MongoDB PHP Perform Create Retrieve Update Delete
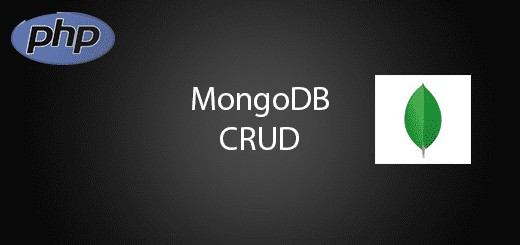
MongoDB is a document type NoSQL database and currently is the most popular NoSQL database use by many developers over the world. All of us curious that why MongoDB is better than MySQL in some area. MongoDB is non-relational database and based on BASE principle which are Basically, Available, Soft State and Eventually Consistent. It is good for handle large volumes of data compare to MySQL and its support unstructured and unpredictable data which dint need a lot of time to construct a database. The performance of MongoDB is quite fast compare to RBMS database like MySQL and MS SQL. In this tutorial, I will tell you how to connect mongodb php perform create retrieve update delete features in your application. This is just very simple.
First, you need to have setup mongodb server to operate in your local computer.
Connect to MongoDB server
Create a php file name “db_connect” and this file will be connect to mongodb server and select the database from your mongodb server. If the database dont exist in your server, it will be automatically create for you.
<?php // connect to mongodb local $m = new MongoClient(); // select a database $db = $m->testdb; ?>
Create a Collection
After that, create another file “create_collection” and copy paste the source code below in this file. It will be create the collection for your database.
<?php include('db_connect.php'); $collection = $db->createCollection("collection"); echo "Collection created succsessfully"; ?>
Insert a Document
Now, add a new php file “insert” and this file will be add the new document in the “collection”. The array filed are title, description and price.
<?php include('db_connect.php'); $collection = $db->collection; $document = array( "title" => "item", "description" => "abc", "price" => 100, "image_url" => "https://questdot.com/image/image.jpg" ); $collection->insert($document); echo "Document inserted successfully"; ?>
Retrieve all Documents
Create “find” php file and it will be retrieve all documents from the selected collection.
<?php include('db_connect.php'); $collection = $db->collection; $cursor = $collection->find(); // iterate cursor to display title of documents foreach ($cursor as $document) { echo $document["title"] . "\n"; } ?>
Update a Document
Create a file name “update” and implement the update document feature by the sample source code below.
<?php include('db_connect.php'); $collection = $db->collection; $collection->update(array("title"=>"item"), array('$set'=>array("title"=>"updated item"))); echo "Document updated successfully"; // now display the updated document $cursor = $collection->find(); // iterate cursor to display title of documents foreach ($cursor as $document) { echo $document["title"] . "\n"; } ?>
Delete a Document
Lastly for delete a document, create a php file “delete” and copy the source code below the perform delete.
<?php include('db_connect.php'); $collection = $db->collection; $collection->remove(array("title"=>"item")); echo "Documents deleted successfully"; // now display the available documents $cursor = $collection->find(); foreach ($cursor as $document) { echo $document["title"] . "\n"; } ?>
Run your Code
In Conclusion, you can now run your php source code in your web server, you will see the changes in your mongodb database. If you cannot view mongodb database from your server, you can download mongodb gui client (MongoBooster).
IS there a way to recover deleted collection?