Android 2D Card Flip Animation Tutorial
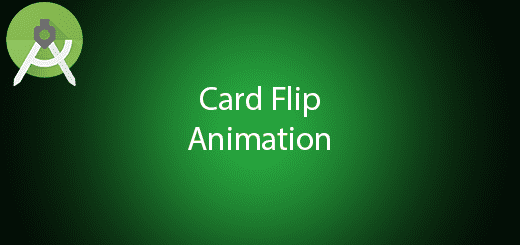
2D Card flip animation will be implement by animate flipping effect between two imageview in the Framelayout. It is very useful in the particular situation for example you want to display a picture with both sides. You can use two imageview in one frame layout and create the card flip effect, so it will seem like a virtual card in the device. In this tutorial, I will give you the source code on how to create this effect in the imageview. Its just very easy you just need to follow my steps.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “2DCardExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add 2 image in the res> drawable folder
You can download any image from the internet and copy paste those image in the res > drawable folder.
Edit activity_main.xml layout
Change the relative layout to frame layout and I will add two imageview in this frame layout. You can copy the source code below.
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:id="@+id/root" > <ImageView android:id="@+id/ivA" android:layout_width="fill_parent" android:layout_height="fill_parent" android:src="@drawable/image_a" /> <ImageView android:id="@+id/ivB" android:layout_width="fill_parent" android:layout_height="fill_parent" android:src="@drawable/image_b" /> </FrameLayout>
Edit MainActivity.java class
Open MainActivity.java and copy paste the following source code. This java class is to create the flipping animation between two image.
import android.app.Activity; import android.os.Bundle; import android.view.Menu; import android.view.MenuItem; import android.view.View; import android.view.View.OnClickListener; import android.view.animation.Animation; import android.view.animation.Animation.AnimationListener; import android.view.animation.ScaleAnimation; import android.widget.ImageView; public class MainActivity extends Activity { private ImageView imageA; private ImageView imageB; private ScaleAnimation sato0 = new ScaleAnimation(1, 0, 1, 1, Animation.RELATIVE_TO_PARENT, 0.5f, Animation.RELATIVE_TO_PARENT, 0.5f); private ScaleAnimation sato1 = new ScaleAnimation(0, 1, 1, 1, Animation.RELATIVE_TO_PARENT, 0.5f, Animation.RELATIVE_TO_PARENT, 0.5f); @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); initView(); findViewById(R.id.root).setOnClickListener(new OnClickListener() { @Override public void onClick(View v) { if (imageA.getVisibility() == View.VISIBLE) { imageA.startAnimation(sato0); }else{ imageB.startAnimation(sato0); } } }); } private void shwoImageA(){ imageA.setVisibility(View.VISIBLE); imageB.setVisibility(View.INVISIBLE); } private void showImageB(){ imageA.setVisibility(View.INVISIBLE); imageB.setVisibility(View.VISIBLE); } private void initView(){ imageA = (ImageView) findViewById(R.id.ivA); imageB = (ImageView) findViewById(R.id.ivB); shwoImageA(); sato0.setDuration(500); sato1.setDuration(500); sato0.setAnimationListener(new AnimationListener() { @Override public void onAnimationStart(Animation animation) { // TODO Auto-generated method stub } @Override public void onAnimationRepeat(Animation animation) { // TODO Auto-generated method stub } @Override public void onAnimationEnd(Animation animation) { if (imageA.getVisibility() == View.VISIBLE) { imageA.setAnimation(null); showImageB(); imageB.startAnimation(sato1); }else{ imageB.setAnimation(null); shwoImageA(); imageA.startAnimation(sato1); } } }); } }
Run your Project
Now, you can run it on your android device to test out the flipping effect.