Android Realm Database Replacement for SQLite and ORMs
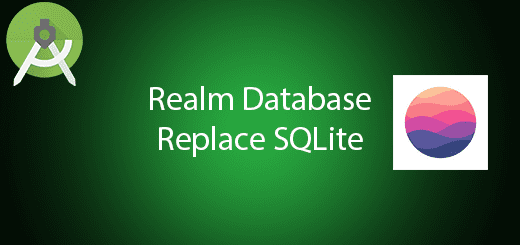
Android provide SQLite database for local database in the android application. SQLite is hard to setting up because it require a lot of implementation and sql command. Due to performance of SQLite, it is much slower than Realm and other ORMs database. So, I would like to suggest the best local database for you which is Realm database. Realm database is easy to setting up in just a few minutes instead of hours if you are a experts. It is fast in performance because it have its own C++ core and aims to provide a better database to mobile application. In this tutorial, I will teach you how to use android realm database in the mobile application to perform create, update , delete and retrieve features.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “RealmExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add a Dependecy
Open build.gradle (Project : RealmExample) and add realm dependency from the below and put inside the dependencies section.
classpath "io.realm:realm-gradle-plugin:1.2.0"
Add a plugin
After that, open build.gradle (Module :app) and apply a plugin after the dependencies section.
apply plugin: 'realm-android'
Create a class to extends Application
Right click your package name and create a new class name to CustomApplication. This class is to add the realm configuration in your application.
import android.app.Application; import io.realm.Realm; import io.realm.RealmConfiguration; public class CustomApplication extends Application { @Override public void onCreate() { super.onCreate(); RealmConfiguration realmConfiguration = new RealmConfiguration.Builder(this) .name(Realm.DEFAULT_REALM_NAME) .schemaVersion(0) .deleteRealmIfMigrationNeeded() .build(); Realm.setDefaultConfiguration(realmConfiguration); } }
Edit AndroidManifest.xml
Add name in the application section. I add android:name”.CustomApplication” in the application level as show below source code. It is to define the startup application class.
<application android:name=".CustomApplication" android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:supportsRtl="true" android:theme="@style/AppTheme">
Create a new class
Besides, create a new class for perform the create, retrieve , update and delete feature for realm database to use it later. Therefore, copy and paste the java code in the below section.
import io.realm.Realm; import io.realm.RealmResults; public class RealmController { private static RealmController instance =new RealmController(); private final Realm realm; public RealmController() { realm = Realm.getDefaultInstance(); } public static RealmController getInstance() { return instance; } public Realm getRealm() { return realm; } //Refresh the realm istance public void refresh() { realm.setAutoRefresh(true); } //clear all objects from User.class public void clearAll() { realm.beginTransaction(); realm.delete(User.class); realm.commitTransaction(); } //find all objects in the User.class public RealmResults<User> getUsers() { return realm.where(User.class).findAll(); } //query a single item with the given id public User getUser(int id) { return realm.where(User.class).equalTo("user_id", id).findFirst(); } //check if User.class is empty public boolean hasUser() { return !realm.isEmpty(); } //query example public RealmResults<User> queriedUser() { return realm.where(User.class) .contains("first_name", "A") .or() .contains("country", "Indonesia") .findAll(); } public void createUser(User userReq){ User user = new User(); user.setUser_id((int) (System.currentTimeMillis())); user.setFirst_name(userReq.getFirst_name()); user.setLast_name(userReq.getLast_name()); user.setCountry(userReq.getCountry()); realm.beginTransaction(); realm.copyToRealm(user); realm.commitTransaction(); } public void updateUser(User userReq){ User user = getUser(userReq.getUser_id()); realm.beginTransaction(); user.setFirst_name(userReq.getFirst_name()); user.setLast_name(userReq.getLast_name()); user.setCountry(userReq.getCountry()); realm.commitTransaction(); } public void deleteUserByPosition(int position){ RealmResults<User> results = realm.where(User.class).findAll(); // All changes to data must happen in a transaction realm.beginTransaction(); // remove single match results.deleteFromRealm(position); realm.commitTransaction(); } }
Create a class model
Create a new model class and name it as “User” and extends the class to RealmObject as the following source code below.
