Android Download Manager download from url Tutorial
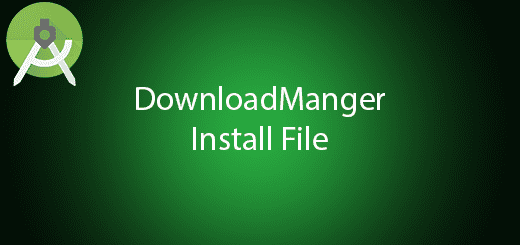
To make your mobile application able to download from http url is majorly use for download mp3 music , images file and apk from websites. Android provide the DownloadManager Object to let android application to download all file from internet that can specific by the developers which can provide better experience to the user. The DownloadManager will conduct the installing process in the background of the application and it is take caring when the connection is failure or system reboots. The broadcastreceiver which can use to combine with download manager for notify downloaded file. In this tutorial, I will teach you how to use android download manager download from url and some other feature of download manager.
Create a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “FileDownloadExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Project” and Click Next button
5. Lastly, press finish button.
Add permissions
Open your AndroidManifest.xml file, adding the internet, read and write external permissions outside the application level so u can access those feature in the application.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.INTERNET"/>
Create a new xml item
Res> layout > right click > new > layout resource file and name the resource to down_load_task_item.xml. This layout is to show the downloaded item in the application.
Edit down_load_task_item.xml layout
Go to down_load_task_item and change your source code to the source code that i provided in the below section.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/downid"/> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/title"/> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/status"/> <TextView android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/address"/> </LinearLayout>
Edit activity_main.xml layout
After that, navigate to your activity_main.xml to edit the main page layout of your android application. It will have 4 basic buttons to perform different actions which are Download, Delete, Download with display progress and Display Downloaded file. The source code will be…
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" > <Button android:id="@+id/btnDown" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Download" /> <Button android:id="@+id/btnDelete" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Delete" /> <Button android:id="@+id/btnDProgress" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Download (display progress)" /> <Button android:id="@+id/btndisplay" android:layout_width="match_parent" android:layout_height="wrap_content" android:text="Display All" /> <ProgressBar android:layout_width="match_parent" android:layout_height="wrap_content" android:id="@+id/downProgress" style="?android:attr/progressBarStyleHorizontal"/> <ListView android:id="@+id/mylist" android:layout_width="match_parent" android:layout_height="wrap_content"/> </LinearLayout>
Create a Broadcastreceiver
Java > right click your package name and create a new java class and name it to DownloadCompleteReceiver after that copy and paste the following source code below.
import android.app.DownloadManager; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.widget.Toast; /** * Created by HP on 16/9/2016. */ class DownLoadCompleteReceiver extends BroadcastReceiver { private MainActivity mainActivity; public DownLoadCompleteReceiver(MainActivity mainActivity) { this.mainActivity = mainActivity; } @Override public void onReceive(Context context, Intent intent) { if (intent.getAction().equals(DownloadManager.ACTION_DOWNLOAD_COMPLETE)) { long id = intent.getLongExtra(DownloadManager.EXTRA_DOWNLOAD_ID, -1); Toast.makeText(mainActivity, "Completed", Toast.LENGTH_SHORT).show(); } else if (intent.getAction().equals(DownloadManager.ACTION_NOTIFICATION_CLICKED)) { Toast.makeText(mainActivity, "Clicked", Toast.LENGTH_SHORT).show(); } } } <br>
Edit MainActivity.java class
Navigate to your mainActivity.java class and paste the following source code. In this application will download the mp3 file from the url.
