Android Write and Read File Tutorial
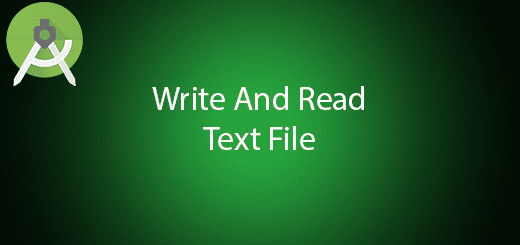
Android are able to let the android application to writing and reading text from a text file. There have two storage option that allow the application to use which are internal and external storage. The internal storage which is embeded in the mobile device while external storage can be remove it or change it such as SD card. There have three ways to write and read the file which are InputStream/OutputStream(byte stream), InputStreamReader/OutputStreamWritter(charater stream) and BufferReader/BufferedWriter(per line). The InputStream/OutputStream is the oldest method to read and write per bytes which not efficient and slow. I use BufferedWriter to writing the text file while BufferedReader to reading the text file. In this tutorial, I will give you some steps to make android write and read file in application.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “FileExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add Permissions
Go to AndroidManifest.xml add two permissions which are writing external storage and reading external storage. You can copy the source code below.
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE"/> <uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE"/>
Edit activity_main.xml layout
In your activity_main.xml add two button for read and write. After that add one editText and textView, you can following the sorce code below.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.example.questdot.fileexample.MainActivity"> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="WRITE" android:id="@+id/btnWrite" android:layout_below="@+id/editText" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <Button android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="READ" android:id="@+id/btnRead" android:layout_alignParentBottom="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" android:layout_alignRight="@+id/btnWrite" android:layout_alignEnd="@+id/btnWrite" /> <EditText android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/editText" android:layout_alignParentTop="true" android:layout_alignParentRight="true" android:layout_alignParentEnd="true" android:layout_alignParentLeft="true" android:layout_alignParentStart="true" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="-" android:id="@+id/textView" android:textSize="30dp" android:layout_centerVertical="true" android:layout_centerHorizontal="true" /> </RelativeLayout>
Edit MainActivity.java class
Copy and paste the following source code below, the two buttons perform the writing and reading the text file functionality.
package com.example.questdot.fileexample; import android.os.Environment; import android.os.Bundle; import android.support.v7.app.AppCompatActivity; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import java.io.BufferedReader; import java.io.BufferedWriter; import java.io.File; import java.io.FileReader; import java.io.FileWriter; import java.io.IOException; public class MainActivity extends AppCompatActivity implements View.OnClickListener{ private EditText editText; private TextView textView; private Button btnWrite,btnRead; String directory = Environment.getExternalStorageDirectory().getAbsolutePath() + "/newDir"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); btnWrite=(Button)findViewById(R.id.btnWrite); btnRead=(Button)findViewById(R.id.btnRead); editText=(EditText) findViewById(R.id.editText); textView=(TextView) findViewById(R.id.textView); btnRead.setOnClickListener(this); btnWrite.setOnClickListener(this); File dir = new File(directory); dir.mkdirs(); } @Override public void onClick(View view) { switch (view.getId()) { case R.id.btnWrite: File fileWrite = new File(directory+"/test1.txt"); String saveText = editText.getText().toString(); try { BufferedWriter bufferedWriter = new BufferedWriter(new FileWriter(fileWrite)); bufferedWriter.write(saveText); bufferedWriter.close(); Toast.makeText(getApplicationContext(), "Saved", Toast.LENGTH_LONG).show(); } catch (IOException e) { e.printStackTrace(); } break; case R.id.btnRead: File fileRead = new File(directory+"/test1.txt"); try { BufferedReader bufferedReader = new BufferedReader(new FileReader(fileRead)); String read; StringBuilder builder = new StringBuilder(""); while ((read = bufferedReader.readLine()) != null) { builder.append(read); } textView.setText(builder.toString()); bufferedReader.close(); } catch (IOException e) { e.printStackTrace(); } break; } } }
Run your project
Now, you can run the project on your android device and also you can check the text file in your directory after you writing the text file in the application.