import io.realm.RealmObject; import io.realm.annotations.PrimaryKey; public class User extends RealmObject { @PrimaryKey private int user_id; private String first_name; private String last_name; private String country; public User() { } public User(int user_id, String first_name, String last_name, String country) { this.country = country; this.first_name = first_name; this.last_name = last_name; this.user_id = user_id; } public User(String first_name, String last_name, String country ) { this.country = country; this.last_name = last_name; this.first_name = first_name; } public String getCountry() { return country; } public void setCountry(String country) { this.country = country; } public String getFirst_name() { return first_name; } public void setFirst_name(String first_name) { this.first_name = first_name; } public String getLast_name() { return last_name; } public void setLast_name(String last_name) { this.last_name = last_name; } public int getUser_id() { return user_id; } public void setUser_id(int user_id) { this.user_id = user_id; } }
Create a new class for Recyclerview Adapter
After that, add a new class and name this class to userAdapter, this adapter is to show each row of content. The following source code is the example on how to write the adapter.
public class UserAdapter extends RecyclerView.Adapter<UserAdapter.UserViewHolder> { private List<User> userList; Context context; public class UserViewHolder extends RecyclerView.ViewHolder { TextView firstName; TextView lastName; TextView country; public UserViewHolder(View view) { super(view); firstName = (TextView) view.findViewById(R.id.first_name); lastName = (TextView)view.findViewById(R.id.last_name); country = (TextView)view.findViewById(R.id.country); } } public UserAdapter(List<User> userList, Context context) { this.userList = userList; this.context = context; } @Override public UserViewHolder onCreateViewHolder(ViewGroup parent, int viewType) { View itemView = LayoutInflater.from(parent.getContext()) .inflate(R.layout.user_list_row, parent, false); return new UserViewHolder(itemView); } @Override public void onBindViewHolder(UserViewHolder holder, final int position) { final User user = userList.get(position); holder.firstName.setText(user.getFirst_name()); holder.country.setText(user.getCountry()); holder.lastName.setText(user.getLast_name()); holder.itemView.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { Intent intent = new Intent(context, UserDetailActivity.class); intent.putExtra("user_id", user.getUser_id()); intent.putExtra("position", position); context.startActivity(intent); } }); } @Override public int getItemCount() { return userList.size(); } }
Add two new Empty Activity for Add User, Edit User and Delete User.
Create two empty activity in your project. The first activity name is AddUserActivity while another activity name is UserDetailActivity is for Edit and delete the selected user.
Add a new xml layout and edit it
Create a new xml in the res>layout folder name it to user_list_row.xml then copy and paste the xml source code in the below. It the layout of recyclerview item.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="10dp"> <TextView android:id="@+id/first_name" android:textSize="16dp" android:textStyle="bold" android:layout_width="match_parent" android:layout_height="wrap_content" /> <TextView android:id="@+id/last_name" android:layout_width="match_parent" android:layout_height="wrap_content" /> <TextView android:id="@+id/country" android:layout_width="match_parent" android:layout_height="wrap_content" /> </LinearLayout>
Edit activity_add_user.xml layout
Open activity_add_user.xml and follow the source code in the following section. It is the layout of add user activity.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:orientation="vertical" tools:context=".UserDetailActivity"> <EditText android:id="@+id/et_firstname" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/activity_vertical_margin" android:hint="First Name"/> <EditText android:id="@+id/et_lastname" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/activity_vertical_margin" android:hint="Last Name"/> <EditText android:id="@+id/et_country" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/activity_vertical_margin" android:hint="Country"/> <Button android:id="@+id/btn_save" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Add"/> </LinearLayout>
Edit activity_user_detail.xml layout
Open activity_user_detail.xml and modify to the following code.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:orientation="vertical" tools:context=".UserDetailActivity"> <EditText android:id="@+id/et_firstname" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/activity_vertical_margin" android:hint="First Name"/> <EditText android:id="@+id/et_lastname" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/activity_vertical_margin" android:hint="Last Name"/> <EditText android:id="@+id/et_country" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/activity_vertical_margin" android:hint="Country"/> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="horizontal"> <Button android:id="@+id/btn_save" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Edit"/> <Button android:id="@+id/btn_delete" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Delete"/> </LinearLayout> </LinearLayout>
Edit activity_main.xml layout
Edit your activity_main.xml to the source code that i provide in the bottom.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:orientation="vertical" > <android.support.v7.widget.RecyclerView android:id="@+id/recycler_view" android:layout_width="match_parent" android:layout_height="match_parent" android:layout_weight="1" android:scrollbars="vertical" /> <Button android:id="@+id/btn_add" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/activity_vertical_margin" android:text="Add New Record"/> </LinearLayout>
Edit AddUser.java class
This class is to perform add user in the realm database.
