Android ContentProvider Tutorial
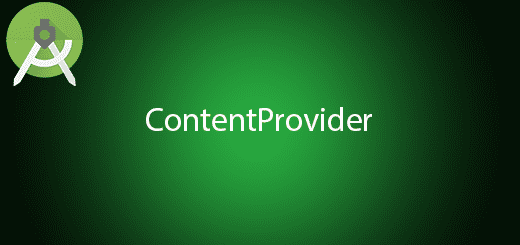
ContentProvider is a component that provides data control from one application to another application. It can choose many different way to store the data. The data can be store in 3 way which is files, SQLite database or over the internet (JSON). The content provider recommended use when share data among multiple application. For example, android operating system provide the contacts data which allow other application to access it by doing some implementation. If you don want to share data, you will be better use SQLite database in android. In this tutorial, I will give you some guideline on how to create a content provider in android which is just a easy method.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “ContentProviderExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Project” and Click Next button
5. Lastly, press finish button.
Create a new ContentProvider
Create a new content provider class. Right click package name > New > Other > Content Provider. It will show a authority URI. After create a new content provider, the Android Manifest file will automatically add the provider.
<provider android:name=".MyContentProvider" android:authorities="com.example.questdot" android:enabled="true" android:exported="true"></provider>
Edit MyContentProvider.java class
In your content provider class, add the following code in your activity.
package com.example.questdot.contentproviderexample; import android.content.ContentProvider; import android.content.ContentValues; import android.content.Context; import android.database.Cursor; import android.database.sqlite.SQLiteDatabase; import android.net.Uri; public class MyContentProvider extends ContentProvider { public MyContentProvider() { } public static final Uri URI = Uri.parse("content://com.example.questdot"); SQLiteDatabase database; @Override public int delete(Uri arg0, String arg1, String[] arg2) { // TODO Auto-generated method stub return 0; } @Override public String getType(Uri arg0) { // TODO Auto-generated method stub return null; } @Override public Uri insert(Uri arg0, ContentValues arg1) { database.insert("tab", "_id", arg1); // database.close(); return null; } @Override public boolean onCreate() { database = getContext().openOrCreateDatabase("dbsample", Context.MODE_PRIVATE, null); database.execSQL("create table tab(_id INTEGER PRIMARY KEY AUTOINCREMENT, name TEXT NOT NULL)"); return true; } @Override public Cursor query(Uri arg0, String[] arg1, String arg2, String[] arg3, String arg4) { Cursor cursor = database.query("tab", null, null, null, null, null, null); return cursor; } @Override public int update(Uri arg0, ContentValues arg1, String arg2, String[] arg3) { // TODO Auto-generated method stub return 0; } }
Edit activity.xml layout
Go to your activity.xml file and add a button in your layout.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/container" android:layout_width="match_parent" android:layout_height="match_parent" tools:ignore="MergeRootFrame" > <Button android:id="@+id/button1" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Write" android:layout_centerVertical="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> </RelativeLayout> /
Edit MainActivity.java class
In your MainActivity.java, add the following code in your activity.
package com.example.questdot.contentproviderexample; import android.app.Activity; import android.app.ActionBar; import android.app.Fragment; import android.content.ContentValues; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.LayoutInflater; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.ViewGroup; import android.os.Build; import android.widget.Toast; public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); findViewById(R.id.button1).setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { write(); Toast.makeText(getApplicationContext(), "Successfully write into content provider", Toast.LENGTH_SHORT).show(); } }); } public void write() { ContentValues values; values = new ContentValues(); values.put("name", "Java"); getContentResolver().insert(MyContentProvider.URI, values); } } /
Create a new module
Add a new module in your project and name it as readcontentprovider.
Edit activity_main.xml
In the module “readcontentprovider”, go to activity_main.xml and add a textView in the layout.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/textView" /> </RelativeLayout>
Edit MainActivity.java class
After that, go to your MainActivity.java and add the following code.
package com.example.questdot.readcontentprovider; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.app.Activity; import android.app.ActionBar; import android.app.Fragment; import android.database.Cursor; import android.net.Uri; import android.os.Bundle; import android.view.LayoutInflater; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.ViewGroup; import android.widget.TextView; import android.widget.Toast; import android.os.Build; import org.w3c.dom.Text; public class MainActivity extends AppCompatActivity { Uri URI = Uri.parse("content://com.example.questdot"); TextView textView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); textView =(TextView)findViewById(R.id.textView); Cursor cursor = getContentResolver().query(URI, null, null, null, null); cursor.moveToFirst(); for (int i = 0; i < cursor.getCount(); i++) { String value = cursor.getString(cursor.getColumnIndex("name")); if(textView.getText().toString().isEmpty()){ textView.setText(value); } else{ textView.append(value); } cursor.moveToNext(); } } } /
Run your Project
Ok…. its finish. Now you can test your project now…. first you need to start the module “app” first to write data in your content provider. After that, you can start “readcontentprovider” module to read the data provided by the module “app”.
1 Response
[…] Add a new class for contentprovider name as SyncProvider, this is a dummy contentprovider without any database. This is to easy for demonstrate the feature. You can add your SQLite to the ContentProvider in the following link. https://questdot.com/android-contentprovider/ […]