Android Retrofit 2 HTTP Client communicate with REST API
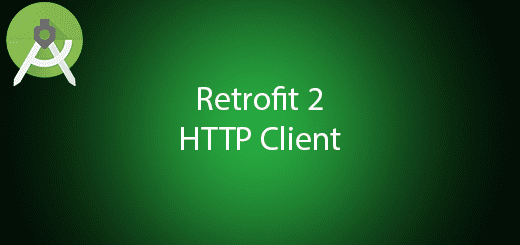
Android provide official way to make a http request by using Apache HttpClient or HttpUrlConnection bundle. The HttpClient is depreciated and removed in API 23 Android 6.0 and not success to use because it will be out dated soon. Besides, while new HttpUrlConnection have slow performance compare to http client library like retrofit, volley and okhttp. Therefore, i recommend the developer to use the latest retrofit 2 in your android application. It will provide you a better experience to the front user so your user will use your app. In this tutorial, I will teach you use the Android Retrofit 2 HTTP Client communicate with REST API in your application.
Creating a New Project
1. Open Android Studio IDE in your computer.
2. Create a new project and Edit the Application name to “RetrofitExample”.
(Optional) You can edit the company domain or select the suitable location for current project tutorial. Then click next button to proceed.
3. Select Minimum SDK (API 15:Android 4.0.3 (IceCreamSandwich). I choose the API 15 because many android devices currently are support more than API 15. Click Next button.
4. Choose “Empty Activity” and Click Next button
5. Lastly, press finish button.
Add 3 Dependencies
Add retrofit and gson dependencies in your dependencies sections so you can will those later.
compile 'com.google.code.gson:gson:2.6.2' compile 'com.squareup.retrofit2:retrofit:2.1.0' compile 'com.squareup.retrofit2:converter-gson:2.1.0'
Extra
I use mocky.io to create a sample rest api , this is the following url http://www.mocky.io/
Create a Class
Add a new class in your project name it to DoorResponse and this class in to get the response object from the rest api.
package com.questdot.retrofitexample; /** * Created by HP on 24/9/2016. */ import java.util.ArrayList; import java.util.List; public class DoorResponse { private Integer id; private String name; private Double price; private List<String> tags = new ArrayList<String>(); /** * No args constructor for use in serialization * */ public DoorResponse() { } /** * * @param tags * @param id * @param price * @param name */ public DoorResponse(Integer id, String name, Double price, List<String> tags) { this.id = id; this.name = name; this.price = price; this.tags = tags; } /** * * @return * The id */ public Integer getId() { return id; } /** * * @param id * The id */ public void setId(Integer id) { this.id = id; } /** * * @return * The name */ public String getName() { return name; } /** * * @param name * The name */ public void setName(String name) { this.name = name; } /** * * @return * The price */ public Double getPrice() { return price; } /** * * @param price * The price */ public void setPrice(Double price) { this.price = price; } /** * * @return * The tags */ public List<String> getTags() { return tags; } /** * * @param tags * The tags */ public void setTags(List<String> tags) { this.tags = tags; } }
Create an Interface
Add a new interface and name it to GetDoorAPI. It will specific the rest api url, you can see in the following source code.
package com.questdot.retrofitexample; import com.google.gson.Gson; import com.google.gson.GsonBuilder; import okhttp3.ResponseBody; import retrofit2.Call; import retrofit2.Retrofit; import retrofit2.converter.gson.GsonConverterFactory; import retrofit2.http.Field; import retrofit2.http.FormUrlEncoded; import retrofit2.http.GET; import retrofit2.http.POST; public interface GetDoorAPI { @POST("v2/57e55317110000e02481b1bf") Call<DoorResponse> postRawJson(); Gson gson = new GsonBuilder() .setDateFormat("yyyy-MM-dd'T'HH:mm:ssZ") .create(); Retrofit retrofit = new Retrofit.Builder() .baseUrl("http://www.mocky.io/") .addConverterFactory(GsonConverterFactory.create(gson)) .build(); GetDoorAPI getDoorAPI = retrofit.create(GetDoorAPI.class); }
Edit activity_main.xml
Open your activity_main.xml to edit the layout, it will be display the response from the retrofit in the activity layout.
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:id="@+id/activity_main" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context="com.questdot.retrofitexample.MainActivity"> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="ID" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:id="@+id/txtID" /> <TextView android:text="Name" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/txtID" android:layout_centerHorizontal="true" android:layout_marginTop="56dp" android:id="@+id/txtName" /> <TextView android:text="Price" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/txtName" android:layout_centerHorizontal="true" android:layout_marginTop="50dp" android:id="@+id/txtPrice" /> <TextView android:text="Tags" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_below="@+id/txtPrice" android:layout_centerHorizontal="true" android:layout_marginTop="109dp" android:id="@+id/txtTag" /> </RelativeLayout>
Edit MainActivity.java class
Go to the main class and edit it to the bottom source code, it will perform the asynchrous retrofit call in this class and set text to the activity.
package com.questdot.retrofitexample; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.widget.Button; import android.widget.EditText; import android.widget.TextView; import android.widget.Toast; import okhttp3.ResponseBody; import retrofit2.Call; import retrofit2.Callback; import retrofit2.Response; public class MainActivity extends AppCompatActivity { private TextView txtID; private TextView txtName; private TextView txtPrice; private TextView txtTag; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); txtID = (TextView) findViewById(R.id.txtID); txtName = (TextView) findViewById(R.id.txtName); txtPrice = (TextView) findViewById(R.id.txtPrice); txtTag = (TextView) findViewById(R.id.txtTag); getDoor(); } private void getDoor(){ Call<DoorResponse> call = GetDoorAPI.getDoorAPI.postRawJson(); //asynchronous call call.enqueue(new Callback<DoorResponse>() { @Override public void onResponse(Call<DoorResponse> call, Response<DoorResponse> response) { int code = response.code(); DoorResponse doorResponse = response.body(); if (code == 200) { txtID.setText(doorResponse.getId().toString()); txtName.setText(doorResponse.getName().toString()); txtPrice.setText(doorResponse.getPrice().toString()); txtTag.setText(doorResponse.getTags().get(0).toString()+ doorResponse.getTags().get(1).toString()); Toast.makeText(getApplicationContext(), "Work : " + response.message(), Toast.LENGTH_LONG).show(); } else { Toast.makeText(getApplicationContext(), "Did not work: "+code, Toast.LENGTH_LONG).show(); } } @Override public void onFailure(Call<DoorResponse> call, Throwable t) { } }); } }
Add permission
Go to your AndroidManifest.xml and add the new permission which is to access the internet in your project.
<uses-permission android:name="android.permission.INTERNET"/>
Run your project
Now, you can run the project and you will see the content will be change immediately.
1 Response
[…] previous tutorial, I had tell you how to use retrofit 2 to retrieve data from the rest api. Able to let user to […]