Android Slider ViewPager Tutorial
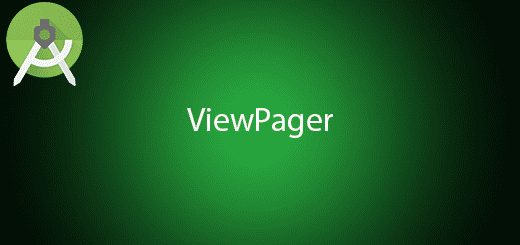
Android ViewPager allow the user to swipe left or right for navigate to another page or view in the android application. It mostly use to combine with fragments because it let the user switch to another fragment just swipe by finger. In this tutorial, I will give you some viewpager tutorial steps in the android application. If you are beginner, you are in the right place.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “ViewPagerExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add a New Class
Right click the package name and add the new class name as “ViewPagerAdapter”.
Edit ViewPagerAdapter.java class
Go to the file and edit to the source code below. This class should be extends to the pageradapter.
package com.example.questdot.viewpagerexample; import android.content.Context; import android.support.v4.view.PagerAdapter; import android.support.v4.view.ViewPager; import android.view.View; import java.util.List; public class ViewPagerAdapter extends PagerAdapter { private List<View> views; private Context context; public ViewPagerAdapter(List<View> views, Context context) { this.views = views; this.context = context; } @Override public void destroyItem(View container, int position, Object object) { ((ViewPager) container).removeView(views.get(position)); } @Override public Object instantiateItem(View container, int position) { ((ViewPager) container).addView(views.get(position)); return views.get(position); } @Override public int getCount() { return views.size(); } @Override public boolean isViewFromObject(View arg0, Object arg1) { return (arg0 == arg1); } }
Add 3 diferent xml layout
Go to res > right click layout > new >layout resource file. First recource file name is one, second is two, the last xml is three.
Edit one,two, three.xml layout
Go to those 3 file and edit to the following code. I change all the relativelayout to the linearlayout and add one textview inside it.
one.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="50dp" android:text="1" android:layout_gravity="center_horizontal" /> </LinearLayout>
two.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="50dp" android:text="2" android:layout_gravity="center_horizontal" /> </LinearLayout>
three.xml
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textSize="50dp" android:text="3" android:layout_gravity="center_horizontal" /> </RelativeLayout>
Edit mainactivity.xml layout
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" > <android.support.v4.view.ViewPager android:id="@+id/viewpager" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="#00000000" > </android.support.v4.view.ViewPager> <LinearLayout android:id="@+id/ll" android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:gravity="center_horizontal" android:orientation="horizontal" > <ImageView android:id="@+id/iv1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/login_point_selected" /> <ImageView android:id="@+id/iv2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/login_point" /> <ImageView android:id="@+id/iv3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:src="@drawable/login_point" /> </LinearLayout> </RelativeLayout>
Add 2 image in drawable folder
Add two indicator image to your drawable folder by copy the image and paste it into the folder.
Edit MainActivity.java class
Go to the mainactivity.java class and edit to the following code.
package com.example.questdot.viewpagerexample; import java.util.ArrayList; import java.util.List; import android.app.Activity; import android.content.Intent; import android.os.Bundle; import android.support.v4.view.ViewPager; import android.support.v4.view.ViewPager.OnPageChangeListener; import android.view.LayoutInflater; import android.view.View; import android.view.View.OnClickListener; import android.widget.Button; import android.widget.ImageView; public class MainActivity extends Activity implements OnPageChangeListener { private ViewPager vp; private ViewPagerAdapter vpAdapter; private List<View> views; private ImageView[] dots; private int[] ids = { R.id.iv1, R.id.iv2, R.id.iv3 }; private Button start_btn; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initViews(); initDots(); } private void initViews() { LayoutInflater inflater = LayoutInflater.from(this); views = new ArrayList<View>(); views.add(inflater.inflate(R.layout.one, null)); views.add(inflater.inflate(R.layout.two, null)); views.add(inflater.inflate(R.layout.three, null)); vpAdapter = new ViewPagerAdapter(views, this); vp = (ViewPager) findViewById(R.id.viewpager); vp.setAdapter(vpAdapter); vp.setOnPageChangeListener(this); } private void initDots() { dots = new ImageView[views.size()]; for (int i = 0; i < views.size(); i++) { dots[i] = (ImageView) findViewById(ids[i]); } } @Override public void onPageScrollStateChanged(int arg0) { // TODO Auto-generated method stub } @Override public void onPageScrolled(int arg0, float arg1, int arg2) { // TODO Auto-generated method stub } @Override public void onPageSelected(int arg0) { for (int i = 0; i < ids.length; i++) { if (arg0 == i) { dots[i].setImageResource(R.drawable.login_point_selected); } else { dots[i].setImageResource(R.drawable.login_point); } } } }
Run your project
You now can run your project in the android emulator. You able to use the viewpager ability in the android application.