import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; public class AddUserActivity extends AppCompatActivity implements View.OnClickListener{ EditText etFirstname; EditText etLastname; EditText etCountry; Button btnSave; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_add_user); etFirstname = (EditText)findViewById(R.id.et_firstname); etLastname = (EditText)findViewById(R.id.et_lastname); etCountry = (EditText)findViewById(R.id.et_country); btnSave = (Button)findViewById(R.id.btn_save); btnSave.setOnClickListener(this); } @Override public void onClick(View v) { switch (v.getId()){ case R.id.btn_save: if (etFirstname.getText().toString().trim().equals("") || etLastname.getText().toString().trim().equals("") || etCountry.getText().toString().trim().equals("")) { Toast.makeText(this, "Entry not saved, missing some fields", Toast.LENGTH_SHORT).show(); } else { RealmController.getInstance().createUser(new User(etFirstname.getText().toString(), etLastname.getText().toString(), etCountry.getText().toString())); finish(); } break; } } }
Edit UserDetailsActivity.java class
After that, edit your userdetailsactivity.java to perform delete and update existing user feature in the realm database.
package com.questdot.realmexample; import android.content.Intent; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; public class UserDetailActivity extends AppCompatActivity implements View.OnClickListener{ EditText etFirstname; EditText etLastname; EditText etCountry; Button btnSave,btnDelete; private int id; private int position; private User user; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_user_detail); etFirstname = (EditText)findViewById(R.id.et_firstname); etLastname = (EditText)findViewById(R.id.et_lastname); etCountry = (EditText)findViewById(R.id.et_country); btnSave = (Button)findViewById(R.id.btn_save); btnDelete = (Button)findViewById(R.id.btn_delete); btnSave.setOnClickListener(this); btnDelete.setOnClickListener(this); Intent intent = getIntent(); id = intent.getIntExtra("user_id", 0); position = intent.getIntExtra("position", 0); user = RealmController.getInstance().getUser(id); etFirstname.setText(user.getFirst_name()); etLastname.setText(user.getLast_name()); etCountry.setText(user.getCountry()); } @Override public void onClick(View v) { switch (v.getId()) { case R.id.btn_delete: RealmController.getInstance().deleteUserByPosition(position); finish(); break; case R.id.btn_save: if (etFirstname.getText().toString().trim().equals("") || etLastname.getText().toString().trim().equals("") || etCountry.getText().toString().trim().equals("")) { Toast.makeText(this, "Entry not saved, missing some fields", Toast.LENGTH_SHORT).show(); } else { RealmController.getInstance().updateUser(new User(id, etFirstname.getText().toString(), etLastname.getText().toString(), etCountry.getText().toString())); finish(); } break; } } }
Edit MainActivity.java class
Open your mainactivity.java class and copy and paste the following source code. This class is to show the user from the realm database.
package com.questdot.realmexample; import android.content.Intent; import android.support.design.widget.FloatingActionButton; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.support.v7.widget.DefaultItemAnimator; import android.support.v7.widget.LinearLayoutManager; import android.support.v7.widget.RecyclerView; import android.support.v7.widget.Toolbar; import android.view.View; import android.widget.Button; import android.widget.EditText; import java.util.ArrayList; import io.realm.Realm; import io.realm.RealmConfiguration; public class MainActivity extends AppCompatActivity implements View.OnClickListener { RecyclerView recyclerView; Button btnAdd; // private Realm realm; private UserAdapter adapter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); recyclerView = (RecyclerView)findViewById(R.id.recycler_view); btnAdd = (Button)findViewById(R.id.btn_add); btnAdd.setOnClickListener(this); //get realm instance // this.realm = RealmController.with(this).getRealm(); } private void setRealmData() { // refresh the realm instance RealmController.getInstance().refresh(); adapter = new UserAdapter(RealmController.getInstance().getUsers(), this); RecyclerView.LayoutManager mLayoutManager = new LinearLayoutManager(getApplicationContext()); recyclerView.setLayoutManager(mLayoutManager); recyclerView.setItemAnimator(new DefaultItemAnimator()); recyclerView.setAdapter(adapter); } @Override protected void onResume() { super.onResume(); setRealmData(); } @Override public void onClick(View v) { switch (v.getId()) { case R.id.btn_add: Intent intent = new Intent(this, AddUserActivity.class); startActivity(intent); } } }
Run your project
Finally, you done the create, update, retrieve and delete feature in the realm database. So you can run the project and try it in the android application.