Android Model View Presenter MVP Tutorial
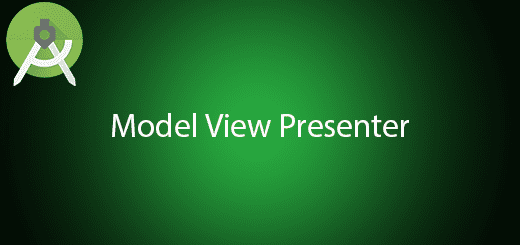
MVP Model View Presenter is the best and most suitable architectural patterns for android development. There have many architectural patterns and why we use Model View Presenter instead of Model View Controller(MVC). It is because activity in android contains view and controller. The Activity class in android doesn’t extend View class but it is handle displaying a dialog and toast to the user. Besides, it also handle the events of windows such as onCreate,onPause and onResume. If you using MVC pattern, the controller will actually be a View-Controller because it is handling display window and activity life cycle event. Therefore, we should use MVP for android developer since the Views in this architectural pattern are serving as a entry point, rendering components and routing user events to the presenter. In this tutorial, I will teach you how to use Android Model View Presenter MVP in your project.
Model basically gateway to the domain layer or business logic and it will help making your domain layer more tight.
View contains your UI components and its almost zero logic.
Presenter will handle communication between your model and your view. It also handler events.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “MVPExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit activity_main.xml layout
After create the new project, go to edit the main activity layout to the source code below.
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context="com.questdot.mvpexample.MainActivity"> <ListView android:id="@+id/list" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" /> <ProgressBar android:id="@+id/progress" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center" android:visibility="gone" /> </FrameLayout>
Create new interface for View
Right click the project name and create a new interface “MainView”. This interface provide all view method in the activity.
public interface MainView { void showProgress(); void hideProgress(); void setItems(List<String> items); void showMessage(String message); }
Create new interface for Presenter
After that also create a new interface “MainPresenter”. This interface handler all event in the mainactivity.
public interface MainPresenter { void onResume(); void onItemClicked(int position); void onDestroy(); }
Edit MainActivity.java class
Go to this class and edit to the following sample source code. This activity implements MainView to have all method on it and pass event to the presenter to handler.
import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.AdapterView; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.ProgressBar; import android.widget.Toast; import java.util.List; public class MainActivity extends AppCompatActivity implements MainView, AdapterView.OnItemClickListener { private ListView listView; private ProgressBar progressBar; private MainPresenter presenter; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); listView = (ListView) findViewById(R.id.list); listView.setOnItemClickListener(this); progressBar = (ProgressBar) findViewById(R.id.progress); presenter = new MainPresenterImpl(this, new LoadItemsInteractorImpl()); } @Override protected void onResume() { super.onResume(); presenter.onResume(); } @Override protected void onDestroy() { presenter.onDestroy(); super.onDestroy(); } @Override public void showProgress() { progressBar.setVisibility(View.VISIBLE); listView.setVisibility(View.INVISIBLE); } @Override public void hideProgress() { progressBar.setVisibility(View.INVISIBLE); listView.setVisibility(View.VISIBLE); } @Override public void setItems(List<String> items) { listView.setAdapter(new ArrayAdapter<>(this, android.R.layout.simple_list_item_1, items)); } @Override public void showMessage(String message) { Toast.makeText(this, message, Toast.LENGTH_LONG).show(); } @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { presenter.onItemClicked(position); } }
Create new interface for interactor(Model)
Besides, we also create a new interface “LoadItemInteractor”. What is interactor? its help you to fetch all data from web server or database. But in this tutorial we only fetch some dummy data.
public interface LoadItemsInteractor { interface OnFinishedListener { void onFinished(List<String> items); } void findItems(OnFinishedListener listener); }
Create a new class for Presenter
This class will be implements all method from MainPresenter interface.
public class MainPresenterImpl implements MainPresenter, LoadItemsInteractor.OnFinishedListener { private MainView mainView; private LoadItemsInteractor findItemsInteractor; public MainPresenterImpl(MainView mainView, LoadItemsInteractor findItemsInteractor) { this.mainView = mainView; this.findItemsInteractor = findItemsInteractor; } @Override public void onResume() { if (mainView != null) { mainView.showProgress(); } findItemsInteractor.findItems(this); } @Override public void onItemClicked(int position) { if (mainView != null) { mainView.showMessage("Position "+(position + 1)+" clicked"); } } @Override public void onDestroy() { mainView = null; } @Override public void onFinished(List<String> items) { if (mainView != null) { mainView.setItems(items); mainView.hideProgress(); } } public MainView getMainView() { return mainView; } }
Create a new class for Interactor
This class will be implements all method from LoadItemsInteractor and fetch all the dummy data.
public class LoadItemsInteractorImpl implements LoadItemsInteractor { @Override public void findItems(final OnFinishedListener listener) { new Handler().postDelayed(new Runnable() { @Override public void run() { listener.onFinished(createArrayList()); } }, 2000); } private List<String> createArrayList() { return Arrays.asList( "1", "2", "3", "4", "5", "6", "7", "8", "9", "10" ); } }
Run Your Project
Finally, the mvp pattern is completed in your project, now you can run it and view on your emulator or devices.
Good for quick start to using MVP in Android
When i click on download!! i gets this msg =>“Your requested URL has been blocked as per the directions received from Department of Telecommunications, Government of India.”