Android Date Picker Dialog Tutorial
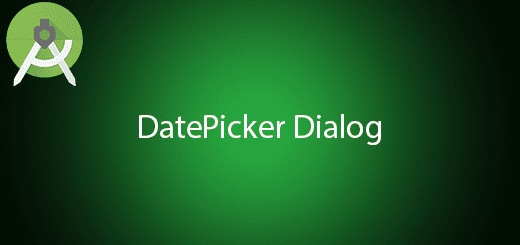
In material design, android provide the material date picker dialog to pick date in the application. It is very useful when come to select a date, its accurate and eliminate the human mistake if they enter by text. Date Picker dialog let the user to pick the date from the calendar with the material design interface. In this tutorial, I will tell you on how to use android date picker dialog to pick date in your android project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “DatePickerExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Create a new Class
Right click your project name and create a new class “DatePickerFragment”, after that, copy the source code in the bottom in your class.
public class DatePickerFragment extends DialogFragment { private onDateClickListener onDateClickListener; @Override public Dialog onCreateDialog(Bundle savedInstanceState) { // Use the current date as the default date in the picker final Calendar c = Calendar.getInstance(); int year = c.get(Calendar.YEAR); int month = c.get(Calendar.MONTH); int day = c.get(Calendar.DAY_OF_MONTH); // Create a new instance of DatePickerDialog and return it return new DatePickerDialog(getActivity(), new DatePickerDialog.OnDateSetListener() { @Override public void onDateSet(DatePicker datePicker, int i, int i1, int i2) { onDateClickListener.onDateSet(datePicker,i, i1, i2); } }, year, month, day); } public void setOnDateClickListener(onDateClickListener onDateClickListener){ if(onDateClickListener != null){ this.onDateClickListener = onDateClickListener; } } } interface onDateClickListener { void onDateSet(DatePicker datePicker, int i, int i1, int i2); }
Edit activity_main.xml
Open the activity_main.xml layout and modify your layout to the following.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity"> <Button android:id="@+id/btn1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Pick Date" android:onClick="showDatePickerDialog" android:layout_centerVertical="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <TextView android:id="@+id/txtDate" android:text="text" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_above="@+id/btn1" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> </RelativeLayout>
Edit MainActivity.java class
Open your mainactivity class and this activity will show the date picker dialog and you can select any date and display in the activity.
public class MainActivity extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); } public void showDatePickerDialog(View v) { DatePickerFragment newFragment = new DatePickerFragment(); newFragment.show(getSupportFragmentManager(), "datePicker"); newFragment.setOnDateClickListener(new onDateClickListener() { @Override public void onDateSet(DatePicker datePicker, int i, int i1, int i2) { TextView tv1= (TextView) findViewById(R.id.txtDate); tv1.setText(datePicker.getYear()+"/"+datePicker.getMonth()+"/"+datePicker.getDayOfMonth()); } }); } }
Run Your Project
Finally, you are successfully implement the date picker in your android project, you can now open and run it in your device.