Android Foreground Service Tutorial
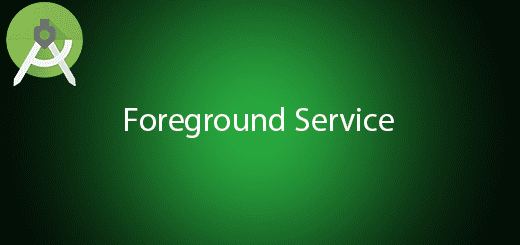
Android service not only can running in the background, it also possible running in the foreground by using notification and startForeground() method. There have a service called Android Foreground Service is the long running foreground service that give a running notification UI to the user. Besides that, it can let user perform some action on the notification and it not bind to any component in the android. For a good example, we can use foreground service to play music and show it in the notification while user switch to another app. In this tutorial, I will give you all the steps to create the foreground service in android. It just take around 10 minutes to complete the foreground service.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “ForegroundServiceExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit activity_main.xml layout
Go to your activity_main.xml insert the below code. I add two button inside the linear layout.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="16dp" > <Button android:id="@+id/button1" android:layout_width="0dp" android:layout_weight="1" android:layout_height="wrap_content" android:text="Start Foreground Service" /> <Button android:id="@+id/button2" android:layout_width="0dp" android:layout_weight="1" android:layout_height="wrap_content" android:text="Stop Foreground Service" /> </LinearLayout>
Create a custom notification layout
Right click layout folder and create a new xml file and name it as notification. It is to customize the notification that you want.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:id="@+id/notification" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_toLeftOf="@+id/llPlayControls" android:padding="10dp" android:orientation="vertical" > <TextView android:id="@+id/notification_text_title" android:layout_width="match_parent" android:layout_height="wrap_content" android:singleLine="true" android:text="Foreground Service" android:textStyle="bold" android:ellipsize="end" android:lines="1" /> </LinearLayout> <LinearLayout android:id="@+id/llPlayControls" android:layout_alignParentRight="true" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:orientation="horizontal"> <ImageView android:id="@+id/notification_button_close" android:layout_width="48dp" android:layout_height="48dp" android:src="@android:drawable/ic_menu_close_clear_cancel" /> </LinearLayout> </RelativeLayout>
Create a Service
Right click you package name and create a new service activity name it as ForegroundService.
Edit ForegroundService.java class
In your foregroundservice.java add the notification and broadcast receiver in your service.
package com.example.questdot.foregroundserviceexample; import android.app.Notification; import android.app.PendingIntent; import android.app.Service; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.graphics.Bitmap; import android.graphics.BitmapFactory; import android.os.IBinder; import android.support.v4.app.NotificationCompat; import android.util.Log; import android.widget.RemoteViews; import android.widget.Toast; public class ForegroundService extends Service { @Override public void onCreate() { super.onCreate(); } @Override public int onStartCommand(Intent intent, int flags, int startId) { Toast.makeText(this,"Start Service",Toast.LENGTH_SHORT).show(); Intent notificationIntent = new Intent(this, MainActivity.class); notificationIntent.setAction(MainActivity.MAIN_ACTION); notificationIntent.setFlags(Intent.FLAG_ACTIVITY_NEW_TASK | Intent.FLAG_ACTIVITY_CLEAR_TASK); PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, notificationIntent, 0); RemoteViews notificationView = new RemoteViews(this.getPackageName(),R.layout.notification); Intent buttonCloseIntent = new Intent(this, NotificationCloseButtonHandler.class); buttonCloseIntent.putExtra("action", "close"); PendingIntent buttonClosePendingIntent = pendingIntent.getBroadcast(this, 0, buttonCloseIntent, 0); notificationView.setOnClickPendingIntent(R.id.notification_button_close, buttonClosePendingIntent); Bitmap icon = BitmapFactory.decodeResource(getResources(), R.mipmap.ic_launcher); Notification notification = new NotificationCompat.Builder(this) .setContentTitle("Test") .setTicker("Test") .setContentText("Test") .setSmallIcon(R.mipmap.ic_launcher) .setLargeIcon( Bitmap.createScaledBitmap(icon, 128, 128, false)) .setContent(notificationView) .setOngoing(true).build(); startForeground(101, notification); return START_STICKY; } @Override public void onDestroy() { super.onDestroy(); stopForeground(true); } @Override public IBinder onBind(Intent intent) { // Used only in case of bound services. return null; } public static class NotificationCloseButtonHandler extends BroadcastReceiver { @Override public void onReceive(Context context, Intent intent) { Toast.makeText(context,"Close Clicked",Toast.LENGTH_SHORT).show(); } } }
Edit MainActivity.java class
Go to your MainActivity.java and copy paste the following code.
package com.example.questdot.foregroundserviceexample; import android.content.Intent; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; public class MainActivity extends AppCompatActivity { public static String MAIN_ACTION = "com.nkdroid.alertdialog.action.main"; private Button startButton,stopButton; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); startButton= (Button)findViewById(R.id.button1); stopButton = (Button)findViewById(R.id.button2); startButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent startIntent = new Intent(MainActivity.this, ForegroundService.class); startService(startIntent); } }); stopButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { Intent stopIntent = new Intent(MainActivity.this, ForegroundService.class); stopService(stopIntent); } }); } }
Run your Project
Now, you can start your project in your android device and test the foreground service.
Didn’t you miss the part about AndroidManifest.xml?
It is really a nice and useful piece of info.
I am glad thwt you shared this useful information wwith us.
Please stay us informed like this. Thank you for sharing.
Really helpful. Thankyou so much