Php Slim Framework Create REST api Tutorial
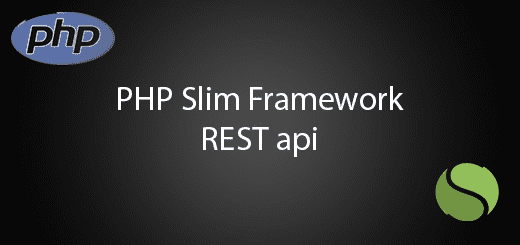
In previous tutorial, you already understand how to setup php slim framework in your computer and also learn how to change the domain name for your api. If you going to build a good mobile application or web application, you need the REST api to manage the database from the web server. It is a good practice for mobile app communicate with server and it is very simple to use and implement. In this tutorial, I will teach you basic method to use php slim framework create rest api for your mobile or web application.
HTTP Methods
There have 4 most used http methods that is very important when we are create the apis. Besides, there have other http methods which are Options, Head, Patch but it is less likely to use it. The good REST api should proper use the http methods. This tutorial I will teach you the following http methods which are GET,POST,PUT and DELETE.
GET | To fetch a resource |
POST | To create a new resource |
PUT | To update existing resource |
DELETE | To delete a resource |
HTTP Status Code
The Http Status Code will let the user know what error occur in the application. It is very important knowledge when create the REST api and use it. Sometime we will occur error, so we can figure out fast and fix it as soon as possible.
200 | OK |
201 | Created |
304 | Not Modified |
400 | Bad Request |
401 | Unauthorized |
403 | Forbidden |
404 | Not Found |
422 | Unprocessable Entity |
500 | Internal Server Error |
URL path
We need to use the proper url to specify the current feature so the user can refer to the url and know what the url will do for them. For an good example: GET http://abc.com/user/ – Will get all the user.
Simple REST API structure
The following table I list out url, method, parameters and the purpose of each api in this tutorial. This table will make you clearer on how to use it later.
URL | HTTP Method | Parameters | Purpose |
/user | GET | – | Get all user |
/user/{id} | GET | – | Get specific user by id |
/user/search/{query} | GET | query | Search all user by name |
/user | POST | name,email,phone | Insert a new user |
/user/{id} | PUT | name | Update specific user by id |
/user/{id} | DELETE | Delete specific user by id |
Create a new MySQL Database
Access your phpMyAdmin add a new database name “slim_db” after that insert the following SQL in query section. It will help you auto generate the table according the SQL.
CREATE TABLE `user` ( `id` int(11) NOT NULL, `name` varchar(50) NOT NULL, `email` varchar(50) NOT NULL, `phone` varchar(50) NOT NULL ) ENGINE=InnoDB DEFAULT CHARSET=latin1; INSERT INTO `user` (`id`, `name`, `email`, `phone`) VALUES (5, '11111', 'dsa', '321'), (6, '11111', 'dsa', '321'); ALTER TABLE `user` ADD PRIMARY KEY (`id`); ALTER TABLE `user` MODIFY `id` int(11) NOT NULL AUTO_INCREMENT;
File Structure
You can following my file structure in the following picture, or you can implement your own structure after this tutorial.
index.php – include all the API requests
.htaccess – Specify rules for url structure and apache
Create a config.php
This php file is to connect to the mysql database so you can do some change in your database.
<?php function getConnection() { $dbhost="localhost"; $dbuser="root"; $dbpass=""; $dbname="slim_db"; $dbh = new PDO("mysql:host=$dbhost;dbname=$dbname", $dbuser, $dbpass); $dbh->setAttribute(PDO::ATTR_ERRMODE, PDO::ERRMODE_EXCEPTION); return $dbh; }
Create a user.php
This php file to create the api and route each api to specific url so you can use it with different url.
/user (GET) – Get all user from your database and order by id. It will give you a json response so you can decode the json response and get data from your mobile app.
$app->get('/user', function ($request, $response, $args) { $sth = getConnection()->prepare("SELECT * FROM user ORDER BY id"); $sth->execute(); $todos = $sth->fetchAll(PDO::FETCH_ASSOC); return $response->withJson($todos); });
/user/{id} (GET) – Get specific user by id from your database, it only response for one user.
$app->get('/user/[{id}]', function ($request, $response, $args) { $sth = getConnection()->prepare("SELECT * FROM user WHERE id=:id"); $sth->bindParam("id", $args['id']); $sth->execute(); $todos = $sth->fetchObject(); return $response->withJson($todos); });
/user/search/{query} (GET) – Get the users according to the search query such as “abc”.
$app->get('/user/search/[{query}]', function ($request, $response, $args) { $sth = getConnection()->prepare("SELECT * FROM user WHERE name LIKE :query ORDER BY name"); $query = "%".$args['query']."%"; $sth->bindParam("query", $query); $sth->execute(); $todos = $sth->fetchAll(PDO::FETCH_ASSOC); return $response->withJson($todos); });
/user (POST) – Insert the new user in your database by using name, email and phone.
$app->post('/user', function ($request, $response) { $input = $request->getParsedBody(); $sql = "INSERT INTO `user` (`name`,`email`,`phone`) VALUES (:name,:email,:phone)"; $sth = getConnection()->prepare($sql); $sth->bindParam("name", $input['name']); $sth->bindParam("email", $input['email']); $sth->bindParam("phone", $input['phone']); $sth->execute(); $input['id'] = getConnection()->lastInsertId(); return $response->withJson($input); });
/user/{id} (DELETE) – Delete the user according to the id.
$app->delete('/user/[{id}]', function ($request, $response, $args) { $sth = getConnection()->prepare("DELETE FROM user WHERE id=:id"); $sth->bindParam("id", $args['id']); $sth->execute(); // $todos = $sth->fetchAll(PDO::FETCH_ASSOC); return 'Deleted'; });
/user/{id} (PUT) – Update the user according to the id.
$app->put('/user/[{id}]', function ($request, $response, $args) { $input = $request->getParsedBody(); $sql = "UPDATE user SET name=:name WHERE id=:id"; $sth = getConnection()->prepare($sql); $sth->bindParam("id", $args['id']); $sth->bindParam("name", $input['name']); $sth->execute(); $input['id'] = $args['id']; return $response->withJson($input); });
Edit index.php
<?php require 'C:\xampp\htdocs\slim-framework-rest-api\vendor\autoload.php'; $app = new Slim\App(); require_once('../include/config.php'); require_once('../api/user.php'); $app->run();
How to Test?
You can go download REST api client to test the api is working or not. I suggest you to use Postman which are the best REST api currently in the market.
For example to get user
(Php Slim Framework Create REST api)
Source Code
2