Android Detect GPS Location Tutorial
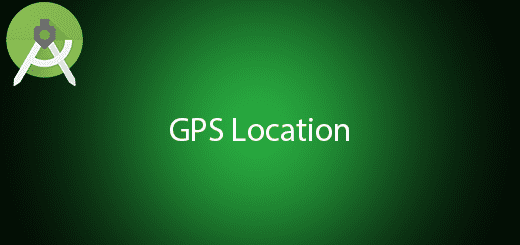
Every android device has GPS feature to detect the device location. Using Location services can automatically get the current user location without his or her to enter the location in the mobile. With the location data, your app can predict a user’s potential actions, recommend actions, or perform actions in the background without user interaction. GPS feature able to get Latitude and Longitude of the device to determine where exactly the device locate. It is worth pointing out that Location can contain much more than just latitude and longitude values. In this tutorial, I will tell you how to use android detect GPS location in your project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “GPSLocationExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit AndroidManifest.xml
Add 2 permissions in your manifest file to access your GPS location.
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> <uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" />
Create a new class
Right click your package name and create a class “GPSTracker” to manage location. Edit the file to the source code below.
public class GPSTracker extends Service implements LocationListener { private final Activity mContext; // flag for GPS status boolean isGPSEnabled = false; /* // flag for network status boolean isNetworkEnabled = false;*/ // flag for GPS status boolean canGetLocation = false; Location location; // location double latitude; // latitude double longitude; // longitude // The minimum distance to change Updates in meters private static final long MIN_DISTANCE_CHANGE_FOR_UPDATES = 10; // 10 meters // The minimum time between updates in milliseconds private static final long MIN_TIME_BW_UPDATES = 1000 * 60 * 1; // 1 minute // Declaring a Location Manager protected LocationManager locationManager; public GPSTracker(Activity context) { this.mContext = context; getLocation(); } public Location getLocation() { if(checkLocationPermission()) { locationManager = (LocationManager) mContext .getSystemService(LOCATION_SERVICE); // getting GPS status isGPSEnabled = locationManager .isProviderEnabled(LocationManager.GPS_PROVIDER); /* // getting network status isNetworkEnabled = locationManager .isProviderEnabled(LocationManager.NETWORK_PROVIDER);*/ if (!isGPSEnabled /*&& !isNetworkEnabled*/) { showSettingsAlert(); } else { this.canGetLocation = true; // First get location from Network Provider /* if (isNetworkEnabled) { locationManager.requestLocationUpdates( LocationManager.NETWORK_PROVIDER, MIN_TIME_BW_UPDATES, MIN_DISTANCE_CHANGE_FOR_UPDATES, this); if (locationManager != null) { location = locationManager .getLastKnownLocation(LocationManager.NETWORK_PROVIDER); if (location != null) { latitude = location.getLatitude(); longitude = location.getLongitude(); } } }*/ // if GPS Enabled get lat/long using GPS Services if (isGPSEnabled) { if (location == null) { locationManager.requestLocationUpdates( LocationManager.GPS_PROVIDER, MIN_TIME_BW_UPDATES, MIN_DISTANCE_CHANGE_FOR_UPDATES, this); if (locationManager != null) { location = locationManager .getLastKnownLocation(LocationManager.GPS_PROVIDER); if (location != null) { latitude = location.getLatitude(); longitude = location.getLongitude(); } } } } } }else{ ActivityCompat.requestPermissions(mContext,new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 1); } return location; } public void stopUsingGPS(){ if(locationManager != null){ locationManager.removeUpdates(GPSTracker.this); } } public double getLatitude(){ if(location != null){ latitude = location.getLatitude(); } // return latitude return latitude; } public double getLongitude(){ if(location != null){ longitude = location.getLongitude(); } return longitude; } /** * Function to check GPS/wifi enabled * @return boolean * */ public boolean canGetLocation() { return this.canGetLocation; } public void showSettingsAlert(){ AlertDialog.Builder alertDialog = new AlertDialog.Builder(mContext); // Setting Dialog Title alertDialog.setTitle("GPS is settings"); // Setting Dialog Message alertDialog.setMessage("GPS is not enabled. Do you want to go to settings menu?"); // On pressing Settings button alertDialog.setPositiveButton("Settings", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog,int which) { Intent intent = new Intent(Settings.ACTION_LOCATION_SOURCE_SETTINGS); mContext.startActivity(intent); } }); // on pressing cancel button alertDialog.setNegativeButton("Cancel", new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { dialog.cancel(); } }); // Showing Alert Message alertDialog.show(); } public boolean checkLocationPermission() { String permission = "android.permission.ACCESS_FINE_LOCATION"; int res = mContext.checkCallingOrSelfPermission(permission); return (res == PackageManager.PERMISSION_GRANTED); } @Override public void onLocationChanged(Location location) { } @Override public void onProviderDisabled(String provider) { } @Override public void onProviderEnabled(String provider) { } @Override public void onStatusChanged(String provider, int status, Bundle extras) { } @Override public IBinder onBind(Intent arg0) { return null; } }
Edit activity_main.xml layout
Add a button in the layout so that you can get the location by clicking the button.
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.questdot.gpslocationexample.MainActivity"> <Button android:id="@+id/button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Get Location" tools:layout_editor_absoluteX="131dp" tools:layout_editor_absoluteY="231dp" /> </android.support.constraint.ConstraintLayout>
Edit MainActivity.java class
Modify the MainActivity class to the following sample source code. By clicking the button, the app will get the location of the device.
public class MainActivity extends AppCompatActivity { Button button; GPSTracker gps; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); ActivityCompat.requestPermissions(this,new String[]{Manifest.permission.ACCESS_FINE_LOCATION}, 1); button = (Button) findViewById(R.id.button); // show location button click event button.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View arg0) { gps = new GPSTracker(MainActivity.this); // check if GPS enabled if(gps.canGetLocation()){ double latitude = gps.getLatitude(); double longitude = gps.getLongitude(); Toast.makeText(getApplicationContext(), "Location - \nLat: " + latitude + "\nLong: " + longitude, Toast.LENGTH_LONG).show(); } } }); } public void onRequestPermissionsResult(int requestCode, String permissions[], int[] grantResults) { switch (requestCode) { case 1: { // If request is cancelled, the result arrays are empty. if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) { Toast.makeText(getApplicationContext(),"granted",Toast.LENGTH_LONG).show(); } else { Toast.makeText(getApplicationContext(),"denied",Toast.LENGTH_LONG).show(); // permission denied, boo! Disable the // functionality that depends on this permission. } return; } // other 'case' lines to check for other // permissions this app might request } } }
Run Your Project
Finally, you can run it in your android device and make sure your GPS is open so it can get your device latitude and longitude.