Android AIDL Remote Service Tutorial
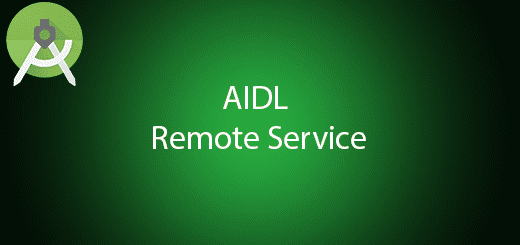
What is AIDL in android? AIDL is Android Interface definition language to establish the communication both application that running at different process. The communication is using IPC inter process communication which allow the process communicate together. In Android OS, different process cannot access to another process. Therefore, Android was solve this problem by using AIDL convert the object to primitive so that the Android OS will understand. It also name as remote service. In this tutorial, i will give you the example and show how the its work in android. It is just easy if you can understand the process.
Create a new project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “AIDL_SBServiceExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Project” and Click Next button
5. Lastly, press finish button.
Add a new Service
Create a new service in your package name by right click > Add > Service > Service. Enter the Service name as “AIDLService”, after that click OK button. Once service class created, you will see <service> is include in your AndroidManist.xml.
<service android:name=".AIDLService" android:enabled="true" android:exported="true"></service>
Create a new aidl file
To add a aidl interface by right click the package name > New > AIDL >AIDL File. The aidl default name is IMyAidlInterface you can change it or let it default. After that click finish button.
After that, it will automatic generate the aidl package for your aidl file.
Edit IMyAdilInterface.aidl interface
Open your IMyAidlInterface.aidl in your aidl package and insert the following code.
// IMyAidlInterface.aidl package com.example.questdot.aidl_sbserviceexample; // Declare any non-default types here with import statements interface IMyAidlInterface { /** * Demonstrates some basic types that you can use as parameters * and return values in AIDL. */ void basicTypes(int anInt, long aLong, boolean aBoolean, float aFloat, double aDouble, String aString); void setData(String data); }
Edit AIDLService.java class
After that, go to your AIDLService.java add the following code.
package com.example.questdot.aidl_sbserviceexample; import android.app.Service; import android.content.Intent; import android.os.IBinder; import android.os.RemoteException; public class AIDLService extends Service { public AIDLService() { } @Override public IBinder onBind(Intent intent) { return new IMyAidlInterface.Stub() { @Override public void basicTypes(int anInt, long aLong, boolean aBoolean, float aFloat, double aDouble, String aString) throws RemoteException { } @Override public void setData(String data) throws RemoteException { AIDLService.this.data=data; } }; } @Override public void onCreate(){ super.onCreate(); System.out.println("Service started"); new Thread(){ @Override public void run() { super.run(); running= true; while(running){ System.out.println(data); try { Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } }.start(); } @Override public void onDestroy() { super.onDestroy(); System.out.println("Service destroyed"); running=false; } private String data ="default"; private boolean running = false; }
Add a new Module in your project
Create a new module name “anotherapp” to start, bind and syn data to the module “app”(service). Different module is a different application, so they have different process. The module “anotherapp” is to connect the service from the “app” module. To create a new module, in the topbar click File > New > New Module.
It will pop out a task, select Phone and Tablet Module, Next and pick the Empty Activity finally finish.
Edit activity_main.xml layout
In the “anotherapp” module, go to the activity_main.xml in the layout folder edit the source code.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context="com.example.questdot.myapplication.MainActivity"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Start Remote Service" android:id="@+id/Startbtn" android:onClick="StartOnClick" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Stop Remote Service" android:id="@+id/Stopbtn" android:onClick="StopOnClick" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="BIND REMOTE SERVICE" android:id="@+id/Bindbtn" android:onClick="BindOnClick" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="UNBIND REMOTE SERVICE" android:id="@+id/Unbindbtn" android:onClick="UnbindOnClick" /> <EditText android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/editText" android:text="hi" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="SYNCHONISE" android:id="@+id/Synbtn" android:onClick="SynOnClick" /> </LinearLayout>
Add a new AIDL folder
In the “anotherapp” module add a new aidl folder.
Copy aidl package and paste it
Copy the aidl package in the “app” module and paste it in your aidl package in the “anotherapp” module. The package name and aidl file name should same as module “app”.
Edit MainActivity.java class
In the module “anotherapp“, go to the MainActivity.java and add the following code.
package com.example.questdot.myapplication; import android.content.ComponentName; import android.content.Context; import android.content.Intent; import android.content.ServiceConnection; import android.os.IBinder; import android.os.RemoteException; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.EditText; import com.example.questdot.aidl_sbserviceexample.IMyAidlInterface; public class MainActivity extends AppCompatActivity implements ServiceConnection { private Intent serviceIntent; private EditText editText; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); editText = (EditText)findViewById(R.id.editText); serviceIntent= new Intent(); serviceIntent.setComponent(new ComponentName("com.example.questdot.aidl_sbserviceexample","com.example.questdot.aidl_sbserviceexample.AIDLService")); } public void StartOnClick(View v){ startService(serviceIntent); } public void BindOnClick(View v){ bindService(serviceIntent,this, Context.BIND_AUTO_CREATE); } public void UnbindOnClick(View v){ unbindService(this); binder=null; } public void StopOnClick(View v){ stopService(serviceIntent); } public void SynOnClick(View v){ if(binder!=null){ try { binder.setData(editText.getText().toString()); } catch (RemoteException e) { e.printStackTrace(); } } } @Override public void onServiceConnected(ComponentName name, IBinder service) { System.out.println("Connected"+service); binder = IMyAidlInterface.Stub.asInterface(service); } @Override public void onServiceDisconnected(ComponentName name) { } private IMyAidlInterface binder = null; }
Run “app” module first
You need to start the module “app” first, after that that only start the module “anotherapp” to test the service in your device.
Yes… you just done this project. You can test out the application and understand how its work.