Android Detect Gestures Tutorial
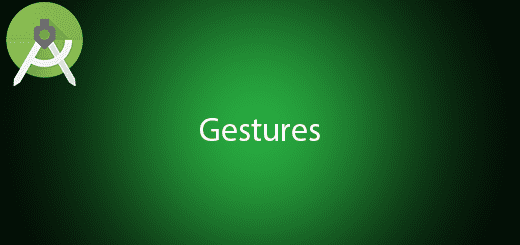
Gesture occurs when a user places one or more fingers on the touch screen, and your application interprets that pattern of touches as a particular gesture. Android provides special types of gestures such as pinch, double tap, scrolls, long presses and flinch. Android provides GestureDetector class to receive gestures events and tell us that these events occur in the smart phone. The purpose of the gesturedetector class is to Gathering data about touch events and Interpreting the data to see if it meets the criteria for any of the gestures your app supports. In this tutorial, I will tell you how to use android detect gestures in your new project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “GesturesExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit activity_main.xml layout
Add the textview inside the constraint-layout, add a new id for textview “txt_gesture” so that you can display the gesture status in the UI.
<?xml version="1.0" encoding="utf-8"?> <android.support.constraint.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.questdot.gesturesexample.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" android:id="@+id/txt_gesture" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintLeft_toLeftOf="parent" app:layout_constraintRight_toRightOf="parent" app:layout_constraintTop_toTopOf="parent" /> </android.support.constraint.ConstraintLayout>
Edit MainActivity.java class
Go to MainActivity.java class and implements GestureDectector listeners, After that initialize all callbacks from the listeners. You can follow the sample below.
package com.questdot.gesturesexample; import android.support.v4.view.GestureDetectorCompat; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.GestureDetector; import android.view.MotionEvent; import android.widget.TextView; public class MainActivity extends AppCompatActivity implements GestureDetector.OnGestureListener, GestureDetector.OnDoubleTapListener{ private TextView txt_gesture; private GestureDetectorCompat gestureDetectorCompat; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); txt_gesture = (TextView)findViewById(R.id.txt_gesture); gestureDetectorCompat = new GestureDetectorCompat(getApplicationContext(),this); gestureDetectorCompat.setOnDoubleTapListener(this); } @Override public boolean onSingleTapConfirmed(MotionEvent motionEvent) { txt_gesture.setText("On Single tap"); return true; } @Override public boolean onDoubleTap(MotionEvent motionEvent) { txt_gesture.setText("On Double tap"); return true; } @Override public boolean onDoubleTapEvent(MotionEvent motionEvent) { txt_gesture.setText("On Double tap event tap"); return true; } @Override public boolean onDown(MotionEvent motionEvent) { txt_gesture.setText("On Down "); return true; } @Override public void onShowPress(MotionEvent motionEvent) { txt_gesture.setText("On Show "); } @Override public boolean onSingleTapUp(MotionEvent motionEvent) { txt_gesture.setText("On Single tap up"); return true; } @Override public boolean onScroll(MotionEvent motionEvent, MotionEvent motionEvent1, float v, float v1) { txt_gesture.setText("On Scroll "); return true; } @Override public void onLongPress(MotionEvent motionEvent) { txt_gesture.setText("On Long Press"); } @Override public boolean onFling(MotionEvent motionEvent, MotionEvent motionEvent1, float v, float v1) { txt_gesture.setText("On Fling"); return true; } @Override public boolean onTouchEvent(MotionEvent event) { gestureDetectorCompat.onTouchEvent(event); return super.onTouchEvent(event); } }
Run Your Project
In conclusion, now you can run this project in the device and test out all the gestures listener that you implement.