package com.questdot.filedownloadexample; import android.app.DownloadManager; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.content.IntentFilter; import android.database.Cursor; import android.net.Uri; import android.os.Environment; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.ListView; import android.widget.ProgressBar; import android.widget.SimpleAdapter; import android.widget.Toast; import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map; import java.util.concurrent.Executors; import java.util.concurrent.ScheduledExecutorService; import java.util.concurrent.TimeUnit; public class MainActivity extends AppCompatActivity implements View.OnClickListener { long id; long idPro; private ProgressBar progressBar; private ListView mlistView; private SimpleAdapter adapter; private List<Map<String, String>> data ; DownloadManager downManager ; private DownLoadCompleteReceiver receiver; @Override protected void onCreate(Bundle savedInstanceState) { // TODO Auto-generated method stub super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); findViewById(R.id.btnDown).setOnClickListener(this); findViewById(R.id.btndisplay).setOnClickListener(this); findViewById(R.id.btnDelete).setOnClickListener(this); findViewById(R.id.btnDProgress).setOnClickListener(this); mlistView = (ListView)findViewById(R.id.mylist); downManager = (DownloadManager)getSystemService(Context.DOWNLOAD_SERVICE); progressBar = (ProgressBar) (findViewById(R.id.downProgress)); data = new ArrayList<Map<String,String>>(); adapter = new SimpleAdapter(this, data, R.layout.down_load_task_item, new String[]{"downid","title","address","status"}, new int[]{R.id.downid,R.id.title,R.id.address,R.id.status}); mlistView.setAdapter(adapter); mlistView.setOnItemClickListener(new AdapterView.OnItemClickListener() { @Override public void onItemClick(AdapterView<?> parent, View view, int position, long id) { Map<String, String> map = data.get(position); downManager.remove(Long.valueOf(map.get("downid"))); //adapter.notifyDataSetChanged(); } }); IntentFilter filter = new IntentFilter(); filter.addAction(DownloadManager.ACTION_DOWNLOAD_COMPLETE); filter.addAction(DownloadManager.ACTION_NOTIFICATION_CLICKED); receiver = new DownLoadCompleteReceiver(this); registerReceiver(receiver, filter); } @Override public void onClick(View v) { DownloadManager.Request request = new DownloadManager.Request(Uri.parse("http://www.stephaniequinn.com/Music/Allegro%20from%20Duet%20in%20C%20Major.mp3")); request.setAllowedNetworkTypes(DownloadManager.Request.NETWORK_WIFI); request.setNotificationVisibility(DownloadManager.Request.VISIBILITY_VISIBLE); request.setTitle("Download"); request.setDescription("Your file is downloading..."); request.setAllowedOverRoaming(false); request.setDestinationInExternalFilesDir(this, Environment.DIRECTORY_DOWNLOADS, "mydown"); switch (v.getId()) { case R.id.btnDown: id= downManager.enqueue(request); break; case R.id.btndisplay: data.clear(); queryDownTask(downManager,DownloadManager.STATUS_FAILED); queryDownTask(downManager,DownloadManager.STATUS_PAUSED); queryDownTask(downManager,DownloadManager.STATUS_PENDING); queryDownTask(downManager,DownloadManager.STATUS_RUNNING); queryDownTask(downManager,DownloadManager.STATUS_SUCCESSFUL); adapter.notifyDataSetChanged(); break; case R.id.btnDelete: downManager.remove(id); break; case R.id.btnDProgress: idPro= downManager.enqueue(request); ScheduledExecutorService ses = Executors.newSingleThreadScheduledExecutor(); ses.scheduleAtFixedRate(new Runnable() { @Override public void run() { queryTaskByIdandUpdateView(idPro); } }, 0, 2, TimeUnit.SECONDS); break; default: break; } } private void queryDownTask(DownloadManager downManager,int status) { DownloadManager.Query query = new DownloadManager.Query(); query.setFilterByStatus(status); Cursor cursor= downManager.query(query); while(cursor.moveToNext()){ String downId= cursor.getString(cursor.getColumnIndex(DownloadManager.COLUMN_ID)); String title = cursor.getString(cursor.getColumnIndex(DownloadManager.COLUMN_TITLE)); String address = cursor.getString(cursor.getColumnIndex(DownloadManager.COLUMN_LOCAL_URI)); //String statuss = cursor.getString(cursor.getColumnIndex(DownloadManager.COLUMN_STATUS)); String size= cursor.getString(cursor.getColumnIndex(DownloadManager.COLUMN_BYTES_DOWNLOADED_SO_FAR)); String sizeTotal = cursor.getString(cursor.getColumnIndex(DownloadManager.COLUMN_TOTAL_SIZE_BYTES)); Map<String, String> map = new HashMap<String, String>(); map.put("downid", downId); map.put("title", title); map.put("address", address); map.put("status", sizeTotal+":"+size); this.data.add(map); } cursor.close(); } private void queryTaskByIdandUpdateView(long id){ DownloadManager.Query query = new DownloadManager.Query(); query.setFilterById(id); Cursor cursor= downManager.query(query); String size="0"; String sizeTotal="0"; if(cursor.moveToNext()){ size= cursor.getString(cursor.getColumnIndex(DownloadManager.COLUMN_BYTES_DOWNLOADED_SO_FAR)); sizeTotal = cursor.getString(cursor.getColumnIndex(DownloadManager.COLUMN_TOTAL_SIZE_BYTES)); } cursor.close(); progressBar.setMax(Integer.valueOf(sizeTotal)); progressBar.setProgress(Integer.valueOf(size)); } @Override protected void onDestroy() { // TODO Auto-generated method stub super.onDestroy(); unregisterReceiver(receiver); // Intent intent = new Intent(Intent.ACTION_VIEW); // intent.setDataAndType(Uri.fromFile(new File(mUrl)), // "application/vnd.android.package-archive"); } }
Run your project
Finally, you can run it and download file from the url, you also can check what you are download in your application.