PHP Slim framework Eloquent ORM Tutorial
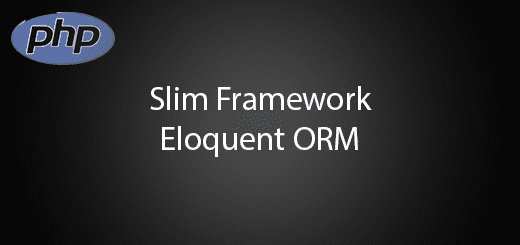
Beside using SQL query to retrieve data from database, you can also use a database Object Relational Mapper (ORM) Eloquent to connect your SlimPHP application. Object Relational Mapper means each of the database tables from the server is object. Therefore, you must write a new class for specific table and extends Eloquent. After that create a new instance of the class, now you can create,retrieve, update and delete the table record so don’t need to write any sql queries. The benefits of using ORM in your project is dont need to write complex sql queries and allow you to write simple syntax to control database. In this tutorial, I will tell you how to use PHP Slim framework Eloquent ORM in your project.
Create new project
Open PHPStorm and create a new project “eloquent-slim”. If you dont have PHPStorm inside your computer, click here to download.
Use composer to download library and framework
Open your command prompt and navigate to the “eloquent-slim” project that just create. After that enter the following command
composer require slim/slim "^3.0"
finsih then,
composer require illuminate/database "~5.1"
If you dont know how to make it, you can refer this https://questdot.com/setup-your-php-slim-framework-tutorial/.
File structure
This is the sample file structure, i create new folder for api,example, include and models.
Start your XAMPP
You need to run your web server and MySQL to proceed. If you dont know how to use XAMPP go to this link.
Create new mysql database
Go to the phpMyAdmin add a new database name “laravel” after that insert the following SQL in query section. It will help you auto generate the table according the SQL.
SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; SET time_zone = "+00:00"; CREATE TABLE `posts` ( `id` int(10) UNSIGNED NOT NULL, `text` text COLLATE utf8_unicode_ci NOT NULL, `created_at` timestamp NULL DEFAULT NULL, `updated_at` timestamp NULL DEFAULT NULL, `deleted_at` timestamp NULL DEFAULT NULL ) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci; INSERT INTO `posts` (`id`, `text`, `created_at`, `updated_at`, `deleted_at`) VALUES (1, 'hellosss', '2016-11-25 23:33:28', '2017-01-29 11:32:50', NULL); ALTER TABLE `posts` ADD PRIMARY KEY (`id`); ALTER TABLE `posts` MODIFY `id` int(10) UNSIGNED NOT NULL AUTO_INCREMENT, AUTO_INCREMENT=7;
Create new config.php file
Right click the project and create a new php file “config” inside the include folder , after that modify to the following source code. It is to connect to your mysql database. Make sure all field are correct.
<?php use Illuminate\Database\Capsule\Manager as Capsule; $app = new \Slim\App; $container = $app->getContainer(); $capsule = new Capsule; $capsule->addConnection([ 'driver' => 'mysql', 'host' => 'localhost', 'database' => 'laravel', 'username' => 'root', 'password' => '', 'charset' => 'utf8', 'collation' => 'utf8_unicode_ci', 'prefix' => '', ]); $capsule->bootEloquent(); $capsule->setAsGlobal();
Create new Post.php file
This is the model class inside the model folder, so you can use this class to control database.
<?php use Illuminate\Database\Eloquent\Model; class Post extends Model { protected $table = 'posts'; protected $fillable = [ 'id', 'text' ]; }
Create new post_api.php file
Add this new php file in your api folder, it route to 4 different feature which are create, delete, retrieve and update post for different url.
<?php use \Psr\Http\Message\ServerRequestInterface as Request; use \Psr\Http\Message\ResponseInterface as Response; $app->get('/post', function (Request $request, Response $response) { $posts = Post::all(); return $response->withJson($posts); }); $app->delete('/post/[{id}]', function ($request, $response, $args) { $id = $args['id']; $posts =Post::destroy($id); return $response->withJson($posts); }); $app->post('/post/[{id}]', function ($request, $response, $args) { $input = $request->getParsedBody(); $posts = Post::create(array( 'text' => $input['text'] )); return $response->withJson($posts); }); $app->put('/post/[{id}]', function ($request, $response, $args) { $input = $request->getParsedBody(); Post::where('id', $args['id'])->update(['text' => $input['text']]); return $response->withJson($input); });
Create a new index.php file
This php file is to include all the file that you just create and run it.
<?php require 'C:\Program Files (x86)\Ampps\www\eloquent-slim\vendor\autoload.php'; require '..\include\config.php'; include '..\models\Post.php'; include '..\api\post_api.php'; $app->run();
How to Test the API?
I suggest you to use Postman to test the rest api by using POST, GET,PUT and DELETE method. It is the best REST api client currently in the market. After test, you will know how the eloquent work in the project.
If you dont know how to use. You can refer to https://questdot.com/php-slim-framework-create-rest-api-tutorial/