Android MediaPlayer Play Audio Tutorial
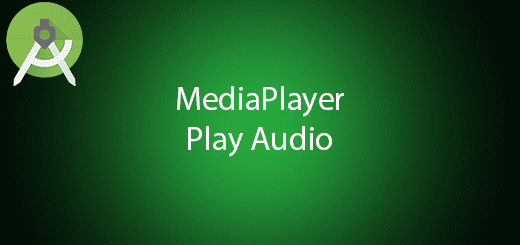
Android have many way to control playback of audio and video in the mobile application. In the Android SDK are come with MediaPlayer which allow the developer to play audio and video. Android MediaPlayer is a high level framework to let the developer easy to learn and use is a quick manner which does not require to understand how the mediaplayer work. For the mediaplayer drawbacks, it does not able to let the developer to customize the behaviour of the mediaplayer which is not flexible for us. In this tutorial, I will teach you on how to use android mediaplayer play audio in the android application.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “AudioPlayerExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit activity_main.xml
After create new project, add four buttons which are play, pause , stop and restart button so we can use it to perform the media player function.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.questdot.audioplayerexample.MainActivity"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Play" android:id="@+id/btnPlay" android:layout_marginTop="53dp" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Pause" android:id="@+id/btnPause" android:layout_below="@+id/btnPlay" android:layout_centerHorizontal="true" android:layout_marginTop="47dp" /> <Button style="?android:attr/buttonStyleSmall" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Restart" android:id="@+id/btnRestart" android:layout_below="@+id/btnPause" android:layout_centerHorizontal="true" android:layout_marginTop="66dp" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Stop" android:id="@+id/btnStop" android:layout_below="@+id/btnRestart" android:layout_centerHorizontal="true" android:layout_marginTop="45dp" /> </RelativeLayout>
Edit MainActivity.java class
In this class will implement the play, stop,restart and pause of the audio. There have 3 option to play the audio that you can see the following source code. In this class i load url to play the audio, while another two method is write in the comment line.
OnErrorListner to get the error occur in the mediaplayer.
OnPreaparedListener is perform when the media player is fully buffering the selected media.
OnCompletionListener is perform when it is finish to play the media.
package com.example.questdot.audioplayerexample; import android.app.ProgressDialog; import android.content.res.AssetFileDescriptor; import android.media.AudioManager; import android.media.MediaPlayer; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.view.Window; import android.widget.Button; import android.widget.ProgressBar; import java.io.File; public class MainActivity extends AppCompatActivity implements View.OnClickListener, MediaPlayer.OnErrorListener, MediaPlayer.OnPreparedListener, MediaPlayer.OnCompletionListener { static final String AUDIO_PATH = "http://www.tonycuffe.com/mp3/tail%20toddle.mp3"; private MediaPlayer mediaPlayer = new MediaPlayer(); private Button btnPlay,btnStop,btnPause,btnRestart; private int playbackPosition=0; private ProgressDialog progressDialog; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnPlay = (Button)findViewById(R.id.btnPlay); btnStop = (Button)findViewById(R.id.btnStop); btnPause = (Button)findViewById(R.id.btnPause); btnRestart = (Button)findViewById(R.id.btnRestart); btnPlay.setOnClickListener(this); btnStop.setOnClickListener(this); btnPause.setOnClickListener(this); btnRestart.setOnClickListener(this); } private void playAudio(String url) throws Exception { killMediaPlayer(); progressDialog = new ProgressDialog(this); progressDialog.setMessage("Buffering....."); progressDialog.show(); mediaPlayer = new MediaPlayer(); mediaPlayer.setAudioStreamType(AudioManager.STREAM_MUSIC); mediaPlayer.setOnPreparedListener(this); mediaPlayer.setOnErrorListener(this); mediaPlayer.setDataSource(url); // mediaPlayer.prepare(); mediaPlayer.prepareAsync(); mediaPlayer.setOnCompletionListener(this); // mediaPlayer.start(); //pb.setVisibility(View.VISIBLE); } private void playLocalAudio() throws Exception { mediaPlayer = MediaPlayer.create(this, R.raw.pokemon); mediaPlayer.start(); } private void playLocalAudio_UsingDescriptor() throws Exception { AssetFileDescriptor fileDesc = getResources().openRawResourceFd( R.raw.pokemon); if (fileDesc != null) { mediaPlayer = new MediaPlayer(); mediaPlayer.setDataSource(fileDesc.getFileDescriptor(), fileDesc .getStartOffset(), fileDesc.getLength()); fileDesc.close(); mediaPlayer.prepare(); mediaPlayer.start(); } } @Override protected void onDestroy() { super.onDestroy(); killMediaPlayer(); } private void killMediaPlayer() { if(mediaPlayer!=null) { try { mediaPlayer.release(); } catch(Exception e) { e.printStackTrace(); } } } @Override public void onClick(View view) { switch (view.getId()) { case R.id.btnPlay: try { // pb.setVisibility(View.VISIBLE); playAudio(AUDIO_PATH); //playLocalAudio(); // playLocalAudio_UsingDescriptor(); } catch (Exception e) { e.printStackTrace(); } break; case R.id.btnPause: if (mediaPlayer != null && mediaPlayer.isPlaying()) { playbackPosition = mediaPlayer.getCurrentPosition(); mediaPlayer.pause(); } break; case R.id.btnRestart: if (mediaPlayer != null && !mediaPlayer.isPlaying()) { mediaPlayer.seekTo(playbackPosition); mediaPlayer.start(); } break; case R.id.btnStop: if (mediaPlayer != null) { mediaPlayer.stop(); playbackPosition = 0; } break; } } @Override public boolean onError(MediaPlayer mediaPlayer, int i, int i1) { progressDialog.dismiss(); return false; } @Override public void onPrepared(MediaPlayer mediaPlayer) { // Log.i("StreamAudioDemo", "prepare finished"); progressDialog.setMessage("Playing....."); mediaPlayer.start(); } @Override public void onCompletion(MediaPlayer mediaPlayer) { progressDialog.dismiss(); } }
Run your project
Finally, you can run your project in your mobile device so you can listen the voice in your area.