Android Splash Screen & Progress Bar Tutorial
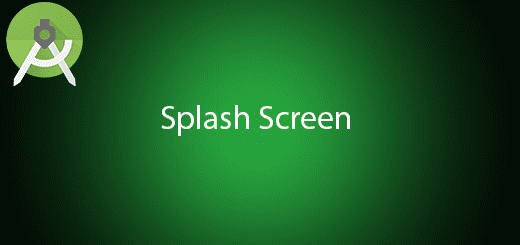
Splash Screen is a graphical image occur just a few second when starting the application. Android application may need to loading some heavy data in the beginning. In this situation, we using splash screen to load all the data before go to main activity. Sometime we use splash screen to identify our application name , logo and do some introduction about the application. In this tutorial, I will teach you how to make android splash screen in the application.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “SplashScreenExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add a New Empty Activity
Right click your java package name > New > Activity > Empty Activity to add a activity. Rename your activity name as SplashScreen. In this steps, it will automatically generate xml and java file for splash screen activity.
Edit activity_splash_screen.xml layout
Go to activity_splash_screen.xml that just automatic generate. Add ProgressBar in this layout and put the style to the following source code.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent"> <ProgressBar android:id="@+id/progressBar" style="?android:attr/progressBarStyleLarge" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
Edit SplashScreen.java class
Next, navigate to splashscreen.java and edit to the following source code. This class will handler 2 seconds delay to the MainActivity. I set this activity to full screen with no action bar.
package com.example.questdot.splashscreenexample; import android.app.Activity; import android.content.Intent; import android.os.Handler; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.view.Window; import android.view.WindowManager; import android.widget.ProgressBar; public class SplashScreen extends Activity { private ProgressBar spinner; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); requestWindowFeature(Window.FEATURE_NO_TITLE); getWindow().setFlags(WindowManager.LayoutParams.FLAG_FULLSCREEN, WindowManager.LayoutParams.FLAG_FULLSCREEN); setContentView(R.layout.activity_splash_screen); spinner = (ProgressBar)findViewById(R.id.progressBar); //hi new Handler().postDelayed(new Runnable(){ @Override public void run() { Intent mainIntent = new Intent(SplashScreen.this,MainActivity.class); SplashScreen.this.startActivity(mainIntent); SplashScreen.this.finish(); } }, 2000); } }
Edit activity_main.xml layout
Last, go to activity_main.xml and edit text field to indicant you are navigate to this layout.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.questdot.splashscreenexample.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Hello World!" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
Run your project
Finally, you can run your project in your android application. It is just simple, you can test the feature to find out the behaviour of source code.