Android Integrate Facebook Login Tutorial
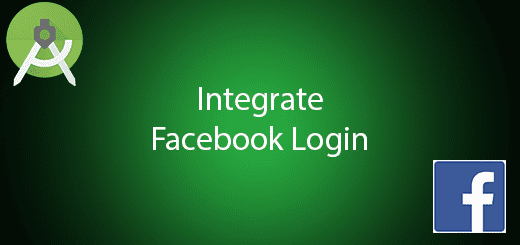
Most of the time login with password maybe not convenience to the user in the mobile application. Therefore, facebook provide a SDK that allow the android application login with just using their facebook account. It no require register an account in the mobile application, mobile user just need to click the facebook login button to use the application. By using facebook SDK, developer allow to get user profile information from the facebook database. Therefore, it is a very nice feature for both developer and user. In this tutorial, I will give you the steps on how to use facebook SDK to perform one click login by using facebook account.
Register a Facebook Developer Account
Enter this link https://developers.facebook.com/ in your browser and register your facebook account to developer account.
Create a New App ID
In the right corner click your profile and select “Add a New App”.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “FacebookLoginExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Edit build.gradle (module:app)
Go to your build.gradle (module:app) file and add maven central responsitory before dependencies.
repositories { mavenCentral() }
After that add dependencies for compiling the facebook sdk provided by facebook.
dependencies { compile fileTree(dir: 'libs', include: ['*.jar']) testCompile 'junit:junit:4.12' compile 'com.facebook.android:facebook-android-sdk:[4,5)' compile 'com.android.support:appcompat-v7:23.0.0' compile 'com.android.support:support-v4:23.4.0' }
Then click build button to build the latest changes on build.gradle.
Edit strings.xml file
You can check your app id from your app name dashboard or click this link to check your app id https://developers.facebook.com/apps/.
<string name="facebook_app_id">your app id</string>
Edit Android.Manifest.xml
Add the internet permission in the android.manifest file so your app able to use internet access.
<uses-permission android:name="android.permission.INTERNET"/>
After that add facebook meta data element in the application element.
<application android:label="@string/app_name" ...> ... <meta-data android:name="com.facebook.sdk.ApplicationId" android:value="@string/facebook_app_id"/> ... <activity android:name="com.facebook.FacebookActivity" android:configChanges= "keyboard|keyboardHidden|screenLayout|screenSize|orientation" android:theme="@android:style/Theme.Translucent.NoTitleBar" android:label="@string/app_name" /> </application>
Generate Key Hashes
Go to your MainActivity.java and insert the following source code.
public void getKeyHash(){ try { PackageInfo info = getPackageManager().getPackageInfo( getPackageName(), PackageManager.GET_SIGNATURES); for (Signature signature : info.signatures) { MessageDigest md = MessageDigest.getInstance("SHA"); md.update(signature.toByteArray()); Log.d("KeyHash:", Base64.encodeToString(md.digest(), Base64.DEFAULT)); } } catch (PackageManager.NameNotFoundException e) { } catch (NoSuchAlgorithmException e) { } }
Put this method in your onCreate() and run your project to get your key hashes in the logcat.
getKeyHash();
App DashBoard Settings
Go to dashboard of your app and select basic settings. Enter your Google Play Package Name, Class Name and Key Hashes that just generate.
Make your App Public
In your App Dashboard, click app review in the left navigate section and click the toggle to change your app to public.
Edit activity.main.xml layout
Go to activity.main.xml and add the facebook login button that provide by the facebook sdk. You can follow the source code below.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.questdot.facebookloginexample.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/textView" android:text="Hello World!" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <com.facebook.login.widget.LoginButton android:id="@+id/login_button" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_gravity="center_horizontal" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
Edit MainActivity.java class
Navigate to this class and copy the source code below and paste it in your class. It is to perform the login feature.
package com.example.questdot.facebookloginexample; import android.content.Context; import android.content.Intent; import android.content.pm.PackageInfo; import android.content.pm.PackageManager; import android.content.pm.Signature; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.AttributeSet; import android.util.Base64; import android.util.Log; import android.view.View; import android.widget.TextView; import com.facebook.CallbackManager; import com.facebook.FacebookCallback; import com.facebook.FacebookException; import com.facebook.FacebookSdk; import com.facebook.appevents.AppEventsLogger; import com.facebook.login.LoginResult; import com.facebook.login.widget.LoginButton; import java.security.MessageDigest; import java.security.NoSuchAlgorithmException; public class MainActivity extends AppCompatActivity implements FacebookCallback<LoginResult>{ private TextView textView; private LoginButton loginButton; private CallbackManager callbackManager; @Override protected void onActivityResult(int requestCode, int resultCode, Intent data) { callbackManager.onActivityResult(requestCode, resultCode, data); } @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); FacebookSdk.sdkInitialize(getApplicationContext()); callbackManager = CallbackManager.Factory.create(); setContentView(R.layout.activity_main); getKeyHash(); textView = (TextView)findViewById(R.id.textView); loginButton = (LoginButton)findViewById(R.id.login_button); textView.setText("hi"); loginButton.registerCallback(callbackManager,this); } public void getKeyHash(){ try { PackageInfo info = getPackageManager().getPackageInfo( getPackageName(), PackageManager.GET_SIGNATURES); for (Signature signature : info.signatures) { MessageDigest md = MessageDigest.getInstance("SHA"); md.update(signature.toByteArray()); Log.d("KeyHash:", Base64.encodeToString(md.digest(), Base64.DEFAULT)); } } catch (PackageManager.NameNotFoundException e) { } catch (NoSuchAlgorithmException e) { } } @Override public View onCreateView(String name, Context context, AttributeSet attrs) { return super.onCreateView(name, context, attrs); } @Override public void onSuccess(LoginResult loginResult) { textView.setText( "User ID: " + loginResult.getAccessToken().getUserId() + "\n" + "Auth Token: " + loginResult.getAccessToken().getToken() ); } @Override public void onCancel() { textView.setText("Login attempt canceled."); } @Override public void onError(FacebookException error) { textView.setText("Login attempt failed."); } }
Run your project
Now, you are able to use facebook login in your android project. Try it now in your android device.
Permissions
This link https://developers.facebook.com/docs/facebook-login/permissions is all the permissions that you able take by user. It able to take many kind of information from the user that given by facebook.
how to detect facebook account from this app? everytime i click login button, i must insert email and password (even i have login in official facebook app and android browser)
sorry for my english
i think u should add this in your Manifest file.
< activity
android:name=”com.facebook.FacebookActivity”
android:configChanges=”keyboard|keyboardHidden|screenLayout|screenSize|orientation”
android:theme=”@android:style/Theme.Translucent.NoTitleBar”
android:label=”@string/app_name”
/>