Android Fingerprint Authentication Tutorial
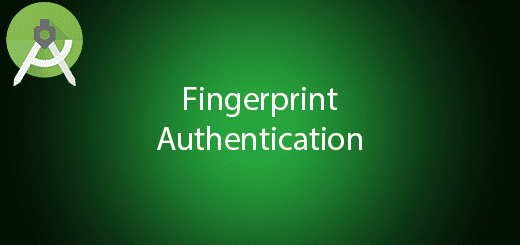
Android 6.0 Marshmallow was released the Fingerprint authentication APIs for Android devices. To use the fingerprint touch sensor, the smartphone must have Android 6.0 and above to use this feature. Fingerprint authentication enhances the security of the application and its does not require to enter the password which fast, convenient and reliable. It is suitable for making a transaction and unlocks some sensitive data in the mobile application. In this tutorial, I will tell you how to use Android fingerprint authentication in your project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “FingerprintExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add a new permission
Open your AndroidManifest.xml, add fingerprint permission so that you allow to perform fingerprint.
<uses-permission android:name="android.permission.USE_FINGERPRINT" />
Create a new class
Right click your project name and create a new class “FingerprintHandler”, this class extends the fingerprint manage callback.
import android.hardware.fingerprint.FingerprintManager; import android.os.Build; import android.os.CancellationSignal; import android.support.annotation.RequiresApi; import android.widget.TextView; @RequiresApi(api = Build.VERSION_CODES.M) public class FingerprintHandler extends FingerprintManager.AuthenticationCallback { private TextView tv; public FingerprintHandler(TextView tv) { this.tv = tv; } public void doAuth(FingerprintManager manager, FingerprintManager.CryptoObject obj) { CancellationSignal signal = new CancellationSignal(); try { manager.authenticate(obj, signal, 0, this, null); } catch(SecurityException sce) {} } @Override public void onAuthenticationError(int errorCode, CharSequence errString) { super.onAuthenticationError(errorCode, errString); tv.setText("Auth Error"); } @Override public void onAuthenticationHelp(int helpCode, CharSequence helpString) { super.onAuthenticationHelp(helpCode, helpString); } @Override public void onAuthenticationSucceeded(FingerprintManager.AuthenticationResult result) { super.onAuthenticationSucceeded(result); tv.setText("Auth Success"); } @Override public void onAuthenticationFailed() { super.onAuthenticationFailed(); } }
Edit activity_main.xml layout
Go to activity_main.xml layout and add one textview, a button in this layout. The textview is to display the status of the fingerprint and button is to perform the fingerprint feature.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:id="@+id/fingerStatus"/> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/authBtn" android:layout_alignParentBottom="true" android:layout_centerHorizontal="true" android:text="Start Scan"/> </RelativeLayout>
Edit MainActivity.java class
Open the MainActivity.java class and it will generate the key, perform encryption and verify your fingerprint. Follow the source code below.
import android.app.KeyguardManager; import android.hardware.fingerprint.FingerprintManager; import android.os.Build; import android.security.keystore.KeyGenParameterSpec; import android.security.keystore.KeyGenParameterSpec.Builder; import android.security.keystore.KeyProperties; import android.support.annotation.RequiresApi; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; import java.io.IOException; import java.security.InvalidAlgorithmParameterException; import java.security.InvalidKeyException; import java.security.KeyStore; import java.security.KeyStoreException; import java.security.NoSuchAlgorithmException; import java.security.NoSuchProviderException; import java.security.UnrecoverableKeyException; import java.security.cert.CertificateException; import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.NoSuchPaddingException; import javax.crypto.SecretKey; public class MainActivity extends AppCompatActivity { private TextView message; private static final String KEY_NAME = "myKey"; private KeyStore keyStore; private KeyGenerator keyGenerator; private FingerprintManager.CryptoObject cryptoObject; private FingerprintHandler fph; private FingerprintManager fingerprintManager; @RequiresApi(api = Build.VERSION_CODES.M) @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); message = (TextView) findViewById(R.id.fingerStatus); Button btn = (Button) findViewById(R.id.authBtn); fph = new FingerprintHandler(message); if (!checkFinger()) { btn.setEnabled(false); } else { // We are ready to set up the cipher and the key try { generateKey(); Cipher cipher = generateCipher(); cryptoObject = new FingerprintManager.CryptoObject(cipher); } catch(FingerprintException fpe) { // Handle exception btn.setEnabled(false); } } btn.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View view) { message.setText("Click the button to start scan your fingerprint"); fph.doAuth(fingerprintManager, cryptoObject); } }); } @RequiresApi(api = Build.VERSION_CODES.M) private boolean checkFinger() { // Keyguard Manager KeyguardManager keyguardManager = (KeyguardManager) getSystemService(KEYGUARD_SERVICE); // Fingerprint Manager fingerprintManager = (FingerprintManager) getSystemService(FINGERPRINT_SERVICE); try { // Check if the fingerprint sensor is present if (!fingerprintManager.isHardwareDetected()) { message.setText("Fingerprint authentication not supported"); return false; } if (!fingerprintManager.hasEnrolledFingerprints()) { message.setText("No fingerprint configured."); return false; } if (!keyguardManager.isKeyguardSecure()) { message.setText("Secure lock screen not enabled"); return false; } } catch(SecurityException se) { se.printStackTrace(); } return true; } @RequiresApi(api = Build.VERSION_CODES.M) private void generateKey() throws FingerprintException { try { // Get the reference to the key store keyStore = KeyStore.getInstance("AndroidKeyStore"); // Key generator to generate the key keyGenerator = KeyGenerator.getInstance(KeyProperties.KEY_ALGORITHM_AES, "AndroidKeyStore"); keyStore.load(null); keyGenerator.init( new KeyGenParameterSpec.Builder(KEY_NAME, KeyProperties.PURPOSE_ENCRYPT | KeyProperties.PURPOSE_DECRYPT) .setBlockModes(KeyProperties.BLOCK_MODE_CBC) .setUserAuthenticationRequired(true) .setEncryptionPaddings( KeyProperties.ENCRYPTION_PADDING_PKCS7) .build()); keyGenerator.generateKey(); } catch(KeyStoreException | NoSuchAlgorithmException | NoSuchProviderException | InvalidAlgorithmParameterException | CertificateException | IOException exc) { exc.printStackTrace(); throw new FingerprintException(exc); } } private Cipher generateCipher() throws FingerprintException { try { Cipher cipher = Cipher.getInstance(KeyProperties.KEY_ALGORITHM_AES + "/" + KeyProperties.BLOCK_MODE_CBC + "/" + KeyProperties.ENCRYPTION_PADDING_PKCS7); SecretKey key = (SecretKey) keyStore.getKey(KEY_NAME, null); cipher.init(Cipher.ENCRYPT_MODE, key); return cipher; } catch (NoSuchAlgorithmException | NoSuchPaddingException | InvalidKeyException | UnrecoverableKeyException | KeyStoreException exc) { exc.printStackTrace(); throw new FingerprintException(exc); } } private class FingerprintException extends Exception { public FingerprintException(Exception e) { super(e); } } }
Run Your Project
In conclusion, now you can run this project in your android device to test the fingerprint authentication.
Hello, this publication is very good.
How can I do when the fingerprint is validated and that event is taken over by a web service?
My mane in Agustin from Argentina, Patagonia.
Thanks.