Android Text to Speech Tutorial
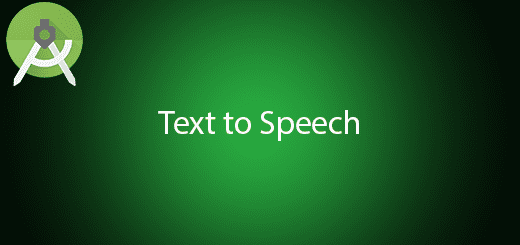
Android allows you convert your text into voice. Therefore, Android providing a feature/class to translate text to speech called (TTS) which can speak the text in different languages. The user can also adjust the speech speed and pitch during the time of generating the text to voice. TTS is good for education purpose in general so that it will provide the user the proper pronunciation of the language. The user can select which language they want to translate such as English, Chinese, Tamil and more. In this tutorial, I will tell you how to implement android text to speech feature in your project.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “SpeechExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit activity_main.xml layout
Go to the mainactivity layout and add two seekbar to adjust the pitch and speed of the voice generated by the text. You can follow the sample at the bottom.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:padding="16dp" tools:context=".MainActivity"> <TextView android:text="Write here the sentence to read" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="20dp" android:id="@+id/txt"/> <EditText android:layout_width="fill_parent" android:layout_height="wrap_content" android:id="@+id/sentence" android:layout_below="@id/txt"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/txtPitch" android:layout_below="@id/sentence" android:text="Pitch" android:layout_marginTop="10dp" /> <SeekBar android:layout_width="200dp" android:layout_height="wrap_content" android:layout_marginTop="5dp" android:layout_below="@id/txtPitch" android:max="10" android:id="@+id/seekPitch" android:progress="5"/> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/txtSpeed" android:layout_below="@id/seekPitch" android:text="Speed" android:layout_marginTop="10dp" /> <SeekBar android:layout_width="200dp" android:layout_height="wrap_content" android:layout_marginTop="5dp" android:layout_below="@id/txtSpeed" android:max="10" android:id="@+id/seekSpeed" android:progress="5"/> <ImageView android:id="@+id/speechImg" android:layout_width="90dp" android:layout_height="90dp" android:layout_alignParentBottom="true" android:layout_centerHorizontal="true" android:layout_marginBottom="10dp" android:src="@android:drawable/ic_btn_speak_now" /> </RelativeLayout>
Edit MainActivity.java class
After modifying the layout, go to this class and performs the text to speech feature when the user clicks the speech image button. Copy and paste the sample below in your MainActivity class.
public class MainActivity extends AppCompatActivity implements TextToSpeech.OnInitListener { private ImageView speechButton; private TextToSpeech engine; private EditText editText; private SeekBar seekPitch; private SeekBar seekSpeed; private double pitch=1.0; private double speed=1.0; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); // Get reference speechButton = (ImageView) findViewById(R.id.speechImg); editText = (EditText) findViewById(R.id.sentence); engine = new TextToSpeech(this, this); speechButton.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { speech(); } }); seekPitch = (SeekBar) findViewById(R.id.seekPitch); seekPitch.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { //Log.d("Speech", "Progress ["+progress+"]"); pitch = (float) progress / (seekBar.getMax() / 2); //Log.d("Speech", "Pitch ["+pitch+"]"); } @Override public void onStartTrackingTouch(SeekBar seekBar) { } @Override public void onStopTrackingTouch(SeekBar seekBar) { } }); seekSpeed = (SeekBar) findViewById(R.id.seekSpeed); seekSpeed.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() { @Override public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) { //Log.d("Speech", "Progress ["+progress+"]"); speed = (float) progress / (seekBar.getMax() / 2); //Log.d("Speech", "Pitch ["+pitch+"]"); } @Override public void onStartTrackingTouch(SeekBar seekBar) { } @Override public void onStopTrackingTouch(SeekBar seekBar) { } }); } @Override public void onInit(int status) { Log.d("Speech", "OnInit - Status ["+status+"]"); if (status == TextToSpeech.SUCCESS) { Log.d("Speech", "Success!"); engine.setLanguage(Locale.UK); } } private void speech() { engine.setPitch((float) pitch); engine.setSpeechRate((float) speed); if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.LOLLIPOP) { engine.speak(editText.getText().toString(),TextToSpeech.QUEUE_FLUSH,null,null); } else { engine.speak(editText.getText().toString(), TextToSpeech.QUEUE_FLUSH, null); } } }
Run Your Project
Lastly, you can now run this project in your real device to test out this feature is working anot. Please make sure you volumn up your mobile device.