How to Scan and Generate QR Code in Android
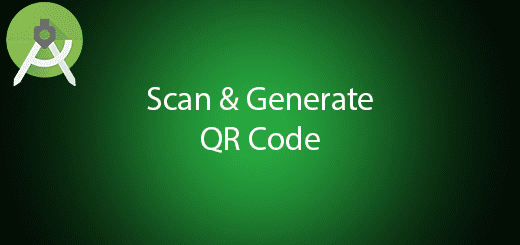
QR code is 2 Dimensional bar code that first designed for Automotive industry in Japan. The QR Code contain information about the item, it able to generate by using a readable human text and decode a QR Code to a readable human text. QR Code is to make the machine easier to understand the information of the specific item by scanning though it. It is far more popular and useful in the world right now. In this tutorial, I will tell you how to scan and generate QR Code in android by using zxing library so you can use it as a feature in your future project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “ZxingExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add a new dependency
After creating a new project, go to add the new dependency for embeded zxing library so you can use zxing in your project.
compile 'com.journeyapps:zxing-android-embedded:3.4.0'
Edit activity_main.xml layout
Then go to the activity_main.xml and add two buttons,one edit text and one imageview in the relative layout such as the source code in the bottom.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" > <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Scan" android:id="@+id/btnScan" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <ImageView android:layout_width="wrap_content" android:layout_height="wrap_content" app:srcCompat="@mipmap/ic_launcher" android:layout_below="@+id/btnGenerate" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_marginTop="24dp" android:id="@+id/imageView" android:layout_alignParentBottom="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:inputType="textPersonName" android:ems="10" android:layout_below="@+id/btnScan" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:id="@+id/editText" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" android:hint="Enter to text to generate" /> <Button android:text="Generate" android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/btnGenerate" android:layout_below="@+id/editText" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> </RelativeLayout> <br>
Edit MainActivity.java class
Last, open the mainactivity class and perform the scan and generate qr code feature. You can copy and paste the sample code below.
public class MainActivity extends AppCompatActivity { private Button btnScan,btnGenerate; private EditText editText; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnScan = (Button) findViewById(R.id.btnScan); btnGenerate = (Button) findViewById(R.id.btnGenerate); editText = (EditText) findViewById(R.id.editText); btnScan.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { IntentIntegrator integrator = new IntentIntegrator(MainActivity.this); integrator.setDesiredBarcodeFormats(IntentIntegrator.ALL_CODE_TYPES); integrator.setPrompt("Scan"); integrator.setCameraId(0); integrator.setBarcodeImageEnabled(false); integrator.initiateScan(); } }); btnGenerate.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if(!editText.getText().toString().isEmpty()){ generateQRCode(editText.getText().toString()); } else{ Toast.makeText(getApplicationContext(), "Please enter text", Toast.LENGTH_LONG).show(); } } }); } public void generateQRCode(String name){ QRCodeWriter writer = new QRCodeWriter(); try { BitMatrix bitMatrix = writer.encode(name, BarcodeFormat.QR_CODE, 512, 512); int width = bitMatrix.getWidth(); int height = bitMatrix.getHeight(); Bitmap bmp = Bitmap.createBitmap(width, height, Bitmap.Config.RGB_565); for (int x = 0; x < width; x++) { for (int y = 0; y < height; y++) { bmp.setPixel(x, y, bitMatrix.get(x, y) ? Color.BLACK : Color.WHITE); } } ((ImageView) findViewById(R.id.imageView)).setImageBitmap(bmp); } catch (WriterException e) { e.printStackTrace(); } } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { IntentResult result = IntentIntegrator.parseActivityResult(requestCode, resultCode, data); if(result != null) { if(result.getContents() == null) { Toast.makeText(this, "Cancelled", Toast.LENGTH_LONG).show(); } else { Toast.makeText(this, "Scanned: " + result.getContents(), Toast.LENGTH_LONG).show(); } } else { // This is important, otherwise the result will not be passed to the fragment super.onActivityResult(requestCode, resultCode, data); } } }
Run your Project
Finally, the qr scanner and generator has been implement until the end, now its time to run the project in your emulator or android device.