Android Dagger 2 Dependency Injection Tutorial
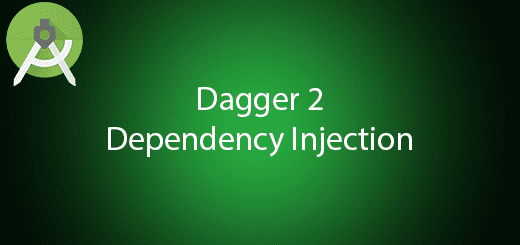
What is Dependency Injection in Android? Dependency Injection is a practice where objects are designed in a manner where they receive instances of the objects from other pieces of code, instead of constructing them internally. This means that any object implementing the interface which is required by the object can be substituted in without changing the code, which simplifies testing, and improves decoupling. It makes the project more easy to maintain and testable. In this tutorial, I will tell you on how to use android dagger 2 dependency injection in your own project.
For example, consider a Car
object.
A Car
depends on (uses) wheels, engine, fuel, battery, etc. to run.
Without Dependency Injection (DI):
class Car{
private Wheel wh= new NepaliRubberWheel();
private Battery bt= new ExcideBattery();
//The rest
}
With Dependency Injection (DI):
class Car{
private Wheel wh= [Inject an Instance of Wheel (dependency of car) at runtime]
private Battery bt= [Inject an Instance of Battery (dependency of car) at runtime]
Car(Wheel wh,Battery bt) {
this.wh = wh;
this.bt = bt;
}
//Or we can have setters
void setWheel(Wheel wh) {
this.wh = wh;
}
}
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “Dagger2Example”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add new dependency
Open your Gradle file and add dagger 2 library in the dependency section.
compile 'com.google.dagger:dagger:2.10' annotationProcessor 'com.google.dagger:dagger-compiler:2.10'
Create ToastUtil class
Create a new class file name “ToastUtil” and Edit this class to the sample source code.
public class ToastUtil { private Context mContext; public ToastUtil(Context context){ this.mContext = context; } public void showToast(String message){ Toast.makeText(mContext,message, Toast.LENGTH_LONG).show(); } }
Create Application class
After that also create an application class to init the dagger app component to all activity.
public class App extends Application { AppComponent mAppComponent; @Override public void onCreate() { super.onCreate(); mAppComponent = DaggerAppComponent.builder().appModule(new AppModule(this)).build(); } public AppComponent getAppComponent(){ return mAppComponent; } }
Create an AppModule class
Add another new class for AppModule, this class provides the context and ToastUtil instances to the whole app.
@Module public class AppModule { Context context; public AppModule(Context context){ this.context = context; } @Provides @Singleton public Context provideContext(){ return context; } @Provides @Singleton public ToastUtil provideToastUtil(){ return new ToastUtil(context); } }
Create an interface
Create a new interface “AppComponent”, this interface is to get all the instances provide by the module class.
@Singleton @Component(modules={AppModule.class}) public interface AppComponent { Context getContext(); ToastUtil getToastUtil(); // Test test(); }
Create MainModule class
This class is for MainActivity, it provides the static text to the MainActivity.
@Module public class MainModule { @Provides public String providesHello(){ return "Main Hello"; } }
Create PerActivity annotation
This annotation is to make sure only perform when the activity is running.
@Scope @Retention(RetentionPolicy.RUNTIME) public @interface PerActivity { }
Create a new interface
Create an interface “MainComponent”, this component will inject the mainActivity class, so that you can get the helloText from the MainModule.
@PerActivity @Component(dependencies = AppComponent.class,modules = {MainModule.class}) public interface MainComponent { void inject(MainActivity mainActivity); }
Edit MainActivity class
Go to this file and edit this file to the following code. It will display 2 difference toast.
public class MainActivity extends AppCompatActivity { private MainComponent mMainComponent; @Inject ToastUtil toastUtil; @Inject String helloText; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mMainComponent = DaggerMainComponent.builder().appComponent(getAppComponent()) .mainModule(new MainModule()) .build(); mMainComponent.inject(this); toastUtil.showToast("Custom Toast Message"); toastUtil.showToast(helloText); } public AppComponent getAppComponent(){ return ((App)getApplication()).getAppComponent(); } }
Edit AndroidManifest.xml
Add android:name =”.App” so that your project will run this file on the beginning.
<application android:name=".App" android:allowBackup="true" android:icon="@mipmap/ic_launcher" android:label="@string/app_name" android:roundIcon="@mipmap/ic_launcher_round" android:supportsRtl="true" android:theme="@style/AppTheme"> <activity android:name=".MainActivity"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application>
Run Your Project
In conclusion, now you can check it out how the dagger 2 works in this project. Run it on your emulator.