Android XML parsing Tutorial
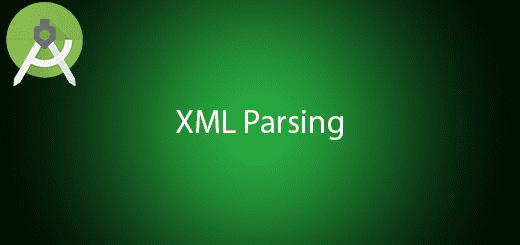
XML is extensible markup language which largely use for android layout and sharing data. XML is one of the popular format to sharing data on the internet while JSON are still not exist. For now is less likely to parsing XML to display content in the mobile application. Why JSON replace XML ? It is because JSON is more light weight , simple and better performance for developer while XML have more duplicate syntax and complex. In this tutorial, I will teach you android xml parsing in the mobile application.
Sample Screen
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “XMLParsingExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add new dependency
Open your build.gradle(Module:app) and add new dependency for parsing the xml.
dependencies { compile 'com.stanfy:gson-xml-java:0.1.+' }
Create two model class
Right click the package name of your application and add new class name “Recipetypes”. After that, follow the source code below.
import java.util.List; public class Recipetypes { private List<Recipetype> recipetype; public List<Recipetype> getRecipetype() { return recipetype; } public void setRecipetype(List<Recipetype> recipetype) { this.recipetype = recipetype; } }
Repeat to create a new model class again and this class will be name “Recipetype”. And also copy paste the below sample source code.
import com.google.gson.annotations.SerializedName; /** * Created by HP on 27/12/2016. */ public class Recipetype { @SerializedName("@id") private String id; private String name; public String getId() { return id; } public void setId(String id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } }
Create assets Folder
By creating an assets folder, right click app > new > folder > assets folder. Then you will see one assets folder in your app folder.
Create a new recipetypes.xml file
Right click assets folder and create a new xml file name “recipetypes”. After that, modify the source code to the below sample.
<?xml version="1.0" encoding="UTF-8"?> <recipetypes> <recipetype id="1"> <name>Vegetarian</name> </recipetype> <recipetype id="2"> <name>Fast Food</name> </recipetype> <recipetype id="3"> <name>Healthy</name> </recipetype> <recipetype id="4"> <name>No-Cook</name> </recipetype> <recipetype id="5"> <name>Make Ahead</name> </recipetype> </recipetypes>
Edit MainActivity.java class
Modify your mainactivity class and this class will be parse the xml file and display into your textview.
import android.content.res.AssetManager; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.ArrayAdapter; import android.widget.TextView; import com.stanfy.gsonxml.GsonXml; import com.stanfy.gsonxml.GsonXmlBuilder; import com.stanfy.gsonxml.XmlParserCreator; import org.xmlpull.v1.XmlPullParser; import org.xmlpull.v1.XmlPullParserFactory; import java.io.IOException; import java.io.InputStream; import java.util.ArrayList; public class MainActivity extends AppCompatActivity { TextView textView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); textView =(TextView)findViewById(R.id.textView); initItem(); } public void initItem(){ XmlParserCreator parserCreator = new XmlParserCreator() { @Override public XmlPullParser createParser() { try { return XmlPullParserFactory.newInstance().newPullParser(); } catch (Exception e) { throw new RuntimeException(e); } } }; GsonXml gsonXml = new GsonXmlBuilder() .setSameNameLists(true) .setXmlParserCreator(parserCreator) .create(); AssetManager assetManager = getAssets(); InputStream input; try { input = assetManager.open("recipetypes.xml"); int size = input.available(); byte[] buffer = new byte[size]; input.read(buffer); input.close(); String xml = new String(buffer); Recipetypes model = gsonXml.fromXml(xml, Recipetypes.class); String text=""; for(int num=0; num<model.getRecipetype().size();num++){ text+= model.getRecipetype().get(num).getName()+"\n"; } textView.setText(text); } catch (IOException e) { // TODO Auto-generated catch block e.printStackTrace(); } } }
Run Your Project
Lastly, you can run your application in your mobile device. You will see your textview text change because of xml parsing in the application.