Integrate Paypal SDK on Android to make payment
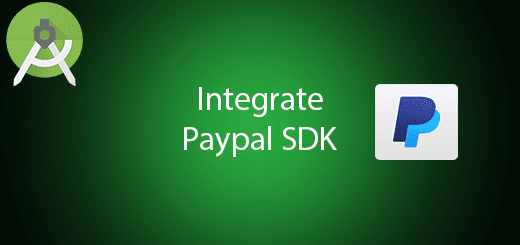
Paypal provides the official Paypal Android SDK to let the android developer easy to make payment by using PayPal in the android application. Paypal is the most popular payment method that used in the world which widely accepts in every country. As a developer, you can integrate the PayPal SDK in your android application so you can be selling your products or services to get some income. In this tutorial, I will teach you all the steps to integrate PayPal SDK on Android to make payment in the application. It is much safer, convenient and fast.
Register a Paypal developer account
In your browser navigate to the PayPal developer website link and log in your PayPal account or register a new account.
Create Sandbox Test Account
After that, go to //developer.paypal.com/developer/accounts to create a business account (merchant acount) and a personal account (buyer account).
Create REST API app
Go to //developer.paypal.com/developer/applications/ and create a new rest API app by clicking the create app button. After that, you will need to fill in some information.
Copy Client ID
After creating REST API app to test, click into the app name “Test”(this is my app name) to check out your client id and copy it, you will need to use the client id in the android project later.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “PayPalExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add dependency
Open build.gradle (Module: app) and add the following source code in your dependencies section to add the PayPal SDK in your app.
compile 'com.paypal.sdk:paypal-android-sdk:2.14.5'
Edit activity_main.xml layout
After that, go to your activity_main.xml to edit the layout. I will add a button to demo the PayPal payment method. You can follow the source code in the bottom section.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" > <LinearLayout android:id="@+id/linearLayout" android:orientation="vertical" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true"> </LinearLayout> <Button android:id="@+id/buttonPay" android:text="Pay $1 for Water" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
Edit MainActivity.java class
In this class, paste your client id in the PAYPAL_CLIENT_ID variable, in this class will perform all the paypal transaction in the application.
package com.questdot.paypalexample; import android.app.Activity; import android.content.DialogInterface; import android.content.Intent; import android.support.v7.app.AlertDialog; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.Button; import android.widget.EditText; import com.paypal.android.sdk.payments.PayPalConfiguration; import com.paypal.android.sdk.payments.PayPalPayment; import com.paypal.android.sdk.payments.PayPalService; import com.paypal.android.sdk.payments.PaymentActivity; import com.paypal.android.sdk.payments.PaymentConfirmation; import org.json.JSONException; import java.math.BigDecimal; public class MainActivity extends Activity implements View.OnClickListener{ public static final String PAYPAL_CLIENT_ID = "AbYB3mWJQUq8lqyEvnKRuXj1d08_q9rBYzE6HGSNi4vz9Q2uNjdC-SALmZi-lsw_LMx8V8DRETUaWMoP"; public static final int PAYPAL_REQUEST_CODE = 123; private Button buttonPay; private static PayPalConfiguration config = new PayPalConfiguration() // sandbox (ENVIRONMENT_SANDBOX) // or live (ENVIRONMENT_PRODUCTION) .environment(PayPalConfiguration.ENVIRONMENT_SANDBOX) .clientId(PAYPAL_CLIENT_ID); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); buttonPay = (Button) findViewById(R.id.buttonPay); buttonPay.setOnClickListener(this); Intent intent = new Intent(this, PayPalService.class); intent.putExtra(PayPalService.EXTRA_PAYPAL_CONFIGURATION, config); startService(intent); } @Override public void onDestroy() { stopService(new Intent(this, PayPalService.class)); super.onDestroy(); } @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { //If the result is from paypal if (requestCode == PAYPAL_REQUEST_CODE) { //If the result is OK i.e. user has not canceled the payment if (resultCode == Activity.RESULT_OK) { //Getting the payment confirmation PaymentConfirmation confirm = data.getParcelableExtra(PaymentActivity.EXTRA_RESULT_CONFIRMATION); //if confirmation is not null if (confirm != null) { try { //Getting the payment details new AlertDialog.Builder(MainActivity.this) .setTitle("Success") .setMessage(confirm.toJSONObject().toString(4)+"\n"+confirm.getPayment().toJSONObject().toString(4)) .setPositiveButton(android.R.string.yes, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { // continue with delete } }) .setNegativeButton(android.R.string.no, new DialogInterface.OnClickListener() { public void onClick(DialogInterface dialog, int which) { // do nothing } }) .setIcon(android.R.drawable.ic_dialog_info) .show(); } catch (JSONException e) { Log.e("paymentExample", "an extremely unlikely failure occurred: ", e); } } } else if (resultCode == Activity.RESULT_CANCELED) { Log.i("paymentExample", "The user canceled."); } else if (resultCode == PaymentActivity.RESULT_EXTRAS_INVALID) { Log.i("paymentExample", "An invalid Payment or PayPalConfiguration was submitted. Please see the docs."); } } } @Override public void onClick(View v) { //Creating a paypalpayment PayPalPayment payment = new PayPalPayment(new BigDecimal(String.valueOf(1)), "USD", "Water", PayPalPayment.PAYMENT_INTENT_SALE); Intent intent = new Intent(this, PaymentActivity.class); intent.putExtra(PayPalService.EXTRA_PAYPAL_CONFIGURATION, config); intent.putExtra(PaymentActivity.EXTRA_PAYMENT, payment); //Starting the intent activity for result //the request code will be used on the method onActivityResult startActivityForResult(intent, PAYPAL_REQUEST_CODE); } }
Run your project
Now, you can try and run it on your android device to make the payment with PayPal SDK.
Login Sandbox test account
After making the payment, navigate to //www.sandbox.paypal.com/my/webapps/mpp/home to log in your sandbox business account and personal account to check out the transaction.
(Integrate Paypal SDK on Android)
Source Code
Hy, thank you for your tutorial.
Please, what I need to change, when I put online the app and receive to receive the money?
Hi, i have an error when the login activity paypal show, the error is “Incorrect login” but my credentials are fine… do you know what could the problem?