Android RatingBar Widget Tutorial
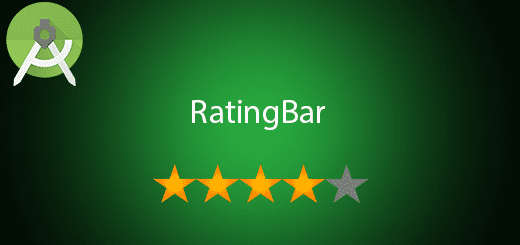
Android have its native widget ratingbar to perform rating in the mobile application. It is useful for eCommerce application or other application that provide services to the user. RatingBar is very easy to use and perform in the android application. You just need to add the widget in the xml file to display it. In this tutorial, I will teach you some basic on how to use android ratingbar in the mobile application.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “RatingBarExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit main_activity.xml layout
This layout will be display the ratingbar ,text and 3 buttons for save rating, add rate and minus rate. You can follow the example below to display it.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MainActivity" android:id="@+id/abc"> <RatingBar android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/ratingBar" android:numStars="5" android:stepSize="1" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:gravity="center" android:text="Rating" android:id="@+id/textView" android:layout_alignParentTop="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" /> <Button android:text="-1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/ratingBar" android:layout_alignLeft="@+id/ratingBar" android:layout_alignStart="@+id/ratingBar" android:layout_marginTop="51dp" android:id="@+id/button" /> <Button android:text="+1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignBottom="@+id/button" android:layout_alignRight="@+id/ratingBar" android:layout_alignEnd="@+id/ratingBar" android:id="@+id/button2" /> <Button android:text="Save" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_above="@+id/ratingBar" android:layout_centerHorizontal="true" android:layout_marginBottom="65dp" android:id="@+id/btnSave" /> </RelativeLayout>
Edit MainActivity.java class
In this class, will use the sharedpreference to save the value of ratingbar and you can either click the ratingbar to change the rating or press the plus and minus button.
package com.questdot.ratingbarexample; import android.content.SharedPreferences; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import android.widget.RatingBar; import android.widget.TextView; public class MainActivity extends AppCompatActivity { private TextView text; private RatingBar rating; private Button btnPlus,btnMinus,btnSave; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); rating = (RatingBar) findViewById(R.id.ratingBar); text = (TextView)findViewById(R.id.textView); btnMinus = (Button)findViewById(R.id.button); btnPlus = (Button)findViewById(R.id.button2); btnSave = (Button)findViewById(R.id.btnSave); rating.setOnRatingBarChangeListener( new RatingBar.OnRatingBarChangeListener() { @Override public void onRatingChanged(RatingBar ratingBar, float rating, boolean fromUser) { text.setText(String.valueOf(rating)); } } ); btnMinus.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { float rate =rating.getRating(); rating.setRating(rate-1); } }); btnPlus.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { float rate =rating.getRating(); rating.setRating(rate+1); } }); btnSave.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { SharedPreferences.Editor editor = getSharedPreferences("myrate", MODE_PRIVATE).edit(); editor.putFloat("rating", rating.getRating()); editor.commit(); } }); SharedPreferences prefs = getSharedPreferences("myrate", MODE_PRIVATE); if (prefs != null) { Float savedrate = prefs.getFloat("rating", 0f); rating.setRating(savedrate); } } }
Run your project
Finally, the simple ratingbar have been implement in your sample project, so you can try it now.