar"> <!-- Customize your theme here. --> <item name="colorPrimary">@color/colorPrimary</item> <item name="colorPrimaryDark">@color/colorPrimaryDark</item> <item name="colorAccent">@color/colorAccent</item> <item name="android:dialogTheme">@style/CustomDialog</item> </style> <style name="CustomDialog" parent="@style/Theme.AppCompat.Light.Dialog"> <item name="android:windowNoTitle">false</item> </style> </resources>
Run Your Project
Lastly, you can try the simple dialog Fragment in your android device. Run it and test it right now.