BigchainDB CRAB (Create, Retrieve, Append and Burn) Tutorial
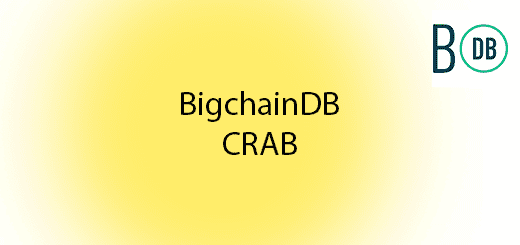
What is BigchainDB?
BigchainDB is a scalable blockchain database. It’s designed to merge the best of two worlds: the “traditional” distributed database world and the “traditional” blockchain world.
BigchainDB starts with a traditional distributed database (initially RethinkDB), which has characteristics of:
- scale (throughput, capacity, low latency), and
- query ability.
From that, we engineered in blockchain characteristics:
- decentralized (no single entity owns or controls it),
- immutable (tamper-resistance), and
- assets (you own the asset if you own the private key, aka blockchain-style permissioning).
BigchainDB supports both public and private deployments. Writes take less than a second because validation is based on a federation of voting nodes. BigchainDB’s querying isn’t in place yet, but will directly leverage the underlying database’s query functionality. From our experiments so far, BigchainDB’s architecture points towards 1 million write per second throughput and storing petabytes of data (via sharding).
Being a decentralized database, BigchainDB is complementary to decentralized processing technologies like Ethereum Virtual Machine, and decentralized file systems like IPFS. It can be used within decentralized computing platforms like BlockApps-Stratos or Eris-Tendermint.
Create a new folder called “bigchaindb_crab”.
mkdir bigchaindb_crab cd bigchaindb_crab
Set up a new npm package.
npm init -y
Install bigchaindb-orm using npm.
npm install --save bigchaindb-orm
Create the following directory structure and contents:
bigchaindb_crab |- package.json + |- connect.js + |- /crab + |- create.js + |- retrieve.js + |- append.js + |- burn.js
Edit the connect.js file.
// import bigchaindb-orm //import Orm from 'bigchaindb-orm' var Orm = require("bigchaindb-orm").default; // connect to BigchainDB const bdbOrm = new Orm("https://test.bigchaindb.com/api/v1/", { app_id: "-", app_key: "-" }); // define(<model name>,<additional information>) // <model name>: represents the name of model you want to store // <additional inf.>: any information you want to pass about the model (can be string or object) // note: cannot be changed once set! bdbOrm.define("myModel", "https://schema.org/v1/myModel"); // create a public and private key for Alice const aliceKeypair = new bdbOrm.driver.Ed25519Keypair(); module.exports = { bdbOrm, aliceKeypair };
Edit the crab/append.js file.
var connect = require("../connect.js"); // create an asset with Alice as owner connect.bdbOrm.models.myModel .create({ keypair: connect.aliceKeypair, data: { key: "dataValue" } }) .then(asset => { // lets append update the data of our asset // since we use a blockchain, we can only append return asset.append({ toPublicKey: connect.aliceKeypair.publicKey, keypair: connect.aliceKeypair, data: { key: "updatedValue" } }); }) .then(updatedAsset => { // updatedAsset contains the last (unspent) state // of our asset so any actions // need to be done to updatedAsset console.log(updatedAsset.data); });
Edit the crab/burn.js file.
var connect = require("../connect.js"); // create an asset with Alice as owner connect.bdbOrm.models.myModel .create({ keypair: connect.aliceKeypair, data: { key: "dataValue" } }) .then(asset => { // lets burn the asset by assigning to a random keypair // since will not store the private key it's infeasible to redeem the asset return asset.burn({ keypair: connect.aliceKeypair }); }) .then(burnedAsset => { // asset is now tagged as "burned" console.log(burnedAsset.data); });
Edit the crab/create.js file.
var connect = require("../connect.js"); // from the defined models in our bdbOrm we create an asset with Alice as owner connect.bdbOrm.models.myModel .create({ keypair: connect.aliceKeypair, data: { key: "dataValue" } }) .then(asset => { /* asset is an object with all our data and functions asset.id equals the id of the asset asset.data is data of the last (unspent) transaction asset.transactionHistory gives the full raw transaction history Note: Raw transaction history has different object structure then asset. You can find specific data change in metadata property. */ console.log(asset.id); });
Edit the crab/retrieve.js file.
var connect = require("../connect.js"); // get all objects with retrieve() // or get a specific object with retrieve(object.id) connect.bdbOrm.models.myModel .retrieve("4a2ca33e:20a1dfb1-d9b9-4fe0-a4aa-012f5d34380a") .then(assets => { // assets is an array of myModel console.log(assets.map(asset => asset.id)) })
Run node js file.
cd crab node create.js