How to configure Webpack and module bundling from scratch
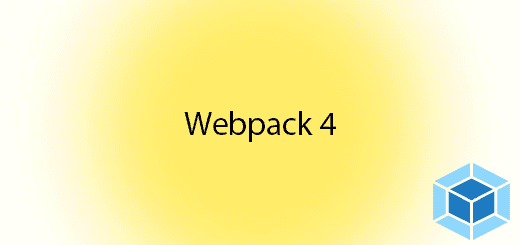
What is Webpack?
Webpack has become one of the most important tools for modern web development. It is a tool that lets you compile JavaScript modules, also known as module bundler. Given a large number of files (front-end assets) like HTML, CSS, even images, it generates a single file (or a few files) that run your app. It can give you more control over the number of HTTP requests your app is making and allows you to use other flavors of those assets such as Pug, Sass, and ES8. Webpack is not limited to be used on the frontend, it’s also useful in backend Node.js development as well.
Get started
Create a new folder “webpack-example”.
mkdir webpack-example cd webpack-example
Set up a new npm package.
npm init -y
Install webpack and webpack-cli using npm.
npm install --save-dev webpack webpack-cli
Create the following directory structure and contents:
webpack-example |- package.json + |- webpack.config.js + |- /src + |- index.js + |- /dist + |- index.html
Edit the dist/index.html file.
<!doctype html> <html> <head> <title>Hello Webpack</title> </head> <body> <script src="bundle.js"></script> </body> </html>Edit the src/index.js file.
const root = document.createElement("div") root.innerHTML = `<p>Hello Webpack.</p>` document.body.appendChild(root)Edit the webpack.config.js file.
const path = require('path') module.exports = { entry: './src/index.js', output: { filename: 'bundle.js', path: path.resolve(__dirname, 'dist') } }Edit the package.json file.
{ ... "scripts": { - "test": "echo \"Error: no test specified\" && exit 1" + "develop": "webpack --mode development --watch", + "build": "webpack --mode production" }, ... }
Run to build webpack production.
npm run build
Take another look at dist/bundle.js and you’ll see an ugly mess of code. Our bundle has been minified with UglifyJS.
The codes will run exactly the same, but it’s done with the smallest file size possible.
- –mode development optimizes for build speed and debugging.
- –mode production optimizes for execution speed at runtime and output file size.
Run your project
Open index.html file in your browser.